在Python列表中判断数值是否存在,可以使用多种方法,如in
运算符、列表的count()
方法、列表推导式和any()
函数等。通过这些方法,可以轻松检查一个数值是否存在于列表中。使用in
运算符、使用count()
方法、使用列表推导式和any()
函数、使用index()
方法。下面将详细介绍这些方法,并重点展开描述如何使用in
运算符。
使用in
运算符:
in
运算符是检查列表中是否存在某个数值的最简单和最直接的方法。它返回一个布尔值,表示数值是否存在于列表中。示例如下:
my_list = [1, 2, 3, 4, 5]
value = 3
if value in my_list:
print(f"{value} is in the list")
else:
print(f"{value} is not in the list")
在上面的示例中,in
运算符检查value
是否存在于my_list
中。如果存在,则输出"{value} is in the list"
,否则输出"{value} is not in the list"
。
一、使用in
运算符
使用in
运算符是最直观且高效的方法之一,尤其在需要快速判断数值是否存在时。Python的in
运算符在内部使用高效的查找算法,通常具有较好的性能表现。
my_list = [10, 20, 30, 40, 50]
value = 25
if value in my_list:
print("Value exists in the list")
else:
print("Value does not exist in the list")
在这个示例中,value
为25,不存在于my_list
中,因此输出为"Value does not exist in the list"
。
二、使用count()
方法
count()
方法可以统计列表中某个数值出现的次数。如果返回值大于0,则表示数值存在于列表中。
my_list = [7, 8, 9, 10, 7]
value = 7
if my_list.count(value) > 0:
print("Value exists in the list")
else:
print("Value does not exist in the list")
在这个示例中,count()
方法返回2
,表示数值7
在列表中出现了两次,因此输出为"Value exists in the list"
。
三、使用列表推导式和any()
函数
列表推导式和any()
函数可以结合使用来检查数值是否存在于列表中。any()
函数返回True
,如果列表推导式生成的任意元素为True
。
my_list = [15, 25, 35, 45, 55]
value = 35
exists = any([True for x in my_list if x == value])
if exists:
print("Value exists in the list")
else:
print("Value does not exist in the list")
在这个示例中,列表推导式生成一个布尔列表,any()
函数检查列表中是否存在True
,如果存在,则表示数值存在于列表中。
四、使用index()
方法
index()
方法返回数值在列表中的第一个匹配项的索引。如果数值不存在于列表中,会引发ValueError
异常。
my_list = [100, 200, 300, 400, 500]
value = 300
try:
index = my_list.index(value)
print(f"Value exists in the list at index {index}")
except ValueError:
print("Value does not exist in the list")
在这个示例中,index()
方法返回2
,表示数值300
在列表中的索引为2
,因此输出为"Value exists in the list at index 2"
。如果数值不存在,程序会捕获ValueError
异常,并输出"Value does not exist in the list"
。
五、使用集合(Set)进行判断
如果需要对大量数据进行多次查找,可以考虑将列表转换为集合。集合的查找操作时间复杂度为O(1),因此在处理大数据量时具有更好的性能。
my_list = [5, 10, 15, 20, 25, 30]
value = 20
my_set = set(my_list)
if value in my_set:
print("Value exists in the list")
else:
print("Value does not exist in the list")
在这个示例中,将列表转换为集合后,使用in
运算符进行查找操作,这样可以更高效地判断数值是否存在于列表中。
六、使用filter()
函数
filter()
函数可以用于筛选出符合条件的元素,结合list()
函数可以将筛选结果转为列表。如果结果列表不为空,则表示数值存在。
my_list = [2, 4, 6, 8, 10]
value = 6
filtered_list = list(filter(lambda x: x == value, my_list))
if filtered_list:
print("Value exists in the list")
else:
print("Value does not exist in the list")
在这个示例中,filter()
函数筛选出符合条件的元素,并转为列表。如果结果列表不为空,则表示数值存在。
七、使用bisect
模块进行二分查找
对于已排序的列表,可以使用bisect
模块进行二分查找,这样可以更快速地判断数值是否存在。
import bisect
my_list = [3, 6, 9, 12, 15]
value = 9
index = bisect.bisect_left(my_list, value)
if index < len(my_list) and my_list[index] == value:
print("Value exists in the list")
else:
print("Value does not exist in the list")
在这个示例中,使用bisect.bisect_left()
方法进行二分查找,判断数值是否存在于已排序的列表中。
八、使用numpy库进行查找
如果处理的是数值列表,可以使用numpy
库进行查找操作,numpy
具有高效的数组操作性能。
import numpy as np
my_list = [11, 22, 33, 44, 55]
value = 33
np_array = np.array(my_list)
if value in np_array:
print("Value exists in the list")
else:
print("Value does not exist in the list")
在这个示例中,使用numpy
库将列表转换为数组,并使用in
运算符进行查找操作。
九、使用pandas库进行查找
如果处理的是数据框,可以使用pandas
库进行查找操作,pandas
具有强大的数据处理能力。
import pandas as pd
my_list = [21, 42, 63, 84, 105]
value = 63
df = pd.DataFrame(my_list, columns=['Values'])
if value in df['Values'].values:
print("Value exists in the list")
else:
print("Value does not exist in the list")
在这个示例中,使用pandas
库将列表转换为数据框,并使用in
运算符进行查找操作。
十、总结
在Python列表中判断数值是否存在,可以使用多种方法,包括in
运算符、count()
方法、列表推导式和any()
函数、index()
方法、集合、filter()
函数、bisect
模块、numpy
库和pandas
库等。选择合适的方法取决于具体的应用场景和数据量。对于大多数情况,in
运算符是最简单和高效的方法,而对于大数据量和特定需求,可以选择其他更合适的方法,如集合、numpy
库或pandas
库等。
相关问答FAQs:
如何在Python列表中高效查找特定数值?
在Python中,可以使用in
关键字来检查一个数值是否存在于列表中。这个方法简单而直观。例如,if value in my_list:
会返回True或False,指示数值是否在列表中。此外,使用list.count(value)
方法可以统计数值在列表中出现的次数,从而进一步了解其存在情况。
列表查找时有哪些常用的方法?
除了使用in
关键字,Python还提供了一些其他方法来判断数值是否存在。index()
方法可以返回数值在列表中的索引,如果数值不存在则会引发ValueError
。还有使用filter()
函数,结合lambda
表达式,可以筛选出满足条件的数值,帮助进行更复杂的查找。
如何处理列表中不存在的数值的情况?
在检查数值是否存在于列表时,考虑到可能不存在的情况,可以使用异常处理来避免程序崩溃。使用try...except
语句来捕获ValueError
,可以优雅地处理查找失败的情形。例如,try: index = my_list.index(value) except ValueError: index = -1
,这样可以返回-1表示未找到,而不会导致程序中断。
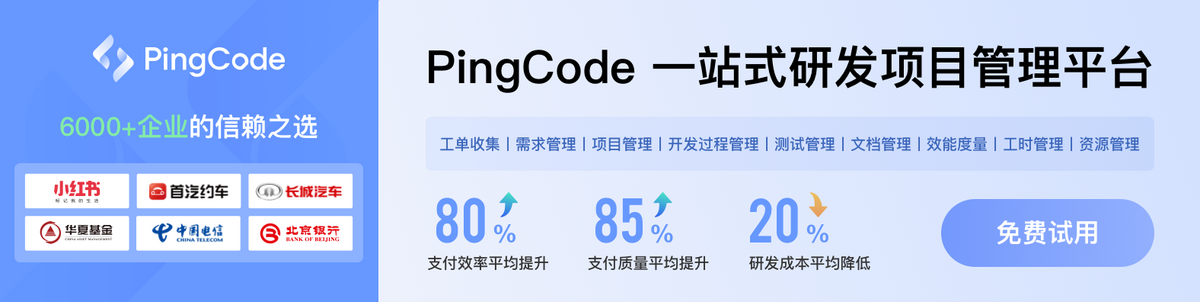