企业邮箱群发邮件的Python实现
企业邮箱群发邮件通常采用Python编程语言进行,主要通过SMTP协议与企业邮箱服务器进行通信。设置SMTP服务器、编写邮件内容、加载收件人列表、发送邮件是实现的主要步骤。下面将详细展开其中一个步骤:设置SMTP服务器。
设置SMTP服务器是实现企业邮箱群发邮件的重要步骤。SMTP(Simple Mail Transfer Protocol)是用于发送电子邮件的协议。你需要确保正确配置SMTP服务器地址、端口号、发件人邮箱及密码等信息。以下是详细的实现步骤。
一、设置SMTP服务器
-
获取SMTP服务器信息:不同的企业邮箱提供商有不同的SMTP服务器地址和端口号。你需要从企业邮箱的设置或帮助文档中获取这些信息。常见的企业邮箱SMTP服务器信息如下:
- Gmail:smtp.gmail.com,端口号587(TLS)或465(SSL)
- Outlook:smtp.office365.com,端口号587(TLS)
- Yahoo:smtp.mail.yahoo.com,端口号587(TLS)或465(SSL)
-
编写SMTP服务器配置代码:在Python中,可以使用
smtplib
库来配置SMTP服务器。以下是一个示例代码:import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
设置SMTP服务器地址和端口号
smtp_server = 'smtp.gmail.com'
smtp_port = 587
发件人邮箱和密码
sender_email = 'your_email@gmail.com'
sender_password = 'your_password'
创建SMTP对象并连接到服务器
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用TLS加密
server.login(sender_email, sender_password) # 登录邮箱
二、编写邮件内容
-
创建邮件对象:使用
email
库创建邮件对象,并设置发件人、收件人、主题和正文内容。以下是一个示例代码:# 创建邮件对象
message = MIMEMultipart()
message['From'] = sender_email
message['To'] = 'recipient@example.com' # 收件人邮箱
message['Subject'] = 'Test Email'
设置邮件正文内容
body = 'This is a test email sent from Python.'
message.attach(MIMEText(body, 'plain'))
-
添加附件(可选):如果需要,可以添加附件。以下是一个示例代码:
# 添加附件
filename = 'document.pdf'
with open(filename, 'rb') as attachment:
part = MIMEApplication(attachment.read(), Name=basename(filename))
part['Content-Disposition'] = f'attachment; filename="{basename(filename)}"'
message.attach(part)
三、加载收件人列表
-
从文件加载收件人列表:可以将收件人邮箱地址存储在文件中,并在Python代码中读取。以下是一个示例代码:
# 从文件加载收件人列表
recipients = []
with open('recipients.txt', 'r') as file:
for line in file:
recipients.append(line.strip())
-
手动添加收件人地址:也可以在代码中手动添加收件人地址。以下是一个示例代码:
# 手动添加收件人地址
recipients = ['recipient1@example.com', 'recipient2@example.com', 'recipient3@example.com']
四、发送邮件
-
循环发送邮件:遍历收件人列表,逐个发送邮件。以下是一个示例代码:
# 遍历收件人列表,逐个发送邮件
for recipient in recipients:
message['To'] = recipient
server.sendmail(sender_email, recipient, message.as_string())
-
关闭SMTP服务器连接:邮件发送完成后,关闭SMTP服务器连接。以下是一个示例代码:
# 关闭SMTP服务器连接
server.quit()
五、错误处理和日志记录
-
添加错误处理:在发送邮件的过程中,可能会遇到各种错误(如网络故障、邮箱账号或密码错误等)。需要添加错误处理代码,捕获并记录错误信息。以下是一个示例代码:
import logging
配置日志记录
logging.basicConfig(filename='email.log', level=logging.ERROR)
遍历收件人列表,逐个发送邮件
for recipient in recipients:
try:
message['To'] = recipient
server.sendmail(sender_email, recipient, message.as_string())
except Exception as e:
logging.error(f'Failed to send email to {recipient}: {e}')
-
记录发送成功的邮件:可以在日志中记录发送成功的邮件,以便后续查询。以下是一个示例代码:
# 配置日志记录
logging.basicConfig(filename='email.log', level=logging.INFO)
遍历收件人列表,逐个发送邮件
for recipient in recipients:
try:
message['To'] = recipient
server.sendmail(sender_email, recipient, message.as_string())
logging.info(f'Successfully sent email to {recipient}')
except Exception as e:
logging.error(f'Failed to send email to {recipient}: {e}')
六、示例代码汇总
以下是一个完整的示例代码,展示了如何使用Python实现企业邮箱群发邮件:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
import logging
设置SMTP服务器地址和端口号
smtp_server = 'smtp.gmail.com'
smtp_port = 587
发件人邮箱和密码
sender_email = 'your_email@gmail.com'
sender_password = 'your_password'
创建SMTP对象并连接到服务器
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用TLS加密
server.login(sender_email, sender_password) # 登录邮箱
创建邮件对象
message = MIMEMultipart()
message['From'] = sender_email
message['Subject'] = 'Test Email'
设置邮件正文内容
body = 'This is a test email sent from Python.'
message.attach(MIMEText(body, 'plain'))
从文件加载收件人列表
recipients = []
with open('recipients.txt', 'r') as file:
for line in file:
recipients.append(line.strip())
配置日志记录
logging.basicConfig(filename='email.log', level=logging.INFO)
遍历收件人列表,逐个发送邮件
for recipient in recipients:
try:
message['To'] = recipient
server.sendmail(sender_email, recipient, message.as_string())
logging.info(f'Successfully sent email to {recipient}')
except Exception as e:
logging.error(f'Failed to send email to {recipient}: {e}')
关闭SMTP服务器连接
server.quit()
通过上述代码,您可以实现企业邮箱群发邮件。在实际应用中,还需要根据具体需求进行调整和优化,例如支持HTML格式的邮件正文、自定义邮件内容模板、定时发送邮件等。希望这篇文章对您有所帮助。
相关问答FAQs:
如何使用Python发送群发邮件?
在Python中,可以通过内置的smtplib
库来实现群发邮件的功能。您需要设置SMTP服务器的连接,并使用sendmail
方法发送邮件。确保您的企业邮箱支持SMTP,并获取相应的服务器地址和端口号。以下是基本步骤:
- 导入
smtplib
和email
库。 - 创建SMTP对象并连接到邮件服务器。
- 登录到企业邮箱。
- 使用循环将邮件发送给多个收件人。
群发邮件时需要注意哪些事项?
在进行群发邮件时,有几个关键点需要关注。首先,确保收件人列表遵循隐私规范,避免泄露个人信息。其次,使用个性化的邮件内容可以提高打开率,增加用户的参与感。此外,遵循反垃圾邮件法规,确保邮件内容符合相应的法律要求,从而避免被标记为垃圾邮件。
如何处理邮件发送失败的情况?
在发送群发邮件时,可能会遇到一些邮件发送失败的情况。可以在Python脚本中添加异常处理机制,以捕获并记录发送失败的邮件地址和原因。通过使用try...except
结构,可以确保脚本在遇到错误时不会崩溃,并能够输出详细的错误信息供后续排查。同时,可以设置重试机制,尝试重新发送失败的邮件,确保信息能够顺利送达。
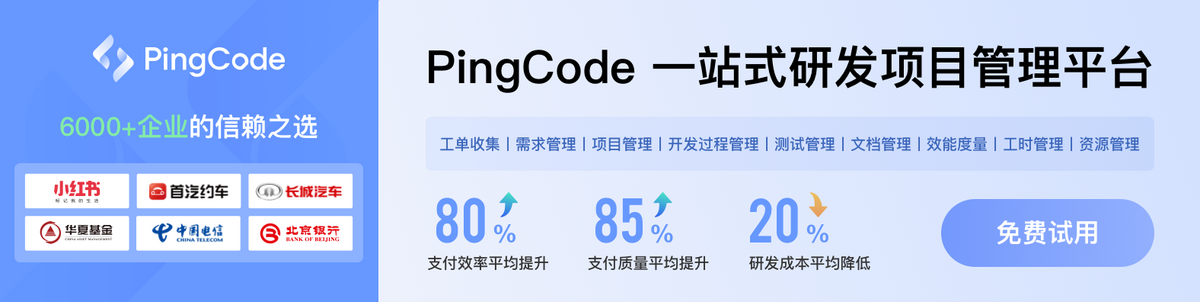