Python 3 如何安装 urllib2?
Python 3 中不需要安装 urllib2、Python 3 已经将 urllib2 拆分为多个模块、使用 urllib.request 和 urllib.error 替代 urllib2。
在 Python 3 中,不再有 urllib2 模块,这个模块已经被拆分为几个不同的模块,包括 urllib.request
和 urllib.error
。因此,在 Python 3 中要使用 urllib2 的功能,您只需导入这些新的模块即可,而不需要进行额外的安装。以下是详细的解释和使用方法。
一、urllib2 在 Python 3 中的替代方案
1. urllib.request
urllib.request
是一个用于打开和读取 URLs(主要是 HTTP URLs)的模块。它提供了一些简单的接口来处理常见的 HTTP 请求。
import urllib.request
url = 'http://www.example.com'
response = urllib.request.urlopen(url)
html = response.read()
print(html)
在这个例子中,我们使用 urllib.request.urlopen
打开一个 URL 并读取其内容。这个函数返回一个类文件对象,因此我们可以像读取文件一样读取数据。
2. urllib.error
urllib.error
包含了用于处理异常的模块。它定义了异常类,用于在处理 URL 时捕获错误。
import urllib.request
import urllib.error
url = 'http://www.example.com'
try:
response = urllib.request.urlopen(url)
html = response.read()
print(html)
except urllib.error.URLError as e:
print(f'Failed to reach the server: {e.reason}')
except urllib.error.HTTPError as e:
print(f'Server could not fulfill the request: {e.code}')
在这个例子中,我们使用 try
和 except
块来捕获 URL 请求过程中可能发生的异常。urllib.error.URLError
用于捕获网络连接错误,而 urllib.error.HTTPError
用于捕获 HTTP 协议错误。
二、如何在 Python 3 中使用 urllib 模块
1. 发送 GET 请求
GET 请求是最常见的 HTTP 请求方法。它用于从服务器获取数据。
import urllib.request
url = 'http://www.example.com'
response = urllib.request.urlopen(url)
html = response.read().decode('utf-8')
print(html)
在这个例子中,我们发送一个 GET 请求并读取响应。decode('utf-8')
用于将字节数据转换为字符串。
2. 发送 POST 请求
POST 请求用于向服务器发送数据。
import urllib.request
import urllib.parse
url = 'http://www.example.com'
data = urllib.parse.urlencode({'key': 'value'}).encode('utf-8')
response = urllib.request.urlopen(url, data=data)
html = response.read().decode('utf-8')
print(html)
在这个例子中,我们首先使用 urllib.parse.urlencode
将数据编码为 application/x-www-form-urlencoded
格式,然后将其转换为字节数据并发送 POST 请求。
3. 添加请求头
有时,我们需要在请求中添加头信息,例如用户代理(User-Agent)。
import urllib.request
url = 'http://www.example.com'
headers = {'User-Agent': 'Mozilla/5.0'}
request = urllib.request.Request(url, headers=headers)
response = urllib.request.urlopen(request)
html = response.read().decode('utf-8')
print(html)
在这个例子中,我们创建了一个 Request
对象,并在其中添加了头信息,然后发送请求。
三、处理 JSON 数据
在现代 web 开发中,JSON 是一种非常常见的数据格式。我们可以使用 json
模块来解析和处理 JSON 数据。
import urllib.request
import json
url = 'http://api.example.com/data'
response = urllib.request.urlopen(url)
data = json.loads(response.read().decode('utf-8'))
print(data)
在这个例子中,我们从一个 API 获取 JSON 数据,并使用 json.loads
将其解析为 Python 字典。
四、处理 URL 编码和解码
在处理 URL 时,我们经常需要进行编码和解码操作。urllib.parse
模块提供了相关的函数。
1. URL 编码
import urllib.parse
params = {'key1': 'value1', 'key2': 'value2'}
encoded_params = urllib.parse.urlencode(params)
print(encoded_params)
在这个例子中,我们使用 urllib.parse.urlencode
将参数字典编码为查询字符串格式。
2. URL 解码
import urllib.parse
encoded_params = 'key1=value1&key2=value2'
decoded_params = urllib.parse.parse_qs(encoded_params)
print(decoded_params)
在这个例子中,我们使用 urllib.parse.parse_qs
将查询字符串解码为字典。
五、使用 urllib 处理文件下载
urllib
模块还可以用于下载文件。
import urllib.request
url = 'http://www.example.com/somefile.zip'
filename = 'somefile.zip'
urllib.request.urlretrieve(url, filename)
print(f'File downloaded: {filename}')
在这个例子中,我们使用 urllib.request.urlretrieve
从 URL 下载文件并保存到本地。
六、使用代理
有时我们需要通过代理服务器访问互联网。我们可以使用 urllib
模块来配置代理。
import urllib.request
proxy = urllib.request.ProxyHandler({'http': 'http://proxy.example.com:8080'})
opener = urllib.request.build_opener(proxy)
urllib.request.install_opener(opener)
url = 'http://www.example.com'
response = urllib.request.urlopen(url)
html = response.read().decode('utf-8')
print(html)
在这个例子中,我们创建了一个代理处理器,并将其安装为全局的 URL 打开器。
七、总结
在 Python 3 中,urllib2
模块已经被拆分为多个模块,包括 urllib.request
和 urllib.error
。通过这些模块,我们可以进行 HTTP 请求、处理异常、解析和编码 URL、处理 JSON 数据、下载文件以及使用代理。这些模块提供了丰富的功能,可以满足大多数网络编程需求。
相关问答FAQs:
1. 在Python 3中,我应该使用什么库来替代urllib2?
在Python 3中,urllib2已被拆分并重构为多个模块。您可以使用urllib.request
来处理与网络请求相关的功能。具体来说,urllib.request
提供了与urllib2相似的功能,如发送HTTP请求和处理响应。
2. 如何在Python 3中使用urllib.request发送GET和POST请求?
要发送GET请求,您可以使用urllib.request.urlopen()
方法。例如:
import urllib.request
response = urllib.request.urlopen('http://example.com')
html = response.read()
对于POST请求,您可以使用urllib.request.Request
类并指定方法为POST:
import urllib.request
import urllib.parse
data = urllib.parse.urlencode({'key': 'value'}).encode()
req = urllib.request.Request('http://example.com', data=data)
response = urllib.request.urlopen(req)
html = response.read()
3. 如果我想在Python 3中处理HTTP请求的异常,应该如何做?
在Python 3中,可以使用try-except块来捕获和处理异常。例如:
import urllib.request
try:
response = urllib.request.urlopen('http://example.com')
html = response.read()
except urllib.error.URLError as e:
print(f'URL error: {e.reason}')
except urllib.error.HTTPError as e:
print(f'HTTP error: {e.code} - {e.reason}')
这种方式可以帮助您捕获网络请求中可能出现的错误,并进行相应的处理。
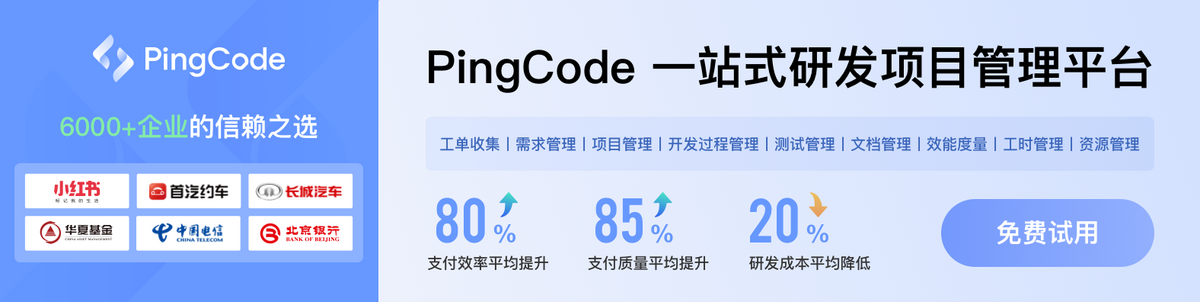