在Python中可以通过多种方式判断多个字符:使用 'in' 运算符、使用字符串方法、使用正则表达式。 其中,使用正则表达式是一种非常强大且灵活的方法。
在Python中处理字符串时,经常需要判断字符串是否包含特定的字符或子字符串。以下将详细介绍几种常见的方法,并以正则表达式为重点,展示如何在Python中实现这一功能。
一、使用 'in' 运算符
'in' 运算符是Python中最简单和直接的方法之一,用于判断一个字符串是否包含另一个字符串。它的语法非常简单,并且非常易于理解和使用。
示例代码:
text = "Hello, welcome to the world of Python programming!"
substring = "Python"
if substring in text:
print(f"The text contains the substring: {substring}")
else:
print(f"The text does not contain the substring: {substring}")
上述代码中,in
运算符用来检查 substring
是否在 text
中,如果存在则打印包含信息,否则打印不包含信息。
二、使用字符串方法
Python提供了一些内置的字符串方法,这些方法可以用来检查字符串是否包含特定的子字符串,例如 str.find()
和 str.index()
方法。
示例代码:
text = "Hello, welcome to the world of Python programming!"
substring = "Python"
使用find()方法
position = text.find(substring)
if position != -1:
print(f"The text contains the substring: {substring} at position {position}")
else:
print(f"The text does not contain the substring: {substring}")
使用index()方法
try:
position = text.index(substring)
print(f"The text contains the substring: {substring} at position {position}")
except ValueError:
print(f"The text does not contain the substring: {substring}")
在上述代码中,find()
方法返回子字符串的起始位置,如果未找到则返回 -1
。index()
方法与 find()
类似,但如果未找到子字符串则会抛出 ValueError
异常。
三、使用正则表达式
正则表达式是一种强大的字符串匹配工具,可以用于复杂的字符串搜索和匹配。Python的 re
模块提供了对正则表达式的支持。
示例代码:
import re
text = "Hello, welcome to the world of Python programming!"
pattern = r"Python"
if re.search(pattern, text):
print(f"The text contains the pattern: {pattern}")
else:
print(f"The text does not contain the pattern: {pattern}")
在上述代码中,re.search()
函数用于搜索匹配正则表达式的字符串。如果匹配成功则返回匹配对象,否则返回 None
。
四、判断多个字符
当需要判断一个字符串中是否包含多个特定的字符时,可以使用上述方法的组合。特别是使用正则表达式,可以轻松处理多个字符的匹配。
示例代码:
import re
text = "Hello, welcome to the world of Python programming!"
patterns = ["Python", "world", "welcome"]
检查所有模式是否都存在于文本中
for pattern in patterns:
if re.search(pattern, text):
print(f"The text contains the pattern: {pattern}")
else:
print(f"The text does not contain the pattern: {pattern}")
五、使用列表推导式
列表推导式是一种简洁而优雅的方式,可以用来检查多个字符或子字符串是否存在于一个字符串中。
示例代码:
text = "Hello, welcome to the world of Python programming!"
patterns = ["Python", "world", "welcome"]
使用列表推导式检查所有模式
results = [pattern for pattern in patterns if pattern in text]
if results:
print(f"The text contains the patterns: {', '.join(results)}")
else:
print("The text does not contain any of the patterns.")
在上述代码中,列表推导式用于生成包含所有匹配模式的列表。如果列表不为空,则打印匹配模式,否则打印未找到任何模式的信息。
六、使用all()函数
all()
函数用于检查是否所有的元素都满足某个条件,它可以与列表推导式或生成器表达式结合使用,来检查多个字符或子字符串是否全部存在于一个字符串中。
示例代码:
text = "Hello, welcome to the world of Python programming!"
patterns = ["Python", "world", "welcome"]
使用all()函数检查所有模式
if all(pattern in text for pattern in patterns):
print(f"The text contains all the patterns: {', '.join(patterns)}")
else:
print("The text does not contain all the patterns.")
在上述代码中,all()
函数用于检查所有的模式是否都存在于 text
中。如果所有模式都存在,则打印包含所有模式的信息,否则打印未包含所有模式的信息。
七、使用集合操作
集合操作是一种高效的方法,可以用来检查多个字符或子字符串是否存在于一个字符串中。集合操作的时间复杂度通常较低,适用于大规模数据处理。
示例代码:
text = "Hello, welcome to the world of Python programming!"
patterns = {"Python", "world", "welcome"}
将文本转换为集合
text_set = set(text.split())
使用集合操作检查所有模式
if patterns.issubset(text_set):
print(f"The text contains all the patterns: {', '.join(patterns)}")
else:
print("The text does not contain all the patterns.")
在上述代码中,issubset()
方法用于检查 patterns
是否是 text_set
的子集。如果是,则打印包含所有模式的信息,否则打印未包含所有模式的信息。
八、使用自定义函数
有时候,为了提高代码的可读性和复用性,可以编写自定义函数来处理多个字符或子字符串的判断逻辑。
示例代码:
def contains_all_patterns(text, patterns):
return all(pattern in text for pattern in patterns)
text = "Hello, welcome to the world of Python programming!"
patterns = ["Python", "world", "welcome"]
使用自定义函数检查所有模式
if contains_all_patterns(text, patterns):
print(f"The text contains all the patterns: {', '.join(patterns)}")
else:
print("The text does not contain all the patterns.")
在上述代码中,自定义函数 contains_all_patterns()
用于检查 patterns
是否都存在于 text
中。通过调用该函数,可以提高代码的可读性和复用性。
九、总结
在Python中判断多个字符或子字符串的方法有很多,每种方法都有其适用场景。简单的匹配可以使用 in
运算符或字符串方法,复杂的匹配可以使用正则表达式。列表推导式、all()
函数、集合操作以及自定义函数都提供了灵活且高效的解决方案。
在实际应用中,选择合适的方法取决于具体的需求和场景。 例如,当处理大规模数据时,集合操作的效率可能更高;当需要复杂匹配时,正则表达式是最佳选择。通过掌握这些方法,可以在Python中高效地处理字符串匹配和判断问题。
相关问答FAQs:
在Python中如何判断一个字符串中是否包含多个特定字符?
可以使用Python的in
运算符结合循环或列表推导式来检查一个字符串是否包含多个特定字符。通过遍历一个字符列表,您可以轻松判断每个字符是否在目标字符串中。例如,您可以这样写:
target_string = "hello world"
characters_to_check = ['h', 'w', 'x']
contains_all = all(char in target_string for char in characters_to_check)
这样,contains_all
将返回False
,因为target_string
中不包含字符'x'
。
如何在Python中找到一个字符串中出现的所有特定字符?
可以使用集合(set)来获取一个字符串中出现的特定字符。通过将字符串转换为集合,您可以找到字符的交集。例如:
target_string = "hello world"
characters_to_check = set('hew')
found_characters = set(target_string) & characters_to_check
found_characters
将返回{'h', 'e', 'w'}
,表示这些字符在目标字符串中存在。
在Python中如何判断字符串中多个字符的出现次数?
可以使用collections.Counter
来计算字符串中每个字符的出现次数。这样您可以轻松获取特定字符的数量。例如:
from collections import Counter
target_string = "hello world"
counter = Counter(target_string)
character_counts = {char: counter[char] for char in 'lo'}
此代码将创建一个字典,character_counts
中将包含字符'l'
和'o'
的出现次数,如{'l': 3, 'o': 2}
。
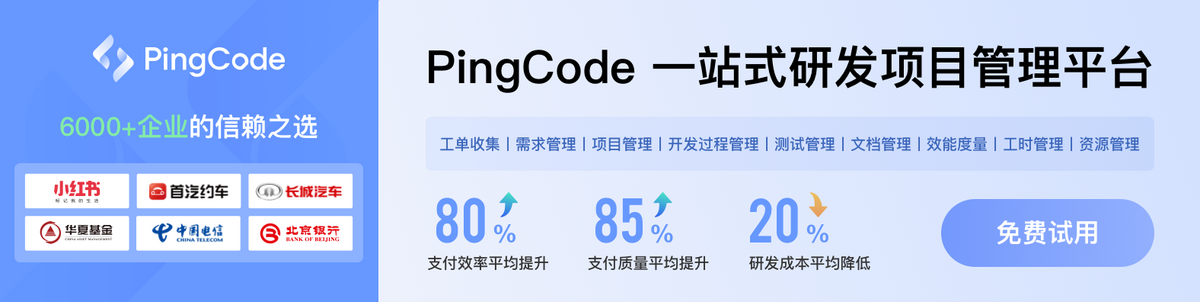