用Python写辞职信代码的方法包括:使用Python的文本处理功能、使用模板引擎生成内容、利用Python自动化发送邮件。 下面我将详细描述其中一种方法,使用Python生成辞职信文本并发送邮件。
一、生成辞职信内容
Python可以通过简单的字符串操作来生成辞职信内容。我们可以定义一个辞职信模板,并使用字符串格式化来填充具体的内容。
def generate_resignation_letter(name, position, company, last_day):
template = f"""
Dear {supervisor_name},
I am writing to formally resign from my position as {position} at {company}. My last day of employment will be {last_day}.
This was not an easy decision for me to make. The past [duration] years at {company} have been incredibly rewarding. I have enjoyed working with the team and have learned so much during my time here.
I will do everything possible to make the transition as smooth as possible. Please let me know how I can help during this time.
Thank you for the opportunity to be part of the team.
Sincerely,
{name}
"""
return template
二、保存辞职信为文件
生成辞职信后,可以将其保存到一个文件中,这样可以方便发送或打印。
def save_letter_to_file(letter, filename="resignation_letter.txt"):
with open(filename, "w") as file:
file.write(letter)
三、使用模板引擎生成辞职信
对于更复杂的辞职信模板,可以使用模板引擎如Jinja2来生成内容。Jinja2可以让你定义模板,并使用变量来填充内容。
from jinja2 import Template
def generate_resignation_letter_with_jinja(name, position, company, last_day, supervisor_name):
template = Template("""
Dear {{ supervisor_name }},
I am writing to formally resign from my position as {{ position }} at {{ company }}. My last day of employment will be {{ last_day }}.
This was not an easy decision for me to make. The past [duration] years at {{ company }} have been incredibly rewarding. I have enjoyed working with the team and have learned so much during my time here.
I will do everything possible to make the transition as smooth as possible. Please let me know how I can help during this time.
Thank you for the opportunity to be part of the team.
Sincerely,
{{ name }}
""")
return template.render(name=name, position=position, company=company, last_day=last_day, supervisor_name=supervisor_name)
四、发送辞职信邮件
Python的smtplib库可以用于发送邮件。以下是一个示例,展示如何使用smtplib发送辞职信邮件。
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_resignation_email(sender_email, sender_password, receiver_email, subject, body):
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = receiver_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
try:
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(sender_email, sender_password)
text = msg.as_string()
server.sendmail(sender_email, receiver_email, text)
server.quit()
print("Email sent successfully")
except Exception as e:
print(f"Failed to send email: {e}")
Example usage
name = "John Doe"
position = "Software Engineer"
company = "Tech Corp"
last_day = "2023-12-31"
supervisor_name = "Jane Smith"
letter = generate_resignation_letter_with_jinja(name, position, company, last_day, supervisor_name)
save_letter_to_file(letter)
sender_email = "your_email@gmail.com"
sender_password = "your_password"
receiver_email = "supervisor_email@company.com"
subject = "Resignation Letter"
send_resignation_email(sender_email, sender_password, receiver_email, subject, letter)
五、总结
用Python写辞职信代码可以简化辞职信的生成和发送过程。通过使用简单的字符串操作、模板引擎以及自动化邮件发送,您可以确保辞职信的内容准确无误并及时递交给相关人员。
希望以上方法和示例代码能帮助您轻松生成和发送辞职信。如果您有任何进一步的问题,欢迎继续探讨。
相关问答FAQs:
如何用Python生成个性化的辞职信?
使用Python编写辞职信的代码可以通过字符串格式化来实现个性化。您可以创建一个模板,使用用户输入的信息(如姓名、职位、公司名和辞职日期)来填充模板内容。以下是一个简单的示例:
def generate_resignation_letter(name, position, company, resignation_date):
letter = f"""
{name}
{position}
{company}
{resignation_date}
尊敬的经理:
我写信是为了正式通知您我决定辞去我的{position}职位。感谢您在我任职期间的支持和指导。
祝一切顺利!
此致,
{name}
"""
return letter
# 示例输入
name = input("请输入您的姓名:")
position = input("请输入您的职位:")
company = input("请输入公司名:")
resignation_date = input("请输入辞职日期:")
print(generate_resignation_letter(name, position, company, resignation_date))
使用Python编写辞职信时需要注意哪些细节?
在编写辞职信时,保持礼貌和专业是至关重要的。确保信件简洁明了,避免使用负面情绪或不满的言辞。同时,使用适当的格式,包括您的联系信息和日期,以确保信件看起来正式。
如何将辞职信保存为文本文件?
您可以使用Python的文件操作功能,将生成的辞职信保存到文本文件中。通过以下代码,可以将辞职信写入一个名为“resignation_letter.txt”的文件:
with open("resignation_letter.txt", "w") as file:
file.write(generate_resignation_letter(name, position, company, resignation_date))
这样,您在终端生成的辞职信就会被保存在本地文件中,方便随时查看和发送。
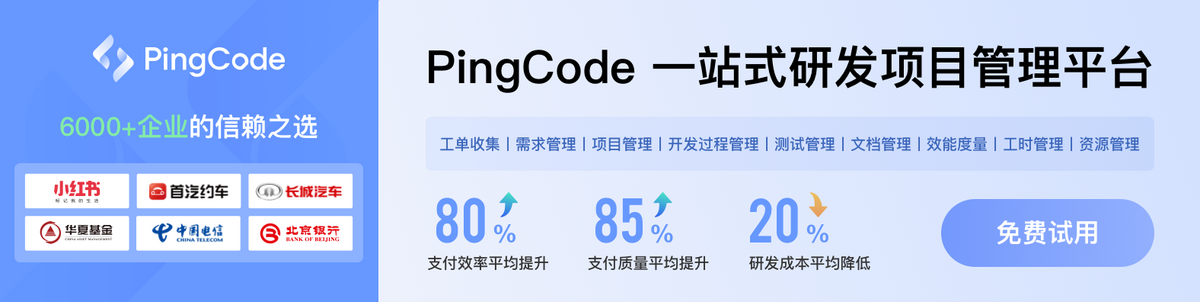