Python定时器如何控制启停:通过 threading.Timer 类、使用 schedule 库、创建自定义类。以下将详细介绍如何利用这些方法控制 Python 定时器的启停。
在编程中,定时器是一个非常有用的工具,可以让我们在特定时间间隔后执行某些操作。Python 提供了多种控制定时器的方法,本文将探讨如何使用 threading.Timer
类、schedule
库以及自定义类来实现定时器的启动和停止。
一、通过 threading.Timer 类
Python 的 threading
模块提供了 Timer
类,可以用来在指定时间后执行一个函数。以下是如何使用 threading.Timer
类控制定时器启停的示例。
1、创建定时器
threading.Timer
类的基本用法非常简单。首先,我们需要导入 threading
模块,并创建一个定时器对象:
import threading
def hello():
print("Hello, World!")
创建一个在 5 秒后执行 hello 函数的定时器
timer = threading.Timer(5.0, hello)
2、启动定时器
调用定时器对象的 start
方法可以启动定时器:
timer.start()
3、停止定时器
如果在定时器到期之前需要取消它,可以调用定时器对象的 cancel
方法:
timer.cancel()
示例代码
以下是一个完整的示例代码,展示了如何创建、启动和停止定时器:
import threading
def hello():
print("Hello, World!")
创建一个在 5 秒后执行 hello 函数的定时器
timer = threading.Timer(5.0, hello)
启动定时器
timer.start()
在 2 秒后取消定时器
threading.Timer(2.0, timer.cancel).start()
在这个示例中,定时器被设置为在 5 秒后执行 hello
函数,但在 2 秒后被取消,因此 hello
函数不会被执行。
二、使用 schedule 库
schedule
库是一个轻量级的 Python 任务调度库,非常适合用来定时运行任务。与 threading.Timer
类不同,schedule
库更适合用于需要反复执行的任务。
1、安装 schedule 库
首先,确保已安装 schedule
库:
pip install schedule
2、创建定时任务
创建定时任务非常简单,可以使用 schedule.every
方法创建任务:
import schedule
import time
def job():
print("Hello, World!")
每 5 秒执行一次 job 函数
schedule.every(5).seconds.do(job)
3、运行定时任务
要运行定时任务,需要在一个循环中调用 schedule.run_pending
方法:
while True:
schedule.run_pending()
time.sleep(1)
示例代码
以下是一个完整的示例代码,展示了如何使用 schedule
库创建和运行定时任务:
import schedule
import time
def job():
print("Hello, World!")
每 5 秒执行一次 job 函数
schedule.every(5).seconds.do(job)
运行定时任务
while True:
schedule.run_pending()
time.sleep(1)
在这个示例中,job
函数将每隔 5 秒被执行一次。
三、创建自定义类
除了使用 threading.Timer
类和 schedule
库,我们还可以创建自定义类来实现定时器的启动和停止。
1、定义自定义定时器类
首先,我们需要定义一个自定义定时器类:
import threading
class CustomTimer:
def __init__(self, interval, function):
self.interval = interval
self.function = function
self.timer = None
def start(self):
self.timer = threading.Timer(self.interval, self.function)
self.timer.start()
def stop(self):
if self.timer is not None:
self.timer.cancel()
self.timer = None
2、使用自定义定时器类
创建和使用自定义定时器类非常简单:
def hello():
print("Hello, World!")
创建一个自定义定时器,每 5 秒执行一次 hello 函数
custom_timer = CustomTimer(5.0, hello)
启动定时器
custom_timer.start()
在 2 秒后停止定时器
threading.Timer(2.0, custom_timer.stop).start()
示例代码
以下是一个完整的示例代码,展示了如何使用自定义定时器类:
import threading
class CustomTimer:
def __init__(self, interval, function):
self.interval = interval
self.function = function
self.timer = None
def start(self):
self.timer = threading.Timer(self.interval, self.function)
self.timer.start()
def stop(self):
if self.timer is not None:
self.timer.cancel()
self.timer = None
def hello():
print("Hello, World!")
创建一个自定义定时器,每 5 秒执行一次 hello 函数
custom_timer = CustomTimer(5.0, hello)
启动定时器
custom_timer.start()
在 2 秒后停止定时器
threading.Timer(2.0, custom_timer.stop).start()
在这个示例中,自定义定时器被设置为在 5 秒后执行 hello
函数,但在 2 秒后被取消,因此 hello
函数不会被执行。
四、总结
在本文中,我们探讨了三种控制 Python 定时器启停的方法:使用 threading.Timer
类、使用 schedule
库以及创建自定义类。这些方法各有优缺点,可以根据具体需求选择合适的方法来实现定时器的启动和停止。
使用 threading.Timer
类:适合一次性任务,简单易用。
使用 schedule
库:适合需要反复执行的任务,功能强大。
创建自定义类:灵活性高,可以根据需求自定义定时器的行为。
通过这些方法,可以轻松实现 Python 定时器的控制,满足各种不同的需求。无论是一次性任务还是反复执行的任务,这些方法都能提供有效的解决方案。
相关问答FAQs:
如何在Python中实现定时器的启停控制?
在Python中,可以使用threading.Timer
类来创建一个定时器。通过定义一个函数作为回调,可以在指定的时间后执行该函数。要控制定时器的启停,可以通过设置一个标志位来判断是否需要继续执行,或者通过调用定时器的cancel()
方法来停止定时器。
定时器可以设置多长时间?
在Python中,threading.Timer
的时间参数可以设置为任意非负浮点数,表示延迟的秒数。无论是短暂的几秒钟,还是长时间的几小时,都可以根据需要进行调整。需要注意的是,定时器的时间精度受系统调度的影响,可能会有轻微延迟。
如果定时器执行的任务需要传递参数,该如何处理?
在使用threading.Timer
时,可以通过args
参数来传递任务所需的参数。该参数应该是一个元组,包含所有需要传递给回调函数的参数。这样,在定时器到达设定时间后,回调函数将能够接收到这些参数并进行相应的处理。
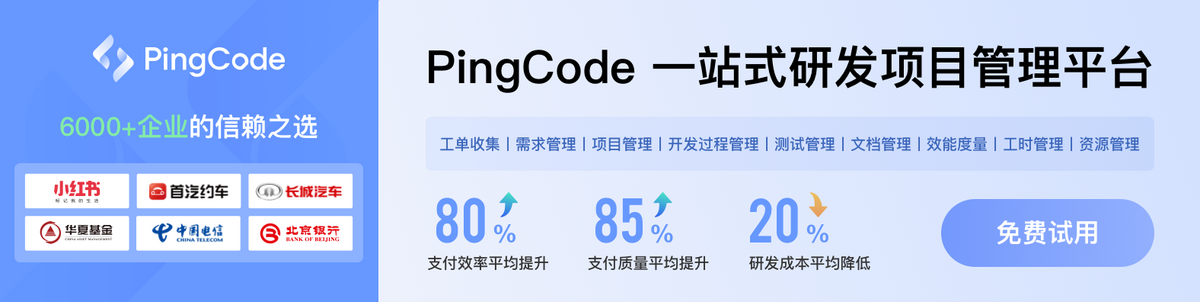