Python中读取多个文件是否存在的方法包括os.path模块、glob模块以及pathlib模块等。其中,os.path模块常用来检查文件的存在性、glob模块适合用于匹配特定模式的文件、pathlib模块则是现代Python开发中推荐的方式。在这些方法中,os.path模块适合基础检查,glob模块便于批量文件处理,而pathlib模块提供了更直观、更易读的代码方式。下面将详细展开介绍其中一种方法,即使用os.path模块进行文件存在性检查。
一、使用os.path模块读取多个文件是否存在
1、基础检查方法
os.path模块提供了几个重要的函数来检查文件和目录的存在性。常用的函数有os.path.exists()
和os.path.isfile()
。
示例如下:
import os
files = ['file1.txt', 'file2.txt', 'file3.txt']
for file in files:
if os.path.exists(file):
print(f"{file} exists")
else:
print(f"{file} does not exist")
在上述代码中,os.path.exists()
函数用于检查文件或目录是否存在。若存在,则返回True,否则返回False。
2、检查特定目录下的文件
如果需要检查特定目录下的文件存在性,可以将目录路径和文件名拼接起来进行检查。
示例如下:
import os
directory = '/path/to/directory'
files = ['file1.txt', 'file2.txt', 'file3.txt']
for file in files:
file_path = os.path.join(directory, file)
if os.path.exists(file_path):
print(f"{file} exists in {directory}")
else:
print(f"{file} does not exist in {directory}")
在上述代码中,os.path.join()
函数用于拼接目录路径和文件名,生成完整的文件路径。
二、使用glob模块读取多个文件是否存在
1、匹配特定模式的文件
glob模块可以用来查找符合特定模式的文件路径名。常用的函数有glob.glob()
。
示例如下:
import glob
pattern = '/path/to/directory/*.txt'
files = glob.glob(pattern)
for file in files:
print(f"{file} exists")
在上述代码中,glob.glob()
函数用于查找所有匹配给定模式的文件路径名,并返回一个列表。
2、递归匹配文件
glob模块在Python 3.5及更高版本中提供了递归匹配文件的功能。可以使用模式和
recursive=True
参数。
示例如下:
import glob
pattern = '/path/to/directory//*.txt'
files = glob.glob(pattern, recursive=True)
for file in files:
print(f"{file} exists")
在上述代码中,模式用于匹配任意层级的目录,
recursive=True
参数启用递归匹配。
三、使用pathlib模块读取多个文件是否存在
1、基础检查方法
pathlib模块提供了面向对象的路径操作方式。常用的类和方法有Path
类和exists()
方法。
示例如下:
from pathlib import Path
files = ['file1.txt', 'file2.txt', 'file3.txt']
for file in files:
file_path = Path(file)
if file_path.exists():
print(f"{file} exists")
else:
print(f"{file} does not exist")
在上述代码中,Path
类用于表示文件路径,exists()
方法用于检查文件或目录是否存在。
2、检查特定目录下的文件
如果需要检查特定目录下的文件存在性,可以将目录路径和文件名拼接起来进行检查。
示例如下:
from pathlib import Path
directory = Path('/path/to/directory')
files = ['file1.txt', 'file2.txt', 'file3.txt']
for file in files:
file_path = directory / file
if file_path.exists():
print(f"{file} exists in {directory}")
else:
print(f"{file} does not exist in {directory}")
在上述代码中,使用/
运算符来拼接目录路径和文件名,生成完整的文件路径。
四、总结
在Python中,读取多个文件是否存在的方法包括os.path模块、glob模块以及pathlib模块。其中,os.path模块适合基础检查,glob模块便于批量文件处理,pathlib模块提供了更直观、更易读的代码方式。选择合适的方法可以提高代码的可读性和维护性。
通过os.path模块,我们可以方便地进行文件和目录的存在性检查;通过glob模块,我们可以高效地匹配特定模式的文件;通过pathlib模块,我们可以采用面向对象的方式进行路径操作,从而使代码更加简洁和易读。根据实际需求,选择合适的方法进行文件存在性检查,可以显著提高代码的效率和可靠性。
相关问答FAQs:
如何在Python中检查多个文件是否存在?
在Python中,可以使用os.path
模块中的exists()
函数来检查文件是否存在。首先,您需要导入os
模块,然后为每个文件路径调用os.path.exists()
。以下是一个示例代码:
import os
file_paths = ['file1.txt', 'file2.txt', 'file3.txt']
for file in file_paths:
if os.path.exists(file):
print(f"{file} 存在")
else:
print(f"{file} 不存在")
这种方法非常简便,适合快速验证多个文件的存在性。
使用Python读取文件时,如何处理不存在的文件?
在读取文件时,如果文件不存在,通常会引发FileNotFoundError
异常。可以通过使用try-except
语句来优雅地处理这种情况。以下是一个示例:
file_paths = ['file1.txt', 'file2.txt', 'file3.txt']
for file in file_paths:
try:
with open(file, 'r') as f:
content = f.read()
print(f"{file} 内容:\n{content}")
except FileNotFoundError:
print(f"{file} 不存在,无法读取。")
这种方法确保在尝试读取文件时不会导致程序崩溃。
在Python中,如何提高文件存在性检查的效率?
如果需要检查的文件数量较多,可以考虑使用多线程或异步编程来提高效率。借助concurrent.futures
模块,可以并行地检查多个文件的存在性。以下是一个简单的示例:
import os
from concurrent.futures import ThreadPoolExecutor
def check_file_exists(file):
return os.path.exists(file)
file_paths = ['file1.txt', 'file2.txt', 'file3.txt']
with ThreadPoolExecutor() as executor:
results = executor.map(check_file_exists, file_paths)
for file, exists in zip(file_paths, results):
status = "存在" if exists else "不存在"
print(f"{file} {status}")
这种方法能够显著缩短检查多个文件的时间。
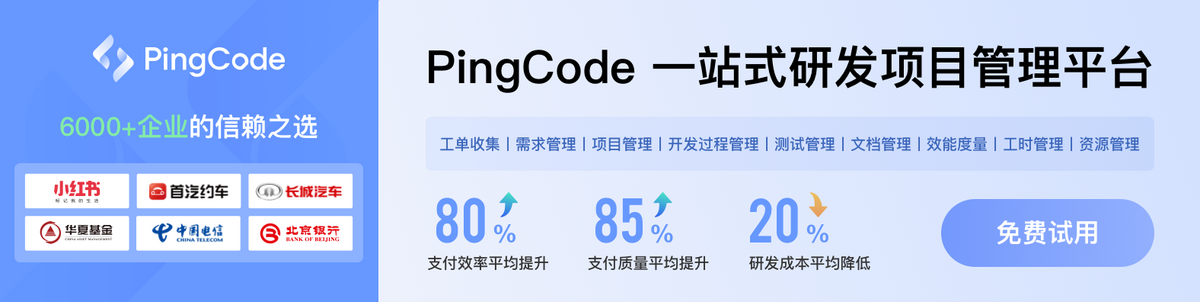