在Python中,逐字从文件读写到屏幕的方法有很多种,常见的方法包括使用内建函数open()、read()、write()等。逐字读取、写入文件内容可以使用读取文件内容并逐字遍历、使用循环读取等方式。
详细描述:
一种常见的方法是使用Python的内建函数open()打开文件,然后使用read()函数逐个读取字符并打印到屏幕。我们可以通过一个简单的循环来实现逐字读取文件内容并将其打印到屏幕上。接下来我将详细描述这种方法的实现步骤。
一、打开文件
首先,使用open()函数以只读模式打开文件。open()函数有两个主要参数:文件路径和打开模式。对于只读模式,我们使用'r':
file_path = 'example.txt'
file = open(file_path, 'r')
二、逐字读取文件内容
一旦文件打开,我们可以使用read()函数逐字读取文件内容。为了逐字读取,我们可以在一个循环中调用read(1),每次读取一个字符,直到读取到文件末尾:
while True:
char = file.read(1)
if not char:
break
print(char, end='')
在这个循环中,read(1)每次读取一个字符并将其存储在变量char中。如果读取到文件末尾,char将为空字符串,这时我们使用break语句退出循环。print(char, end='')用于逐字打印字符到屏幕,end=''参数确保每个字符在同一行显示。
三、关闭文件
读取操作完成后,记得关闭文件以释放系统资源:
file.close()
示例代码
以下是完整的Python代码示例:
file_path = 'example.txt'
file = open(file_path, 'r')
while True:
char = file.read(1)
if not char:
break
print(char, end='')
file.close()
四、逐字写入文件内容
如果需要将逐字读入的内容写入另一个文件,我们可以在读取的同时打开另一个文件以写入模式进行写操作:
input_file_path = 'example.txt'
output_file_path = 'output.txt'
input_file = open(input_file_path, 'r')
output_file = open(output_file_path, 'w')
while True:
char = input_file.read(1)
if not char:
break
output_file.write(char)
input_file.close()
output_file.close()
五、逐字读写的应用场景
逐字读写操作在处理大文件时非常有用,因为它可以减少内存消耗。逐字处理也适用于需要精确控制文件内容的情况,比如文本处理、字符编码转换等。
六、使用with语句管理文件
为了确保文件在操作完成后总是能被正确关闭,我们可以使用with语句来管理文件。with语句会在代码块结束后自动关闭文件:
input_file_path = 'example.txt'
output_file_path = 'output.txt'
with open(input_file_path, 'r') as input_file, open(output_file_path, 'w') as output_file:
while True:
char = input_file.read(1)
if not char:
break
print(char, end='')
output_file.write(char)
七、处理文件异常
在处理文件操作时,可能会遇到各种异常情况,如文件不存在、权限不足等。我们可以使用try…except语句来捕获并处理这些异常:
try:
input_file_path = 'example.txt'
output_file_path = 'output.txt'
with open(input_file_path, 'r') as input_file, open(output_file_path, 'w') as output_file:
while True:
char = input_file.read(1)
if not char:
break
print(char, end='')
output_file.write(char)
except FileNotFoundError:
print("Error: The file was not found.")
except IOError:
print("Error: An I/O error occurred.")
八、逐字读写与字符编码
处理不同字符编码的文件时,需要确保使用正确的编码方式。open()函数有一个encoding参数,可以指定文件的字符编码,例如utf-8:
input_file_path = 'example.txt'
output_file_path = 'output.txt'
with open(input_file_path, 'r', encoding='utf-8') as input_file, open(output_file_path, 'w', encoding='utf-8') as output_file:
while True:
char = input_file.read(1)
if not char:
break
print(char, end='')
output_file.write(char)
九、逐字读写的性能优化
逐字读写可能会导致性能问题,因为每次读取一个字符会触发I/O操作。为了提高性能,可以使用较大缓冲区进行批量读取,然后逐字处理缓冲区中的字符:
input_file_path = 'example.txt'
output_file_path = 'output.txt'
buffer_size = 1024 # 缓冲区大小
with open(input_file_path, 'r', encoding='utf-8') as input_file, open(output_file_path, 'w', encoding='utf-8') as output_file:
while True:
buffer = input_file.read(buffer_size)
if not buffer:
break
for char in buffer:
print(char, end='')
output_file.write(char)
十、总结
逐字读取和写入文件是Python文件操作中的基本技能,通过上述方法可以有效地实现文件内容的逐字读写。掌握这些方法和技巧,可以帮助我们在处理大文件、字符处理、文本转换等场景中灵活应对各种需求。此外,注意文件操作的性能优化和异常处理也是编写健壮、高效代码的关键。
相关问答FAQs:
如何在Python中逐字读取文件内容并显示在屏幕上?
在Python中,可以使用内置的open()
函数逐字读取文件。使用文件对象的read()
方法,可以逐个字符地获取文件内容,并通过print()
函数将其输出到屏幕。例如:
with open('filename.txt', 'r') as file:
while (char := file.read(1)):
print(char, end='')
这种方法允许您逐个字符地读取文件,并立即在屏幕上显示。
有没有更高效的方式在Python中逐字写入文件?
虽然逐字读取文件内容是一种常见的方法,但可以使用缓冲技术提高效率。通过读取一定数量的字符,然后再打印,可以减少文件操作次数,进而提升性能。例如:
with open('filename.txt', 'r') as file:
while True:
chars = file.read(1024) # 一次读取1024个字符
if not chars:
break
print(chars, end='')
这种方法适合处理较大的文件,能够更高效地在屏幕上显示内容。
在Python中逐字读取文件时,如何处理编码问题?
在读取文件时,编码问题可能会导致字符显示错误。使用open()
函数时,可以指定encoding
参数,以确保正确处理不同编码的文件。例如:
with open('filename.txt', 'r', encoding='utf-8') as file:
while (char := file.read(1)):
print(char, end='')
指定合适的编码方式有助于避免乱码,确保文件内容能够正确显示。
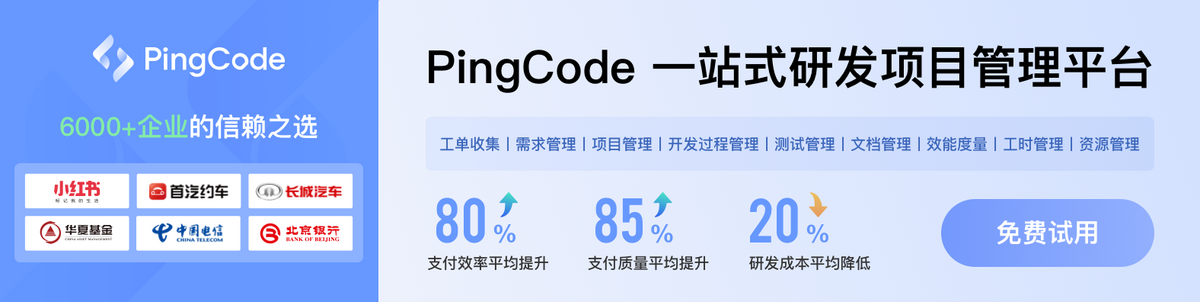