在Python中查询元素的偏移量可以通过多种方法实现,常见的有:使用BeautifulSoup库解析HTML文档、使用Selenium库进行网页自动化操作、使用Pygame库处理游戏开发中的元素偏移量。本文将详细介绍这些方法,并探讨它们在不同场景中的应用。
一、使用BeautifulSoup库查询HTML文档中的元素偏移量
1. BeautifulSoup简介
BeautifulSoup是一个可以从HTML或XML文件中提取数据的Python库。它创建一个解析树并将获取的数据转换为Python对象,方便操作和查询。
2. 安装BeautifulSoup
首先需要安装BeautifulSoup库,可以使用pip命令进行安装:
pip install beautifulsoup4
3. 使用BeautifulSoup查询元素偏移量
使用BeautifulSoup解析HTML文档并查询元素的偏移量,具体代码如下:
from bs4 import BeautifulSoup
html_doc = """
<html>
<head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
查找所有链接
links = soup.find_all('a')
for link in links:
print(link.get('href'), link.text)
代码解析:
- 使用BeautifulSoup解析HTML文档。
- 使用
soup.find_all('a')
查找所有的<a>
标签。 - 遍历所有的
<a>
标签,输出其href
属性和文本内容。
4. 获取元素的偏移量
虽然BeautifulSoup可以方便地解析和查询HTML文档中的元素,但它并不能直接获取元素在页面中的偏移量。要获取元素的偏移量,可以结合JavaScript和Python。
二、使用Selenium库进行网页自动化操作
1. Selenium简介
Selenium是一个用于Web应用程序测试的工具。它可以通过Python脚本控制浏览器的行为,模拟用户操作,并获取网页中的数据。
2. 安装Selenium
可以使用pip命令安装Selenium库:
pip install selenium
3. 使用Selenium查询元素偏移量
使用Selenium库可以获取网页中元素的偏移量,具体代码如下:
from selenium import webdriver
设置Chrome驱动路径
driver_path = 'path/to/chromedriver'
初始化浏览器
driver = webdriver.Chrome(driver_path)
打开网页
driver.get('http://example.com')
查找元素
element = driver.find_element_by_id('link1')
获取元素偏移量
offset_top = element.location['y']
offset_left = element.location['x']
print(f'Element offset - Top: {offset_top}, Left: {offset_left}')
关闭浏览器
driver.quit()
代码解析:
- 使用Selenium控制浏览器打开指定网页。
- 查找网页中的元素。
- 使用
element.location
属性获取元素的偏移量。 - 输出元素的偏移量。
4. 使用Selenium处理动态网页
对于动态网页,Selenium更具优势。它可以处理JavaScript生成的内容,并模拟用户交互。以下是一个处理动态网页的示例:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
设置Chrome驱动路径
driver_path = 'path/to/chromedriver'
初始化浏览器
driver = webdriver.Chrome(driver_path)
打开网页
driver.get('http://example.com')
try:
# 等待元素加载
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, 'link1'))
)
# 获取元素偏移量
offset_top = element.location['y']
offset_left = element.location['x']
print(f'Element offset - Top: {offset_top}, Left: {offset_left}')
finally:
# 关闭浏览器
driver.quit()
代码解析:
- 使用
WebDriverWait
等待元素加载。 - 使用
EC.presence_of_element_located
判断元素是否存在。 - 获取并输出元素的偏移量。
三、使用Pygame库处理游戏开发中的元素偏移量
1. Pygame简介
Pygame是一个用于开发2D游戏的Python库。它提供了处理游戏元素、事件、图形和声音的功能。
2. 安装Pygame
可以使用pip命令安装Pygame库:
pip install pygame
3. 使用Pygame查询元素偏移量
使用Pygame库可以处理游戏元素的偏移量,具体代码如下:
import pygame
初始化Pygame
pygame.init()
设置窗口大小
screen = pygame.display.set_mode((800, 600))
定义颜色
white = (255, 255, 255)
red = (255, 0, 0)
加载图像
image = pygame.image.load('path/to/image.png')
image_rect = image.get_rect()
设置图像位置
image_rect.topleft = (100, 100)
运行游戏循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 清屏
screen.fill(white)
# 绘制图像
screen.blit(image, image_rect)
# 获取图像偏移量
offset_top = image_rect.top
offset_left = image_rect.left
print(f'Image offset - Top: {offset_top}, Left: {offset_left}')
# 更新显示
pygame.display.flip()
退出Pygame
pygame.quit()
代码解析:
- 初始化Pygame并设置窗口大小。
- 加载图像并设置其位置。
- 在游戏循环中绘制图像并获取其偏移量。
- 输出图像的偏移量。
4. 处理游戏元素的偏移量
在游戏开发中,处理游戏元素的偏移量非常重要。以下是一个处理游戏元素偏移量的示例:
import pygame
初始化Pygame
pygame.init()
设置窗口大小
screen = pygame.display.set_mode((800, 600))
定义颜色
white = (255, 255, 255)
red = (255, 0, 0)
加载图像
image = pygame.image.load('path/to/image.png')
image_rect = image.get_rect()
设置图像位置
image_rect.topleft = (100, 100)
定义速度
speed = [2, 2]
运行游戏循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 移动图像
image_rect = image_rect.move(speed)
# 检查边界
if image_rect.left < 0 or image_rect.right > 800:
speed[0] = -speed[0]
if image_rect.top < 0 or image_rect.bottom > 600:
speed[1] = -speed[1]
# 清屏
screen.fill(white)
# 绘制图像
screen.blit(image, image_rect)
# 获取图像偏移量
offset_top = image_rect.top
offset_left = image_rect.left
print(f'Image offset - Top: {offset_top}, Left: {offset_left}')
# 更新显示
pygame.display.flip()
退出Pygame
pygame.quit()
代码解析:
- 在游戏循环中移动图像,并检查边界碰撞。
- 获取并输出图像的偏移量。
四、总结
在Python中查询元素的偏移量可以通过多种方法实现,常见的有:使用BeautifulSoup库解析HTML文档、使用Selenium库进行网页自动化操作、使用Pygame库处理游戏开发中的元素偏移量。BeautifulSoup适用于解析和查询静态HTML文档中的元素,Selenium适用于处理动态网页和模拟用户交互,Pygame适用于处理游戏开发中的元素偏移量。根据不同的应用场景选择合适的方法,可以有效地解决元素偏移量查询的问题。
相关问答FAQs:
如何在Python中获取列表元素的索引?
在Python中,可以使用list.index()
方法来获取元素在列表中的索引。例如,如果你有一个列表my_list = [10, 20, 30, 40]
,并想要找到元素30
的索引,可以使用my_list.index(30)
,这将返回2
,因为30
在列表中的位置是第3个(索引从0开始)。
可以通过哪些方式获取字典中元素的偏移量?
字典的元素没有偏移量的概念,因为字典是无序的。然而,如果你想要获取特定键的索引,可以将字典的键转换为列表,然后使用list.index()
方法。比如,对于字典my_dict = {'a': 1, 'b': 2, 'c': 3}
,可以使用list(my_dict.keys()).index('b')
来获取键'b'
的索引,这将返回1
。
在Python中如何处理多维数组元素的偏移量?
对于多维数组,尤其是使用NumPy库时,可以使用numpy.where()
函数来查找特定元素的位置。假设你有一个二维数组array = np.array([[1, 2], [3, 4]])
,你可以使用np.where(array == 3)
来获取元素3
的偏移量,它将返回一个包含行和列索引的元组,例如(array([1]), array([0]))
,表示3
在第二行第一列。
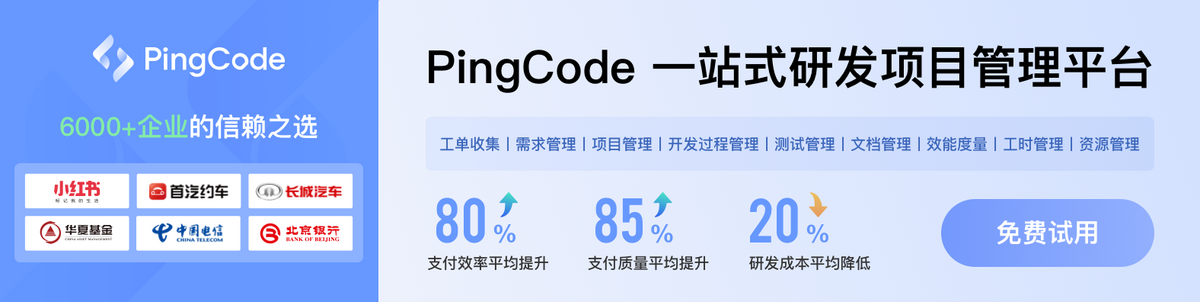