在Python中,可以通过使用内置函数len()
来获取字符串的长度。使用len()
函数非常简单,只需要将字符串作为参数传递给该函数,它将返回字符串的长度。例如,如果我们有一个字符串"Hello, World!"
,我们可以通过调用len("Hello, World!")
来获取其长度。接下来我们将详细描述如何在不同情况下使用len()
函数获取字符串的长度,并探索一些相关的高级用法。
一、基本用法
在Python中,获取字符串长度的最基本方法是使用len()
函数。以下是一个简单的示例:
my_string = "Hello, World!"
length = len(my_string)
print(f"The length of the string is: {length}")
在这个例子中,我们首先定义了一个字符串my_string
,然后使用len()
函数来计算字符串的长度,并将结果存储在变量length
中。最后,我们通过print()
函数输出字符串的长度。
二、处理用户输入的字符串
在实际应用中,我们常常需要处理用户输入的字符串,并计算其长度。以下是一个示例,展示了如何获取用户输入的字符串并计算其长度:
user_input = input("Please enter a string: ")
length = len(user_input)
print(f"The length of the entered string is: {length}")
在这个示例中,我们使用input()
函数从用户那里获取一个字符串,并将其存储在变量user_input
中。然后,我们使用len()
函数计算字符串的长度,并输出结果。
三、处理多行字符串
Python允许我们处理多行字符串,这些字符串可以通过三重引号('''
或"""
)来定义。以下是一个示例,展示了如何计算多行字符串的长度:
multi_line_string = """This is a string
that spans multiple
lines."""
length = len(multi_line_string)
print(f"The length of the multi-line string is: {length}")
在这个示例中,我们定义了一个多行字符串multi_line_string
,并使用len()
函数计算其长度。请注意,多行字符串中的换行符也会被计算在内。
四、忽略空白字符和换行符
有时候,我们可能需要计算字符串的长度,但忽略其中的空白字符和换行符。我们可以使用字符串的replace()
方法来去除这些字符。以下是一个示例:
my_string = "Hello, \nWorld!"
trimmed_string = my_string.replace(" ", "").replace("\n", "")
length = len(trimmed_string)
print(f"The length of the trimmed string is: {length}")
在这个示例中,我们首先定义了一个包含空白字符和换行符的字符串my_string
。然后,我们使用replace()
方法去除字符串中的空白字符和换行符,并将结果存储在变量trimmed_string
中。最后,我们使用len()
函数计算修剪后的字符串的长度。
五、处理特殊字符和Unicode字符
在某些情况下,字符串中可能包含特殊字符或Unicode字符。Python中的len()
函数能够正确处理这些字符。以下是一个示例,展示了如何计算包含特殊字符和Unicode字符的字符串的长度:
special_string = "Hello, 世界!"
length = len(special_string)
print(f"The length of the string with special characters is: {length}")
在这个示例中,我们定义了一个包含特殊字符和Unicode字符的字符串special_string
,并使用len()
函数计算其长度。请注意,Python中的len()
函数能够正确处理这些字符,并返回正确的字符串长度。
六、字符串长度与编码
在处理字符串时,我们需要注意字符串的编码问题。Python中的字符串默认使用Unicode编码,这意味着每个字符都被表示为一个Unicode码点。在某些情况下,我们可能需要处理不同编码方式的字符串,例如UTF-8或ASCII编码。以下是一个示例,展示了如何处理不同编码方式的字符串:
utf8_string = "Hello, 世界!".encode('utf-8')
ascii_string = "Hello, World!".encode('ascii')
length_utf8 = len(utf8_string)
length_ascii = len(ascii_string)
print(f"The length of the UTF-8 encoded string is: {length_utf8}")
print(f"The length of the ASCII encoded string is: {length_ascii}")
在这个示例中,我们首先将字符串"Hello, 世界!"
和"Hello, World!"
分别编码为UTF-8和ASCII。然后,我们使用len()
函数计算编码后的字符串长度。请注意,UTF-8编码的字符串长度可能与原始字符串长度不同,因为每个Unicode字符可能被表示为多个字节。
七、处理空字符串
在某些情况下,我们可能会遇到空字符串,即长度为0的字符串。以下是一个示例,展示了如何处理空字符串:
empty_string = ""
length = len(empty_string)
print(f"The length of the empty string is: {length}")
在这个示例中,我们定义了一个空字符串empty_string
,并使用len()
函数计算其长度。请注意,空字符串的长度为0。
八、字符串长度与列表和元组
除了字符串,Python中的len()
函数还可以用于计算列表和元组的长度。以下是一个示例,展示了如何计算列表和元组的长度:
my_list = [1, 2, 3, 4, 5]
my_tuple = (1, 2, 3, 4, 5)
length_list = len(my_list)
length_tuple = len(my_tuple)
print(f"The length of the list is: {length_list}")
print(f"The length of the tuple is: {length_tuple}")
在这个示例中,我们定义了一个列表my_list
和一个元组my_tuple
,并使用len()
函数分别计算它们的长度。请注意,len()
函数可以用于计算任意可迭代对象的长度。
九、字符串长度与嵌套结构
在某些情况下,我们可能需要处理嵌套结构的字符串。例如,一个字符串可能包含嵌套的列表或字典。以下是一个示例,展示了如何处理嵌套结构的字符串:
nested_string = "Hello, [1, 2, [3, 4], 5]!"
length = len(nested_string)
print(f"The length of the nested string is: {length}")
在这个示例中,我们定义了一个包含嵌套结构的字符串nested_string
,并使用len()
函数计算其长度。请注意,len()
函数只计算字符串的长度,而不会解析嵌套结构。
十、字符串长度与正则表达式
在某些情况下,我们可能需要使用正则表达式来匹配特定模式的字符串,并计算其长度。以下是一个示例,展示了如何使用正则表达式匹配字符串并计算其长度:
import re
my_string = "Hello, World! 123"
pattern = r"\d+"
match = re.search(pattern, my_string)
if match:
matched_string = match.group()
length = len(matched_string)
print(f"The length of the matched string is: {length}")
在这个示例中,我们首先定义了一个包含数字的字符串my_string
,然后使用正则表达式模式\d+
来匹配字符串中的数字。接着,我们使用re.search()
函数查找匹配的字符串,并通过match.group()
方法获取匹配的字符串。最后,我们使用len()
函数计算匹配字符串的长度。
十一、字符串长度与切片
在处理字符串时,我们可能需要对字符串进行切片,并计算切片后的字符串长度。以下是一个示例,展示了如何对字符串进行切片并计算其长度:
my_string = "Hello, World!"
sliced_string = my_string[7:]
length = len(sliced_string)
print(f"The length of the sliced string is: {length}")
在这个示例中,我们定义了一个字符串my_string
,并通过切片操作my_string[7:]
获取从索引7开始到字符串末尾的子字符串。然后,我们使用len()
函数计算切片后的字符串长度。
十二、字符串长度与拼接
在某些情况下,我们可能需要拼接多个字符串,并计算拼接后的字符串长度。以下是一个示例,展示了如何拼接字符串并计算其长度:
string1 = "Hello"
string2 = ", "
string3 = "World!"
concatenated_string = string1 + string2 + string3
length = len(concatenated_string)
print(f"The length of the concatenated string is: {length}")
在这个示例中,我们定义了三个字符串string1
、string2
和string3
,并通过字符串拼接操作将它们连接成一个新的字符串concatenated_string
。然后,我们使用len()
函数计算拼接后的字符串长度。
十三、字符串长度与格式化
在处理字符串时,我们可能需要使用字符串格式化来生成新的字符串,并计算其长度。以下是一个示例,展示了如何使用字符串格式化并计算其长度:
name = "Alice"
age = 30
formatted_string = f"My name is {name} and I am {age} years old."
length = len(formatted_string)
print(f"The length of the formatted string is: {length}")
在这个示例中,我们定义了两个变量name
和age
,并通过f字符串格式化生成一个新的字符串formatted_string
。然后,我们使用len()
函数计算格式化后的字符串长度。
十四、字符串长度与字符串方法
Python中的字符串对象提供了许多内置方法,这些方法可以对字符串进行各种操作。在某些情况下,我们可能需要在使用这些方法后计算字符串的长度。以下是一个示例,展示了如何使用字符串方法并计算其长度:
my_string = " Hello, World! "
stripped_string = my_string.strip()
length = len(stripped_string)
print(f"The length of the stripped string is: {length}")
在这个示例中,我们定义了一个包含前后空白字符的字符串my_string
,并使用strip()
方法去除字符串两端的空白字符。然后,我们使用len()
函数计算去除空白字符后的字符串长度。
十五、字符串长度与文件操作
在实际应用中,我们可能需要从文件中读取字符串,并计算其长度。以下是一个示例,展示了如何从文件中读取字符串并计算其长度:
with open("example.txt", "r") as file:
file_content = file.read()
length = len(file_content)
print(f"The length of the file content is: {length}")
在这个示例中,我们使用open()
函数以只读模式打开一个名为example.txt
的文件,并通过file.read()
方法读取文件内容。然后,我们使用len()
函数计算文件内容的长度。
十六、字符串长度与异常处理
在处理字符串长度时,我们可能会遇到各种异常情况,例如空字符串、无效输入等。我们可以使用异常处理机制来处理这些情况。以下是一个示例,展示了如何使用异常处理来处理字符串长度计算中的异常情况:
try:
user_input = input("Please enter a string: ")
if not user_input:
raise ValueError("The input string cannot be empty.")
length = len(user_input)
print(f"The length of the entered string is: {length}")
except ValueError as e:
print(f"Error: {e}")
在这个示例中,我们使用try
块来捕获用户输入,并检查输入字符串是否为空。如果输入字符串为空,我们通过raise
语句抛出一个ValueError
异常。然后,我们使用except
块来捕获并处理该异常,并输出错误消息。
通过以上示例,我们可以看到,Python中的len()
函数在计算字符串长度时非常强大且灵活。无论是处理基本字符串、用户输入、特殊字符、多行字符串还是嵌套结构,len()
函数都能够准确地计算字符串的长度。此外,我们还可以结合正则表达式、字符串方法、文件操作和异常处理等技术,进一步增强字符串长度计算的功能和灵活性。希望这些示例能够帮助您更好地理解和应用Python中的字符串长度计算。
相关问答FAQs:
如何在Python中获取用户输入的字符串长度?
在Python中,您可以使用内置的input()
函数来获取用户输入的字符串。获取字符串后,可以使用len()
函数来计算该字符串的长度。示例代码如下:
user_input = input("请输入一个字符串:")
length = len(user_input)
print("字符串的长度是:", length)
通过这种方式,您能够轻松获取任何字符串的长度。
Python支持哪些字符串操作以获取长度信息?
除了使用len()
函数,Python还支持多种字符串操作,如字符串切片、遍历等。您可以使用字符串的特性来处理和分析字符串。例如,您可以通过遍历字符串中的每个字符来手动计算长度,尽管这在实际应用中不太常见。
是否有方法可以处理空字符串或特殊字符的长度?
在Python中,len()
函数能够正确处理空字符串和特殊字符。对于空字符串,返回的长度为0;而对于包含特殊字符的字符串,它会将每个字符都计算在内。无论字符串中包含何种字符,len()
函数都会给出准确的长度信息。例如:
special_string = "Hello, 世界! 😊"
print("特殊字符串的长度是:", len(special_string))
此代码将输出特殊字符串的真实字符数。
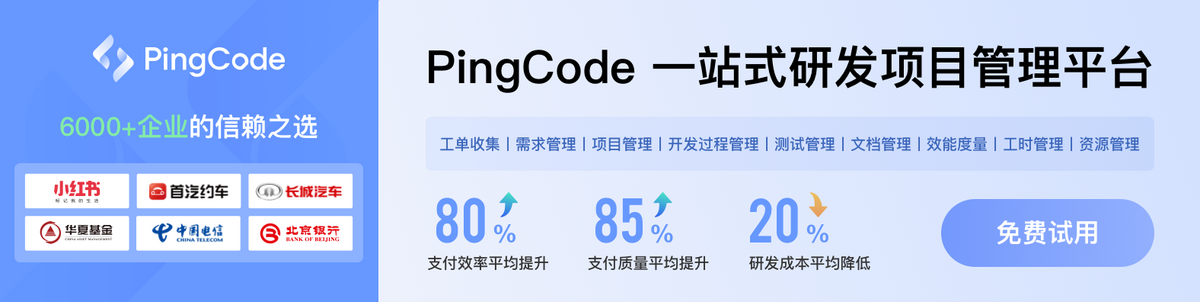