Python批处理是通过脚本编写、使用循环和条件语句、调用系统命令、处理文件和目录、以及使用多线程和多进程技术来实现的。 其中,脚本编写是最核心的部分,它决定了批处理任务的逻辑和流程。接下来,我们将详细介绍Python批处理的各个方面。
一、脚本编写
脚本编写是Python批处理的核心。通过编写脚本,可以实现自动化处理任务,避免人工操作的繁琐和错误。Python脚本的编写包括编写函数、定义变量、使用模块和库等。
1、定义变量和函数
在Python脚本中,变量用于存储数据,函数用于封装重复使用的代码块。通过定义变量和函数,可以提高代码的可读性和可维护性。
# 定义变量
input_file = 'input.txt'
output_file = 'output.txt'
定义函数
def process_file(input_file, output_file):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
processed_line = line.strip().upper()
outfile.write(processed_line + '\n')
2、使用模块和库
Python提供了丰富的标准库和第三方库,可以帮助我们更高效地完成批处理任务。例如,使用os
库处理文件和目录,使用subprocess
库调用系统命令,使用csv
库处理CSV文件等。
import os
import subprocess
import csv
使用os库处理文件和目录
if not os.path.exists('output'):
os.makedirs('output')
使用subprocess库调用系统命令
subprocess.run(['ls', '-l'])
使用csv库处理CSV文件
with open('data.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
二、使用循环和条件语句
循环和条件语句是编程的基础,通过使用它们可以实现复杂的逻辑控制。在批处理任务中,经常需要遍历文件列表、处理多行数据、根据条件执行不同的操作等。
1、遍历文件列表
批处理任务中经常需要处理多个文件,可以使用循环遍历文件列表,逐个处理文件。
import os
获取文件列表
file_list = os.listdir('input')
遍历文件列表
for file_name in file_list:
input_file = os.path.join('input', file_name)
output_file = os.path.join('output', file_name)
process_file(input_file, output_file)
2、处理多行数据
在处理文本文件时,通常需要逐行读取和处理数据,可以使用循环遍历文件的每一行。
def process_file(input_file, output_file):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
processed_line = line.strip().upper()
outfile.write(processed_line + '\n')
三、调用系统命令
在批处理任务中,有时需要调用系统命令来完成某些操作,例如压缩文件、执行外部程序等。Python的subprocess
库提供了强大的功能,可以方便地调用系统命令。
1、简单调用系统命令
可以使用subprocess.run
函数调用简单的系统命令,并获取命令的输出和返回值。
import subprocess
调用系统命令
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
获取命令输出
print(result.stdout)
获取命令返回值
print(result.returncode)
2、处理复杂的系统命令
对于复杂的系统命令,可以使用subprocess.Popen
类,提供更灵活的控制和处理。
import subprocess
启动外部程序
process = subprocess.Popen(['my_program', 'arg1', 'arg2'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
获取程序输出
stdout, stderr = process.communicate()
获取返回值
returncode = process.returncode
处理输出和返回值
if returncode == 0:
print('Program executed successfully:')
print(stdout)
else:
print('Program failed with error:')
print(stderr)
四、处理文件和目录
文件和目录操作是批处理任务的重要组成部分。Python的os
和shutil
库提供了丰富的文件和目录操作功能,可以方便地进行文件复制、移动、删除等操作。
1、文件操作
可以使用os
库进行文件的基本操作,例如检查文件是否存在、删除文件等。
import os
检查文件是否存在
if os.path.exists('input.txt'):
print('File exists')
删除文件
os.remove('input.txt')
2、目录操作
可以使用os
和shutil
库进行目录的基本操作,例如创建目录、删除目录、复制目录等。
import os
import shutil
创建目录
if not os.path.exists('output'):
os.makedirs('output')
删除目录
shutil.rmtree('output')
复制目录
shutil.copytree('input', 'output')
五、使用多线程和多进程
在批处理任务中,有时需要处理大量数据或执行耗时的操作,可以使用多线程和多进程技术提高处理效率。Python的threading
和multiprocessing
库提供了多线程和多进程的支持。
1、多线程处理
使用threading
库可以方便地创建和管理线程,实现多线程处理。
import threading
定义线程函数
def process_file_thread(input_file, output_file):
process_file(input_file, output_file)
创建线程
threads = []
for file_name in file_list:
input_file = os.path.join('input', file_name)
output_file = os.path.join('output', file_name)
thread = threading.Thread(target=process_file_thread, args=(input_file, output_file))
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
2、多进程处理
使用multiprocessing
库可以方便地创建和管理进程,实现多进程处理。
import multiprocessing
定义进程函数
def process_file_process(input_file, output_file):
process_file(input_file, output_file)
创建进程池
with multiprocessing.Pool() as pool:
for file_name in file_list:
input_file = os.path.join('input', file_name)
output_file = os.path.join('output', file_name)
pool.apply_async(process_file_process, args=(input_file, output_file))
# 等待所有进程完成
pool.close()
pool.join()
六、错误处理和日志记录
在批处理任务中,错误处理和日志记录是非常重要的。通过捕获异常和记录日志,可以提高程序的健壮性和可维护性。
1、错误处理
可以使用try...except
语句捕获异常,并进行相应的处理。
def process_file(input_file, output_file):
try:
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
processed_line = line.strip().upper()
outfile.write(processed_line + '\n')
except Exception as e:
print(f'Error processing file {input_file}: {e}')
2、日志记录
可以使用logging
库记录日志,方便调试和排查问题。
import logging
配置日志记录
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def process_file(input_file, output_file):
try:
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
processed_line = line.strip().upper()
outfile.write(processed_line + '\n')
logging.info(f'Successfully processed file {input_file}')
except Exception as e:
logging.error(f'Error processing file {input_file}: {e}')
七、总结
Python批处理通过脚本编写、使用循环和条件语句、调用系统命令、处理文件和目录、以及使用多线程和多进程技术来实现。通过以上方法,可以高效地完成各种批处理任务,提高工作效率和程序的健壮性。在实际应用中,可以根据具体需求选择合适的方法和技术,灵活组合使用,以达到最佳效果。
相关问答FAQs:
Python批处理的基本概念是什么?
Python批处理是一种通过Python脚本自动执行多个任务的方式。与手动执行每个任务相比,批处理可以显著提高效率,特别是在处理大量数据或重复性操作时。通过编写脚本,用户可以一次性处理文件、数据或其他资源,而无需单独执行每个步骤。
如何在Python中实现批处理?
要实现批处理,用户需要编写一个Python脚本,其中包括所需的功能和操作。这通常涉及到使用循环、条件语句和文件处理模块。常见的库如os和shutil可以帮助用户处理文件和目录。例如,用户可以编写一个脚本来遍历特定文件夹中的所有文件,并对每个文件执行特定操作,比如重命名或格式转换。
有哪些常见的Python批处理应用场景?
Python批处理广泛应用于数据分析、文件管理和自动化测试等领域。在数据分析中,用户可以批量处理CSV文件,进行数据清洗和转换。在文件管理方面,批处理可以用于整理和分类大量的文档或图片。此外,在自动化测试中,批处理可以帮助运行一系列测试用例并收集结果,从而提高测试效率。
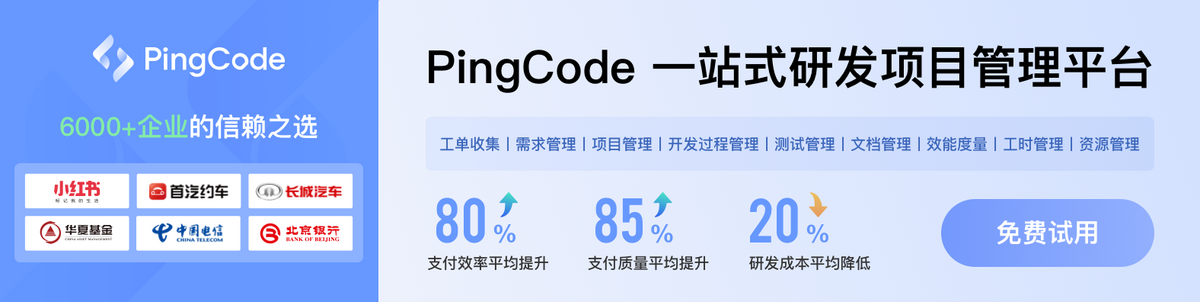