Python使用关键字查询信息的方法:使用适当的数据结构、使用字符串操作、利用正则表达式。 其中,利用正则表达式 是一种强大且灵活的方式,可以让你在文本中进行复杂的模式匹配和提取信息。正则表达式是一种用于描述文本模式的强大工具,它提供了一种简洁而灵活的方法来处理字符串。
正则表达式(Regular Expressions,简称re)在Python中由re
模块支持。通过定义一个模式,你可以在文本中搜索、替换和提取信息。例如,如果你想从一段文本中提取所有以“关键字”开头的单词,可以使用正则表达式来实现。正则表达式不仅适用于简单的查找操作,还可以处理复杂的文本处理任务,如验证输入格式、解析日志文件等。
下面我们将详细介绍在Python中使用关键字查询信息的各种方法。
一、使用适当的数据结构
在Python中,有多种数据结构可以用来存储和查询信息。选择合适的数据结构可以极大地提高查询效率和代码的可读性。
1、列表
列表是一种常见的数据结构,适用于存储和查询顺序数据。你可以使用in
关键字来检查一个元素是否在列表中。
data = ["apple", "banana", "cherry"]
keyword = "banana"
if keyword in data:
print(f"{keyword} is in the list")
else:
print(f"{keyword} is not in the list")
2、字典
字典是一种键值对的数据结构,适用于快速查找。你可以使用字典来存储信息,并使用关键字来查询。
data = {"apple": 1, "banana": 2, "cherry": 3}
keyword = "banana"
if keyword in data:
print(f"{keyword} is in the dictionary with value {data[keyword]}")
else:
print(f"{keyword} is not in the dictionary")
3、集合
集合是一种无序且唯一的数据结构,适用于需要快速查找和去重的场景。
data = {"apple", "banana", "cherry"}
keyword = "banana"
if keyword in data:
print(f"{keyword} is in the set")
else:
print(f"{keyword} is not in the set")
二、使用字符串操作
字符串操作是最基本的文本处理方法,适用于简单的查询和替换任务。
1、查找子字符串
你可以使用find
方法来查找子字符串的位置,或者使用in
关键字来检查子字符串是否存在。
text = "The quick brown fox jumps over the lazy dog"
keyword = "fox"
if keyword in text:
print(f"{keyword} is in the text")
else:
print(f"{keyword} is not in the text")
position = text.find(keyword)
if position != -1:
print(f"{keyword} found at position {position}")
else:
print(f"{keyword} not found")
2、替换子字符串
你可以使用replace
方法来替换子字符串。
text = "The quick brown fox jumps over the lazy dog"
keyword = "fox"
replacement = "cat"
new_text = text.replace(keyword, replacement)
print(new_text)
三、利用正则表达式
正则表达式提供了一种灵活而强大的方式来查找和处理文本中的模式。
1、基本用法
在Python中,你可以使用re
模块来处理正则表达式。常用的方法包括re.search
、re.match
、re.findall
和re.sub
。
import re
text = "The quick brown fox jumps over the lazy dog"
keyword = "fox"
查找关键字
match = re.search(keyword, text)
if match:
print(f"{keyword} found at position {match.start()}")
else:
print(f"{keyword} not found")
查找所有匹配项
text = "The quick brown fox jumps over the lazy dog. The fox is clever."
matches = re.findall(keyword, text)
print(f"Found {len(matches)} occurrences of '{keyword}'")
2、复杂模式
正则表达式可以用来处理复杂的文本模式,比如匹配电子邮件地址、电话号码等。
text = "Contact us at support@example.com or sales@example.com"
email_pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
emails = re.findall(email_pattern, text)
print(f"Found emails: {emails}")
3、分组和捕获
正则表达式支持分组和捕获,可以提取匹配结果中的特定部分。
text = "John Doe, 123-456-7890; Jane Smith, 987-654-3210"
phone_pattern = r'(\d{3})-(\d{3})-(\d{4})'
matches = re.findall(phone_pattern, text)
for match in matches:
print(f"Area code: {match[0]}, Exchange: {match[1]}, Subscriber number: {match[2]}")
4、替换匹配项
你可以使用re.sub
方法来替换匹配项。
text = "The quick brown fox jumps over the lazy dog"
keyword = "fox"
replacement = "cat"
new_text = re.sub(keyword, replacement, text)
print(new_text)
四、使用数据库查询
在处理大量数据时,使用数据库进行查询是一种高效的方法。你可以使用SQL语句来查询和处理数据。
1、SQLite示例
SQLite是一个轻量级的嵌入式数据库,适用于小型应用程序。
import sqlite3
创建数据库连接
conn = sqlite3.connect(':memory:')
cursor = conn.cursor()
创建表
cursor.execute('''CREATE TABLE fruits (name TEXT, quantity INTEGER)''')
插入数据
cursor.execute("INSERT INTO fruits VALUES ('apple', 10)")
cursor.execute("INSERT INTO fruits VALUES ('banana', 20)")
cursor.execute("INSERT INTO fruits VALUES ('cherry', 30)")
查询数据
keyword = 'banana'
cursor.execute("SELECT * FROM fruits WHERE name=?", (keyword,))
result = cursor.fetchone()
if result:
print(f"Found {result[1]} {keyword}s")
else:
print(f"{keyword} not found")
关闭数据库连接
conn.close()
2、使用SQLAlchemy
SQLAlchemy是一个功能强大的ORM(对象关系映射)库,适用于更复杂的数据库操作。
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
创建数据库引擎
engine = create_engine('sqlite:///:memory:')
Base = declarative_base()
定义模型
class Fruit(Base):
__tablename__ = 'fruits'
id = Column(Integer, primary_key=True)
name = Column(String)
quantity = Column(Integer)
创建表
Base.metadata.create_all(engine)
创建会话
Session = sessionmaker(bind=engine)
session = Session()
插入数据
session.add_all([Fruit(name='apple', quantity=10), Fruit(name='banana', quantity=20), Fruit(name='cherry', quantity=30)])
session.commit()
查询数据
keyword = 'banana'
result = session.query(Fruit).filter_by(name=keyword).first()
if result:
print(f"Found {result.quantity} {keyword}s")
else:
print(f"{keyword} not found")
关闭会话
session.close()
五、使用搜索引擎和API
有时候,我们需要查询的信息存在于网络上,而不是在本地数据中。此时,可以使用搜索引擎和API来获取信息。
1、使用Google搜索
你可以使用Python库(如googlesearch-python
)来执行Google搜索,并获取搜索结果。
from googlesearch import search
keyword = "Python programming"
num_results = 5
for result in search(keyword, num_results=num_results):
print(result)
2、使用API
许多网站和服务提供API来查询信息。例如,你可以使用GitHub API来查询代码仓库。
import requests
username = "octocat"
url = f"https://api.github.com/users/{username}/repos"
response = requests.get(url)
repos = response.json()
for repo in repos:
print(repo['name'])
六、使用自定义索引和全文搜索
在处理大量文本数据时,使用自定义索引和全文搜索引擎(如Elasticsearch)可以显著提高查询效率。
1、使用Whoosh
Whoosh是一个轻量级的全文搜索库,适用于构建自定义搜索引擎。
from whoosh.index import create_in
from whoosh.fields import Schema, TEXT
from whoosh.qparser import QueryParser
定义索引架构
schema = Schema(title=TEXT(stored=True), content=TEXT)
创建索引
index_dir = "indexdir"
import os
if not os.path.exists(index_dir):
os.mkdir(index_dir)
ix = create_in(index_dir, schema)
添加文档
writer = ix.writer()
writer.add_document(title="Document 1", content="The quick brown fox jumps over the lazy dog")
writer.add_document(title="Document 2", content="The quick brown fox is clever")
writer.commit()
查询文档
with ix.searcher() as searcher:
query = QueryParser("content", ix.schema).parse("fox")
results = searcher.search(query)
for result in results:
print(result['title'])
2、使用Elasticsearch
Elasticsearch是一个分布式搜索和分析引擎,适用于处理大规模数据。
from elasticsearch import Elasticsearch
创建Elasticsearch客户端
es = Elasticsearch()
索引文档
doc1 = {"title": "Document 1", "content": "The quick brown fox jumps over the lazy dog"}
doc2 = {"title": "Document 2", "content": "The quick brown fox is clever"}
es.index(index="documents", id=1, document=doc1)
es.index(index="documents", id=2, document=doc2)
查询文档
query = {
"query": {
"match": {
"content": "fox"
}
}
}
response = es.search(index="documents", body=query)
for hit in response['hits']['hits']:
print(hit['_source']['title'])
七、使用自然语言处理(NLP)技术
自然语言处理(NLP)技术可以帮助你处理和分析文本数据,提取关键信息。
1、使用NLTK
NLTK是一个常用的NLP库,提供了丰富的文本处理功能。
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
from nltk.probability import FreqDist
下载NLTK数据
nltk.download('punkt')
nltk.download('stopwords')
text = "The quick brown fox jumps over the lazy dog. The fox is clever."
分词
tokens = word_tokenize(text)
去除停用词
filtered_tokens = [word for word in tokens if word.lower() not in stopwords.words('english')]
统计词频
fdist = FreqDist(filtered_tokens)
print(fdist.most_common(5))
2、使用spaCy
spaCy是一个高性能的NLP库,适用于构建复杂的文本处理任务。
import spacy
加载语言模型
nlp = spacy.load("en_core_web_sm")
text = "The quick brown fox jumps over the lazy dog. The fox is clever."
处理文本
doc = nlp(text)
提取命名实体
for ent in doc.ents:
print(ent.text, ent.label_)
提取关键词
keywords = [token.text for token in doc if token.is_stop == False and token.is_punct == False]
print(keywords)
总结
在Python中,有多种方法可以使用关键字查询信息,包括使用适当的数据结构、字符串操作、正则表达式、数据库查询、搜索引擎和API、自定义索引和全文搜索,以及自然语言处理技术。每种方法都有其独特的优势和适用场景,选择合适的方法可以帮助你高效地完成信息查询任务。
相关问答FAQs:
如何使用Python进行关键字查询?
在Python中,可以使用多种方法进行关键字查询。最常见的方式是利用内置的数据结构如列表、字典及字符串操作来实现。你可以使用in
关键字检查某个元素是否存在于列表中,或者利用str.contains()
方法来查找字符串中是否包含特定关键字。此外,使用正则表达式也是一种强大的方式,可以处理复杂的匹配需求。
有哪些Python库可以帮助进行关键字查询?
Python提供了多个强大的库来简化关键字查询的操作。pandas
是一个非常流行的数据分析库,可以用来处理数据框中列的查询。re
库则专注于正则表达式,可以处理复杂的字符串匹配。Whoosh
和Elasticsearch
是用于全文搜索的库,适合大规模数据集中的关键字搜索。
在查询过程中如何优化性能?
在进行关键字查询时,性能优化非常重要。使用合适的数据结构是关键,比如使用集合(set)而不是列表,以提高查找效率。此外,考虑使用索引来加速查询,尤其是在处理数据库时。对于大量数据,使用并行处理或异步编程也能显著提高查询速度。使用time
模块进行性能监控和分析,可以帮助你找到瓶颈并进行优化。
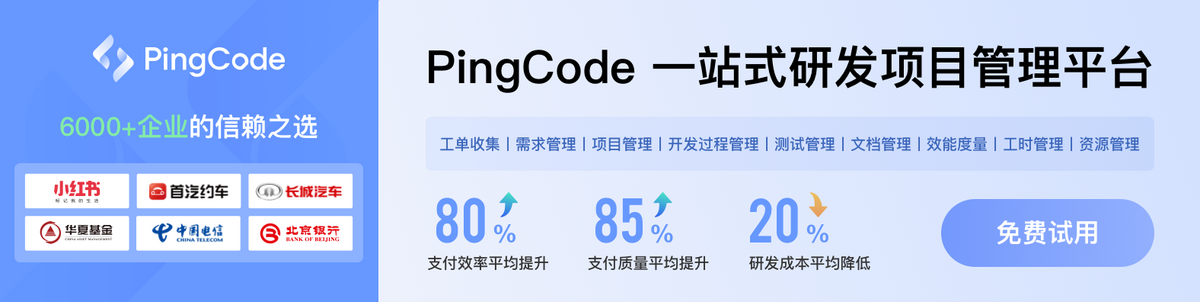