用Python如何跑局部最高点可以通过以下方法实现:定义目标函数、选择合适的优化算法、设置初始点、运行算法。这些步骤可以帮助我们找到函数在给定区间内的局部最高点。其中选择合适的优化算法尤其重要,因为不同的优化算法适用于不同的问题。下面将详细介绍如何选择和应用这些优化算法。
局部最优化是指在某个特定区间内寻找函数的极值点,常见的方法包括梯度上升法、牛顿法和模拟退火法等。选择合适的优化算法取决于函数的性质和问题的具体要求,例如函数是否光滑、是否有多个局部极值点等。本文将介绍几种常见的优化算法,并给出在Python中实现这些算法的示例代码。
一、梯度上升法
梯度上升法是一种迭代优化算法,通过沿着目标函数梯度的方向进行搜索,逐步逼近局部最高点。梯度上升法适用于光滑连续的函数。
1.1 定义目标函数和梯度
首先,我们需要定义目标函数和其梯度。假设目标函数为f(x),其梯度为f'(x)。
import numpy as np
def f(x):
return -x2 + 4*x
def gradient(x):
return -2*x + 4
1.2 实现梯度上升法
接下来,我们实现梯度上升法。设定初始点、学习率和迭代次数。
def gradient_ascent(starting_point, learning_rate, iterations):
x = starting_point
for _ in range(iterations):
grad = gradient(x)
x += learning_rate * grad
return x
1.3 运行梯度上升法
设置初始点为0,学习率为0.1,迭代次数为100,运行梯度上升法找到局部最高点。
starting_point = 0
learning_rate = 0.1
iterations = 100
optimal_x = gradient_ascent(starting_point, learning_rate, iterations)
optimal_y = f(optimal_x)
print(f"局部最高点: x = {optimal_x}, y = {optimal_y}")
二、牛顿法
牛顿法是一种基于二阶导数的优化算法,通过泰勒展开近似目标函数,迭代更新以逼近局部最高点。牛顿法适用于二阶导数存在且连续的函数。
2.1 定义目标函数及其一阶和二阶导数
def f(x):
return -x2 + 4*x
def gradient(x):
return -2*x + 4
def hessian(x):
return -2
2.2 实现牛顿法
def newton_method(starting_point, iterations):
x = starting_point
for _ in range(iterations):
grad = gradient(x)
hess = hessian(x)
x -= grad / hess
return x
2.3 运行牛顿法
设定初始点为0,迭代次数为10,运行牛顿法找到局部最高点。
starting_point = 0
iterations = 10
optimal_x = newton_method(starting_point, iterations)
optimal_y = f(optimal_x)
print(f"局部最高点: x = {optimal_x}, y = {optimal_y}")
三、模拟退火法
模拟退火法是一种全局优化算法,通过模拟物理退火过程,在搜索过程中允许偶尔接受劣解,以跳出局部极值点,逼近全局最优解。
3.1 定义目标函数
import numpy as np
def f(x):
return -x2 + 4*x
3.2 实现模拟退火法
def simulated_annealing(starting_point, temperature, cooling_rate, iterations):
x = starting_point
current_value = f(x)
best_x = x
best_value = current_value
for _ in range(iterations):
new_x = x + np.random.uniform(-1, 1)
new_value = f(new_x)
acceptance_probability = np.exp((new_value - current_value) / temperature)
if new_value > current_value or np.random.rand() < acceptance_probability:
x = new_x
current_value = new_value
if current_value > best_value:
best_x = x
best_value = current_value
temperature *= cooling_rate
return best_x
3.3 运行模拟退火法
设定初始点为0,初始温度为100,冷却率为0.99,迭代次数为1000,运行模拟退火法找到局部最高点。
starting_point = 0
temperature = 100
cooling_rate = 0.99
iterations = 1000
optimal_x = simulated_annealing(starting_point, temperature, cooling_rate, iterations)
optimal_y = f(optimal_x)
print(f"局部最高点: x = {optimal_x}, y = {optimal_y}")
四、粒子群优化
粒子群优化(PSO)是一种基于群体智能的优化算法,通过模拟鸟群觅食行为,多个粒子在解空间中同时搜索,依靠全局最优解和个体最优解的信息更新位置。
4.1 定义目标函数
def f(x):
return -x2 + 4*x
4.2 实现粒子群优化
class Particle:
def __init__(self, x):
self.position = x
self.velocity = np.random.uniform(-1, 1)
self.best_position = x
self.best_value = f(x)
def update_velocity(self, global_best_position, w, c1, c2):
inertia = w * self.velocity
cognitive = c1 * np.random.rand() * (self.best_position - self.position)
social = c2 * np.random.rand() * (global_best_position - self.position)
self.velocity = inertia + cognitive + social
def update_position(self):
self.position += self.velocity
value = f(self.position)
if value > self.best_value:
self.best_value = value
self.best_position = self.position
def pso(num_particles, iterations, w, c1, c2):
particles = [Particle(np.random.uniform(-10, 10)) for _ in range(num_particles)]
global_best_position = max(particles, key=lambda p: p.best_value).best_position
for _ in range(iterations):
for particle in particles:
particle.update_velocity(global_best_position, w, c1, c2)
particle.update_position()
global_best_position = max(particles, key=lambda p: p.best_value).best_position
return global_best_position
4.3 运行粒子群优化
设定粒子数量为30,迭代次数为100,惯性权重为0.5,个体和社会加速度系数分别为1.5和1.5,运行粒子群优化找到局部最高点。
num_particles = 30
iterations = 100
w = 0.5
c1 = 1.5
c2 = 1.5
optimal_x = pso(num_particles, iterations, w, c1, c2)
optimal_y = f(optimal_x)
print(f"局部最高点: x = {optimal_x}, y = {optimal_y}")
五、总结
通过上述几种方法,我们可以在Python中实现不同的优化算法,以找到目标函数的局部最高点。梯度上升法适用于光滑连续的函数,牛顿法适用于二阶导数存在且连续的函数,模拟退火法和粒子群优化适用于全局优化问题。选择合适的优化算法取决于具体问题的性质和要求。希望本文的介绍能帮助您在实际应用中有效地找到局部最高点。
相关问答FAQs:
如何使用Python找到局部最高点的算法?
在Python中,找到局部最高点的常用方法是通过遍历数组,检查每个元素是否比它的相邻元素大。可以使用简单的循环,结合条件判断来实现这一目标。此外,NumPy库中的一些函数也能有效帮助识别局部最高点。以下是一个简单的示例代码:
import numpy as np
def find_local_peaks(data):
peaks = []
for i in range(1, len(data) - 1):
if data[i] > data[i - 1] and data[i] > data[i + 1]:
peaks.append(data[i])
return peaks
data = [1, 3, 2, 5, 4, 6, 5]
local_peaks = find_local_peaks(data)
print(local_peaks) # 输出局部最高点
在处理大数据时,如何优化局部最高点的查找?
在处理大规模数据时,优化查找局部最高点的效率至关重要。一种常用的方法是采用二分查找算法,通过不断缩小查找范围来快速定位峰值。另一种方法是使用信号处理库,如SciPy中的find_peaks
函数,这个函数提供了多种参数设置,能够灵活适应不同数据特点,从而提升效率。
局部最高点在数据分析中有什么应用?
局部最高点的识别在多个领域中具有重要意义,例如在信号处理领域,可以用来检测音频信号中的音符;在市场分析中,局部最高点能帮助识别价格波动的趋势;在生物信息学中,局部最高点的查找可以用于基因表达数据分析。因此,掌握局部最高点的查找方法对从事相关领域的研究人员非常重要。
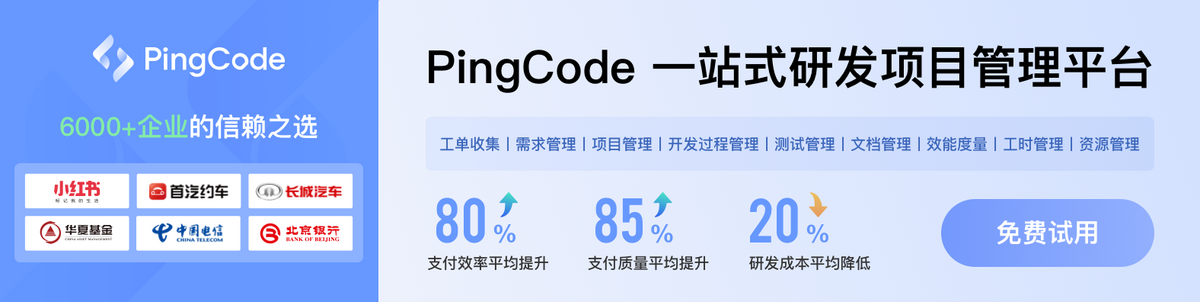