在Python中输出两位小数的方法包括:使用字符串格式化、使用 format()
方法、使用 f-strings
、以及 round()
函数。这些方法各有优点,例如:字符串格式化操作灵活、f-strings
语法简洁等。接下来,我们将详细介绍这些方法及其应用。
一、使用字符串格式化
字符串格式化是一种经典的方法,在输出控制方面非常灵活。通过 %.2f
可以指定输出两位小数。
value = 3.14159
formatted_value = "%.2f" % value
print(formatted_value)
这种方法使用百分号 %
作为格式化操作符,%.2f
表示小数点后保留两位。
二、使用 format()
方法
format()
方法是 Python 2.7 和 3.1 引入的一个新的格式化字符串的方法,可以更灵活地处理字符串格式化。
value = 3.14159
formatted_value = "{:.2f}".format(value)
print(formatted_value)
{:.2f}
是格式化说明符,其中 :
引导格式说明符, .2
表示小数点后保留两位,f
表示浮点数。
三、使用 f-strings
(Python 3.6+)
f-strings
是 Python 3.6 引入的新特性,可以在字符串前加上 f
或 F
,并在字符串内使用 {}
包含表达式。
value = 3.14159
formatted_value = f"{value:.2f}"
print(formatted_value)
f-strings
语法简洁,且性能优于 format()
方法,适合在大量格式化操作时使用。
四、使用 round()
函数
round()
函数可以对浮点数进行四舍五入,返回指定精度的小数。
value = 3.14159
rounded_value = round(value, 2)
print(rounded_value)
需要注意的是,round()
函数返回的是浮点数,如果需要输出为字符串格式,仍需进行字符串转换。
深入解析 format()
方法
format()
方法不仅可以指定小数位数,还可以进行更多复杂的格式化操作。以下是一些常见的使用方式:
value = 3.14159
保留两位小数
formatted_value = "{:.2f}".format(value)
以科学计数法表示
formatted_sci = "{:.2e}".format(value)
添加千位分隔符
large_value = 1234567.8901
formatted_large = "{:,.2f}".format(large_value)
print(formatted_value)
print(formatted_sci)
print(formatted_large)
{:.2e}
表示以科学计数法表示, {:,.2f}
表示带千位分隔符并保留两位小数。
综合应用实例
以下示例展示了如何在实际应用中使用这些方法:
def format_prices(prices):
formatted_prices = []
for price in prices:
formatted_price = f"${price:.2f}"
formatted_prices.append(formatted_price)
return formatted_prices
示例数据
prices = [3.14159, 2.71828, 1.41421, 0.57721]
formatted_prices = format_prices(prices)
for price in formatted_prices:
print(price)
在这个例子中,我们定义了一个 format_prices
函数,使用 f-strings
对价格列表中的每个价格进行格式化,输出结果为保留两位小数的美元价格。
进阶技巧:结合正则表达式进行格式化
在某些情况下,可能需要对复杂的文本进行格式化,这时可以结合正则表达式进行处理:
import re
def format_numbers_in_text(text):
pattern = r'\d+\.\d+'
def format_match(match):
return f"{float(match.group()):.2f}"
formatted_text = re.sub(pattern, format_match, text)
return formatted_text
示例文本
text = "The values are 3.14159, 2.71828, and 1.41421."
formatted_text = format_numbers_in_text(text)
print(formatted_text)
在这个例子中,使用正则表达式匹配浮点数,并对匹配到的浮点数进行格式化,输出保留两位小数的结果。
通过上述方法,Python 提供了多种灵活的方式来输出两位小数,可以根据具体需求选择合适的方法。在实际开发中,掌握这些技巧可以显著提高代码的可读性和执行效率。
相关问答FAQs:
如何在Python中格式化输出为两位小数?
在Python中,可以使用内置的format()
函数或f-string来实现两位小数的格式化输出。使用format()
时,您可以这样写:"{:.2f}".format(数字)
,而使用f-string的方式则为f"{数字:.2f}"
。这两种方法都会将数字格式化为两位小数。
如果我想在输出中包含千位分隔符,该如何做?
要在输出中加入千位分隔符并保持两位小数,可以使用format()
函数或f-string中的格式说明符。例如,使用"{:,.2f}".format(数字)
或f"{数字:,.2f}"
,这样输出的数字将包含千位分隔符,并保留两位小数。
在Python中,如何处理小数点后多于两位的数字?
当您处理的小数点后位数超过两位时,可以使用round()
函数先进行四舍五入,然后再格式化输出。例如,数字 = round(数字, 2)
可以将数字四舍五入到两位小数,接着使用前面提到的格式化方法输出结果。这样可以确保输出的数字始终符合两位小数的要求。
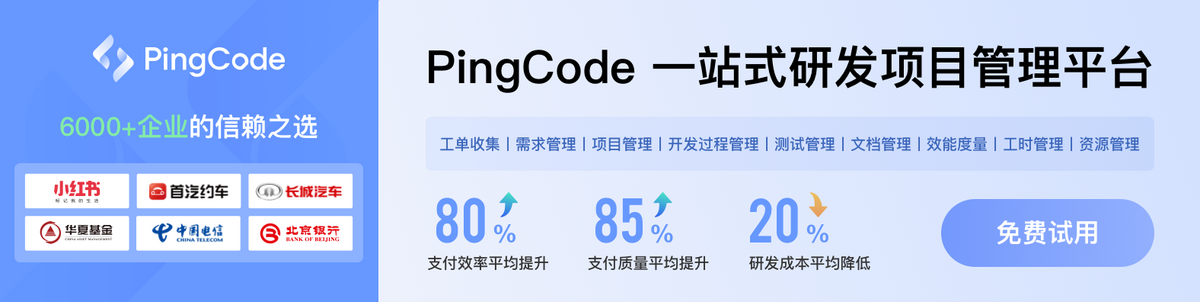