Python循环一次知乎的核心观点有:使用requests库发送请求、使用BeautifulSoup解析HTML、模拟浏览器请求、处理反爬虫机制、分页抓取内容。下面将详细描述其中的一个核心观点:使用requests库发送请求。
在进行知乎的内容抓取时,首先需要发送HTTP请求获取网页内容。requests库是一个非常流行的Python库,专门用于发送HTTP请求。使用requests库可以方便地发送GET或POST请求,并获取响应内容。比如,使用requests库获取知乎某个问题页面的HTML内容,代码如下:
import requests
url = 'https://www.zhihu.com/question/123456789' # 替换为实际问题的URL
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
html_content = response.text
以上代码中,我们设置了User-Agent头部,模拟浏览器请求,以避免被知乎的反爬虫机制检测到。同时,将获取到的HTML内容存储在html_content变量中,方便后续解析。
一、使用requests库发送请求
使用requests库发送HTTP请求是获取网页内容的第一步。requests库不仅支持GET和POST请求,还支持其他HTTP方法,如PUT、DELETE等。通过设置请求头部,我们可以模拟浏览器的请求,绕过一些简单的反爬虫机制。
import requests
def fetch_page_content(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
return response.text
url = 'https://www.zhihu.com/question/123456789' # 替换为实际问题的URL
html_content = fetch_page_content(url)
在上面的代码中,我们定义了一个fetch_page_content函数,该函数接受URL作为参数,并返回网页的HTML内容。通过设置请求头部,我们可以模拟浏览器发送请求。
二、使用BeautifulSoup解析HTML
获取到网页的HTML内容后,我们需要使用BeautifulSoup库解析HTML,提取我们需要的信息。BeautifulSoup是一个功能强大的Python库,专门用于解析HTML和XML文档。
from bs4 import BeautifulSoup
def parse_html_content(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
question_title = soup.find('h1', class_='QuestionHeader-title').get_text()
return question_title
html_content = fetch_page_content(url)
question_title = parse_html_content(html_content)
print(question_title)
在上面的代码中,我们定义了一个parse_html_content函数,该函数接受HTML内容作为参数,并返回问题的标题。我们使用BeautifulSoup解析HTML,并查找具有特定类名的h1标签,提取其文本内容。
三、模拟浏览器请求
为了绕过知乎的反爬虫机制,我们可以使用requests库模拟浏览器请求。除了设置User-Agent头部外,我们还可以添加其他头部信息,如Referer、Cookies等。
import requests
def fetch_page_content(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://www.zhihu.com/',
'Cookie': 'your_cookie_here' # 替换为实际的Cookie
}
response = requests.get(url, headers=headers)
return response.text
url = 'https://www.zhihu.com/question/123456789' # 替换为实际问题的URL
html_content = fetch_page_content(url)
在上面的代码中,我们添加了Referer和Cookie头部信息,以进一步模拟浏览器请求。这可以帮助我们绕过一些更复杂的反爬虫机制。
四、处理反爬虫机制
知乎等网站通常会使用反爬虫机制来防止自动化抓取。为了应对这些机制,我们需要使用一些技术手段,如设置请求头部、使用代理服务器、添加延时等。
import requests
import time
def fetch_page_content(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://www.zhihu.com/',
'Cookie': 'your_cookie_here' # 替换为实际的Cookie
}
proxies = {
'http': 'http://your_proxy_here', # 替换为实际的代理服务器
'https': 'https://your_proxy_here'
}
response = requests.get(url, headers=headers, proxies=proxies)
time.sleep(1) # 添加延时,避免过于频繁的请求
return response.text
url = 'https://www.zhihu.com/question/123456789' # 替换为实际问题的URL
html_content = fetch_page_content(url)
在上面的代码中,我们使用了代理服务器,并添加了延时,以避免过于频繁的请求。这些措施可以帮助我们绕过一些反爬虫机制。
五、分页抓取内容
知乎的问题页面可能包含多个分页,为了抓取所有内容,我们需要处理分页逻辑。通常,可以通过分析网页的分页结构,找到下一页的URL,并递归抓取所有分页内容。
import requests
from bs4 import BeautifulSoup
def fetch_page_content(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://www.zhihu.com/',
'Cookie': 'your_cookie_here' # 替换为实际的Cookie
}
response = requests.get(url, headers=headers)
return response.text
def parse_html_content(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
question_title = soup.find('h1', class_='QuestionHeader-title').get_text()
answers = []
for answer in soup.find_all('div', class_='List-item'):
answer_content = answer.find('div', class_='RichContent-inner').get_text()
answers.append(answer_content)
next_page = soup.find('button', class_='Button PaginationButton PaginationButton-next Button--plain')
next_page_url = next_page['href'] if next_page else None
return question_title, answers, next_page_url
def fetch_all_pages(url):
all_answers = []
while url:
html_content = fetch_page_content(url)
question_title, answers, next_page_url = parse_html_content(html_content)
all_answers.extend(answers)
url = next_page_url
return question_title, all_answers
url = 'https://www.zhihu.com/question/123456789' # 替换为实际问题的URL
question_title, all_answers = fetch_all_pages(url)
print(question_title)
print(all_answers)
在上面的代码中,我们定义了fetch_all_pages函数,该函数递归抓取所有分页内容。通过解析下一页的URL,我们可以依次获取所有分页内容,直至没有下一页为止。
六、处理JavaScript动态加载内容
有些网页内容是通过JavaScript动态加载的,requests库无法直接获取这些内容。此时,我们可以使用Selenium等浏览器自动化工具模拟用户操作,加载动态内容,并获取完整的网页内容。
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
def fetch_page_content_with_selenium(url):
options = Options()
options.add_argument('--headless')
options.add_argument('--disable-gpu')
service = Service(executable_path='/path/to/chromedriver') # 替换为实际的chromedriver路径
driver = webdriver.Chrome(service=service, options=options)
driver.get(url)
html_content = driver.page_source
driver.quit()
return html_content
url = 'https://www.zhihu.com/question/123456789' # 替换为实际问题的URL
html_content = fetch_page_content_with_selenium(url)
在上面的代码中,我们使用Selenium模拟浏览器操作,加载动态内容,并获取完整的网页HTML内容。通过设置无头模式和禁用GPU,我们可以在后台运行Selenium,避免占用过多资源。
七、数据存储与处理
抓取到的知乎内容可以存储在文件、数据库等不同的存储介质中。为了方便后续数据分析和处理,我们可以将抓取到的内容存储在结构化的数据库中,如MySQL、MongoDB等。
import pymysql
def store_data_in_mysql(question_title, answers):
connection = pymysql.connect(host='localhost',
user='root',
password='your_password',
database='zhihu')
cursor = connection.cursor()
cursor.execute("INSERT INTO questions (title) VALUES (%s)", (question_title,))
question_id = cursor.lastrowid
for answer in answers:
cursor.execute("INSERT INTO answers (question_id, content) VALUES (%s, %s)", (question_id, answer))
connection.commit()
cursor.close()
connection.close()
question_title, all_answers = fetch_all_pages(url)
store_data_in_mysql(question_title, all_answers)
在上面的代码中,我们使用pymysql库将抓取到的知乎内容存储在MySQL数据库中。首先,我们将问题标题插入questions表,并获取其ID。然后,将每个答案插入answers表,并关联到对应的问题ID。
八、处理异常和错误
在进行网络请求和数据抓取时,可能会遇到各种异常和错误,如网络超时、请求失败等。为了保证程序的稳定性,我们需要处理这些异常,并采取适当的重试机制。
import requests
from requests.exceptions import RequestException
import time
def fetch_page_content(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://www.zhihu.com/',
'Cookie': 'your_cookie_here' # 替换为实际的Cookie
}
for _ in range(3): # 重试3次
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
return response.text
except RequestException as e:
print(f"Error fetching {url}: {e}")
time.sleep(2) # 等待2秒后重试
return None
url = 'https://www.zhihu.com/question/123456789' # 替换为实际问题的URL
html_content = fetch_page_content(url)
在上面的代码中,我们在fetch_page_content函数中添加了异常处理和重试机制。当请求失败时,程序会等待2秒后重试,最多重试3次。通过这种方式,可以提高程序的稳定性,避免因临时网络问题导致抓取失败。
九、优化抓取性能
在进行大规模数据抓取时,性能是一个重要的考虑因素。为了提高抓取性能,我们可以使用多线程或异步编程技术,并优化请求和解析流程。
import requests
from concurrent.futures import ThreadPoolExecutor
from bs4 import BeautifulSoup
def fetch_page_content(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://www.zhihu.com/',
'Cookie': 'your_cookie_here' # 替换为实际的Cookie
}
response = requests.get(url, headers=headers)
return response.text
def parse_html_content(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
question_title = soup.find('h1', class_='QuestionHeader-title').get_text()
answers = []
for answer in soup.find_all('div', class_='List-item'):
answer_content = answer.find('div', class_='RichContent-inner').get_text()
answers.append(answer_content)
return question_title, answers
def fetch_and_parse(url):
html_content = fetch_page_content(url)
return parse_html_content(html_content)
urls = [
'https://www.zhihu.com/question/123456789', # 替换为实际问题的URL
'https://www.zhihu.com/question/987654321',
# 添加更多URL
]
with ThreadPoolExecutor(max_workers=5) as executor:
results = list(executor.map(fetch_and_parse, urls))
for question_title, answers in results:
print(question_title)
print(answers)
在上面的代码中,我们使用ThreadPoolExecutor实现多线程抓取。通过设置最大线程数,我们可以同时发送多个请求,提高抓取效率。将fetch_page_content和parse_html_content函数组合为fetch_and_parse函数,并使用executor.map并行处理多个URL。
十、总结
本文详细介绍了如何使用Python循环抓取知乎内容的步骤和技巧。从使用requests库发送请求、使用BeautifulSoup解析HTML,到模拟浏览器请求、处理反爬虫机制,再到分页抓取内容、处理JavaScript动态加载内容,最后到数据存储与处理、处理异常和错误、优化抓取性能,每一步都进行了详细的讲解。通过掌握这些技术和方法,您可以高效地抓取知乎等网站的内容,并进行数据分析和处理。希望本文对您有所帮助。
相关问答FAQs:
如何使用Python进行知乎内容抓取?
使用Python抓取知乎内容通常可以借助一些库,如requests和BeautifulSoup。requests库用于发送HTTP请求获取页面内容,而BeautifulSoup则用于解析HTML文档。通过这两者的结合,可以提取出所需的信息,比如文章标题、作者、评论等。
Python中有哪些库可以帮助实现循环和抓取知乎数据?
在Python中,除了requests和BeautifulSoup外,Scrapy也是一个强大的框架,专门用于网络抓取。使用Scrapy可以更高效地处理请求和解析数据。对于处理循环操作,Python的for循环和while循环都能够实现重复抓取特定页面或内容的需求。
在抓取知乎内容时需要注意哪些法律和道德问题?
抓取知乎内容需要遵守相关法律法规,确保不侵犯知识产权。避免过于频繁地访问同一页面,以免对知乎服务器造成负担。此外,建议在抓取数据之前,查看并遵守知乎的使用条款,确保抓取行为不违反平台政策。
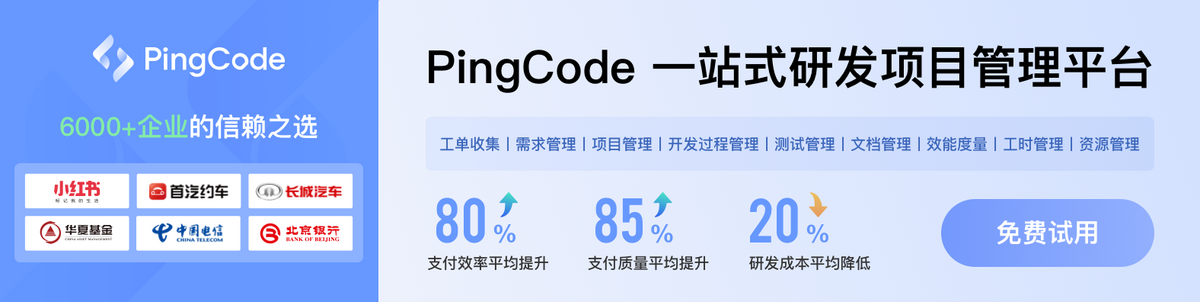