Python将文字转换为字符串的方法包括使用引号、str()函数、repr()函数等方式。在Python中,字符串可以通过单引号或双引号来定义。
要详细了解其中的一种方法,我们可以深入探讨使用str()函数将其他数据类型转换为字符串的过程。str()函数是Python内置的一个函数,它能够将对象转化为适合人类阅读的形式。对于非字符串数据类型(如整数、浮点数、列表等),使用str()函数可以将其转换为字符串格式,方便进行后续的字符串操作。
下面我们将详细探讨如何在Python中将文字转换为字符串。
一、使用引号定义字符串
在Python中,最简单的方法是使用单引号或双引号将文字括起来,直接定义成字符串。具体示例如下:
# 使用单引号定义字符串
string1 = 'Hello, World!'
使用双引号定义字符串
string2 = "Hello, World!"
在上述代码中,我们分别使用单引号和双引号将文字 "Hello, World!" 定义为字符串。这种方法非常直观,并且适用于大多数场景。
二、使用str()函数将其他类型转换为字符串
str()函数是Python内置的一个函数,用于将其他数据类型转换为字符串。以下是使用str()函数将不同数据类型转换为字符串的示例:
# 将整数转换为字符串
num = 123
str_num = str(num)
print(str_num) # 输出: '123'
将浮点数转换为字符串
float_num = 123.456
str_float_num = str(float_num)
print(str_float_num) # 输出: '123.456'
将列表转换为字符串
my_list = [1, 2, 3]
str_list = str(my_list)
print(str_list) # 输出: '[1, 2, 3]'
将字典转换为字符串
my_dict = {'a': 1, 'b': 2}
str_dict = str(my_dict)
print(str_dict) # 输出: "{'a': 1, 'b': 2}"
通过上述示例可以看到,str()函数能够将整数、浮点数、列表、字典等数据类型转换为字符串,方便进行后续的字符串操作。
三、使用repr()函数获取更精确的字符串表示
repr()函数与str()函数类似,也可以将其他数据类型转换为字符串。不同的是,repr()函数返回的是一个更精确的字符串表示,通常用于调试。以下是使用repr()函数的示例:
# 使用repr()函数将整数转换为字符串
num = 123
repr_num = repr(num)
print(repr_num) # 输出: '123'
使用repr()函数将浮点数转换为字符串
float_num = 123.456
repr_float_num = repr(float_num)
print(repr_float_num) # 输出: '123.456'
使用repr()函数将列表转换为字符串
my_list = [1, 2, 3]
repr_list = repr(my_list)
print(repr_list) # 输出: '[1, 2, 3]'
使用repr()函数将字典转换为字符串
my_dict = {'a': 1, 'b': 2}
repr_dict = repr(my_dict)
print(repr_dict) # 输出: "{'a': 1, 'b': 2}"
通过上述示例可以看到,repr()函数与str()函数的输出非常相似,但repr()函数通常用于返回更精确的字符串表示。
四、字符串格式化
Python还提供了一些字符串格式化的方法,可以将其他数据类型转换为字符串并插入到字符串中。主要有以下几种格式化方式:
- 百分号格式化(旧式格式化)
# 使用百分号格式化将整数插入到字符串中
num = 123
formatted_string = "The number is %d" % num
print(formatted_string) # 输出: 'The number is 123'
使用百分号格式化将浮点数插入到字符串中
float_num = 123.456
formatted_string = "The float number is %.2f" % float_num
print(formatted_string) # 输出: 'The float number is 123.46'
- str.format()方法
# 使用str.format()方法将整数插入到字符串中
num = 123
formatted_string = "The number is {}".format(num)
print(formatted_string) # 输出: 'The number is 123'
使用str.format()方法将浮点数插入到字符串中
float_num = 123.456
formatted_string = "The float number is {:.2f}".format(float_num)
print(formatted_string) # 输出: 'The float number is 123.46'
- f-字符串(格式化字符串字面量)
# 使用f-字符串将整数插入到字符串中
num = 123
formatted_string = f"The number is {num}"
print(formatted_string) # 输出: 'The number is 123'
使用f-字符串将浮点数插入到字符串中
float_num = 123.456
formatted_string = f"The float number is {float_num:.2f}"
print(formatted_string) # 输出: 'The float number is 123.46'
通过上述示例可以看到,百分号格式化、str.format()方法和f-字符串都可以将其他数据类型转换为字符串并插入到字符串中。这些方法提供了灵活的字符串格式化方式,适用于不同的场景。
五、总结
在Python中,将文字转换为字符串的方法包括使用引号、str()函数、repr()函数、字符串格式化等方式。使用引号可以直接定义字符串,str()函数可以将其他数据类型转换为字符串,repr()函数返回更精确的字符串表示,字符串格式化方法可以将其他数据类型插入到字符串中。
根据具体需求选择合适的方法,可以灵活地处理字符串操作。希望本文对你理解Python中将文字转换为字符串的方法有所帮助。
相关问答FAQs:
如何在Python中将文本转换为字符串?
在Python中,文本通常以字符串的形式表示。要将普通文本转换为字符串,你可以使用简单的赋值方法。例如,可以直接将文本用引号包围起来:my_string = "这是一个字符串"
。这样,你就创建了一个字符串类型的变量。
在Python中如何处理包含空格和特殊字符的文本?
处理包含空格或特殊字符的文本时,确保使用引号将整个文本包围起来。例如,对于包含空格的文本,可以写成:my_string = "这是一个包含空格的字符串"
。如果文本中含有引号,可以使用转义字符(\)来处理,例如:my_string = "这是一个包含\"引号\"的字符串"
。
Python中如何将字符串转换为其他数据类型?
如果需要将字符串转换为其他数据类型,可以使用相应的内置函数。例如,使用int()
函数可以将数字字符串转换为整数,float()
函数可以将数字字符串转换为浮点数。示例代码如下:
number_string = "123"
number_integer = int(number_string) # 转换为整数
number_float = float(number_string) # 转换为浮点数
这样,你可以根据需要将字符串转换为不同的数据类型。
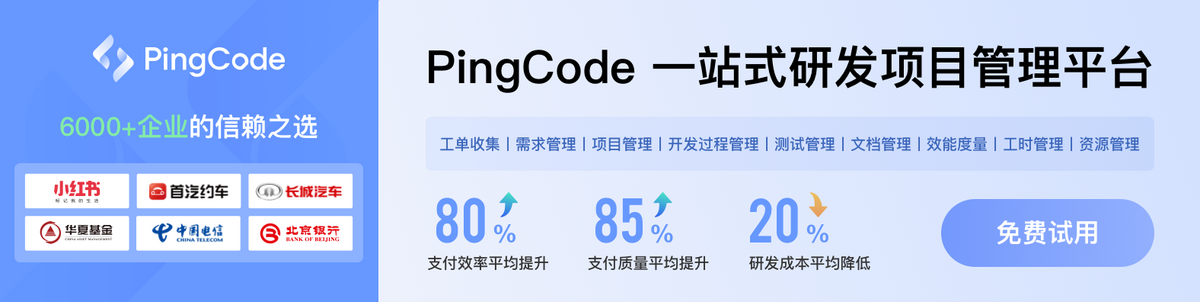