在Python中删除文件夹内容有多种方法,包括使用内置库os
、shutil
以及第三方库pathlib
。 下面我们将详细解释这些方法,并提供代码示例和注意事项。
一、使用os
模块
os
模块是Python的标准库之一,提供了与操作系统进行交互的多种方法,包括文件和目录操作。要删除文件夹内容,可以使用os.listdir()
列出目录中的文件和子目录,然后遍历并删除它们。
import os
def delete_folder_contents(folder_path):
# 获取目录中的所有文件和子目录
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename)
try:
if os.path.isfile(file_path) or os.path.islink(file_path):
os.unlink(file_path) # 删除文件或符号链接
elif os.path.isdir(file_path):
os.rmdir(file_path) # 删除空目录
except Exception as e:
print(f'删除 {file_path} 时发生错误: {e}')
示例用法
folder_path = '/path/to/your/folder'
delete_folder_contents(folder_path)
注意:os.rmdir
只能删除空目录。如果目录中有文件或子目录,需要先递归删除它们。
二、使用shutil
模块
shutil
模块提供了一个更高级的文件操作接口,可以递归删除目录及其内容。shutil.rmtree()
是一个强大的函数,可以删除整个目录树。
import shutil
def delete_folder_contents(folder_path):
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename)
try:
if os.path.isfile(file_path) or os.path.islink(file_path):
os.unlink(file_path)
elif os.path.isdir(file_path):
shutil.rmtree(file_path)
except Exception as e:
print(f'删除 {file_path} 时发生错误: {e}')
示例用法
folder_path = '/path/to/your/folder'
delete_folder_contents(folder_path)
shutil.rmtree()
将递归地删除目录及其所有内容,因此在删除文件夹时需要特别小心,以免误删重要数据。
三、使用pathlib
模块
pathlib
是Python 3.4引入的一个模块,为面向对象的文件系统路径操作提供了更加简洁和直观的接口。它使路径操作更具可读性。
from pathlib import Path
def delete_folder_contents(folder_path):
path = Path(folder_path)
for item in path.iterdir():
try:
if item.is_file() or item.is_symlink():
item.unlink()
elif item.is_dir():
shutil.rmtree(item)
except Exception as e:
print(f'删除 {item} 时发生错误: {e}')
示例用法
folder_path = '/path/to/your/folder'
delete_folder_contents(folder_path)
四、删除文件夹内容的注意事项
- 备份数据:在执行删除操作之前,建议备份重要数据,以防误删。
- 权限问题:确保您有权限删除指定目录中的文件和子目录。
- 错误处理:在删除文件或目录时,可能会遇到各种错误(如文件被占用、权限不足等),应做好错误处理。
五、示例代码及解释
import os
import shutil
from pathlib import Path
def delete_folder_contents(folder_path):
# 使用os模块删除文件夹内容
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename)
try:
if os.path.isfile(file_path) or os.path.islink(file_path):
os.unlink(file_path) # 删除文件或符号链接
elif os.path.isdir(file_path):
os.rmdir(file_path) # 删除空目录
except Exception as e:
print(f'使用os模块删除 {file_path} 时发生错误: {e}')
# 使用shutil模块删除文件夹内容
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename)
try:
if os.path.isfile(file_path) or os.path.islink(file_path):
os.unlink(file_path)
elif os.path.isdir(file_path):
shutil.rmtree(file_path)
except Exception as e:
print(f'使用shutil模块删除 {file_path} 时发生错误: {e}')
# 使用pathlib模块删除文件夹内容
path = Path(folder_path)
for item in path.iterdir():
try:
if item.is_file() or item.is_symlink():
item.unlink()
elif item.is_dir():
shutil.rmtree(item)
except Exception as e:
print(f'使用pathlib模块删除 {item} 时发生错误: {e}')
示例用法
folder_path = '/path/to/your/folder'
delete_folder_contents(folder_path)
通过这篇文章,我们详细介绍了如何在Python中删除文件夹内容,分别使用了os
、shutil
和pathlib
模块。每种方法都有其优点,选择适合自己需求的方法即可。同时,注意在删除文件夹内容时做好数据备份和错误处理,以免造成数据丢失或其他问题。
相关问答FAQs:
在Python中,如何安全地删除文件夹内的所有内容?
在Python中,可以使用shutil
模块的rmtree
函数来安全地删除文件夹及其所有内容。使用时,需要确保你有适当的权限,并且确认该操作是必要的。示例代码如下:
import shutil
shutil.rmtree('your_directory_path')
在执行此操作之前,建议备份重要数据,以防意外删除。
删除文件夹内容时,如何避免删除整个文件夹?
如果希望仅删除文件夹内的所有文件而保留文件夹本身,可以使用os
模块。通过遍历文件夹内的所有文件和子文件夹,可以逐一删除它们。示例代码如下:
import os
folder_path = 'your_directory_path'
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename)
if os.path.isfile(file_path):
os.remove(file_path)
elif os.path.isdir(file_path):
shutil.rmtree(file_path)
这种方法确保了文件夹仍然存在。
删除文件夹内容后,如何确认删除操作成功?
在执行删除操作后,可以再次列出文件夹内的内容来确认删除是否成功。使用os.listdir()
函数可以获取当前文件夹的内容,并通过判断返回的列表是否为空来确认。示例代码如下:
remaining_files = os.listdir('your_directory_path')
if not remaining_files:
print("文件夹内容已成功删除。")
else:
print("文件夹内仍然有内容。")
这种方式提供了一种简单的验证机制,确保您的删除操作按预期执行。
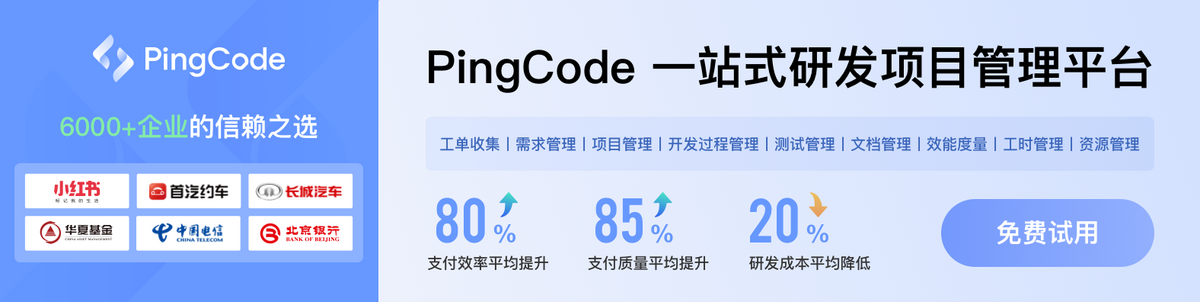