在Python中,你可以使用各种库来绘制图形和图像。对于绘制国旗,最常用的库是Matplotlib和Turtle。下面我将详细介绍如何使用这两个库分别绘制中国国旗。
一、使用Matplotlib绘制中国国旗
Matplotlib是一个广泛使用的绘图库,适用于生成各种图表和图像。要绘制中国国旗,我们需要结合使用Matplotlib的基本图形绘制功能。
import matplotlib.pyplot as plt
import matplotlib.patches as patches
def draw_china_flag():
# 创建图形和轴
fig, ax = plt.subplots()
# 绘制红色背景
ax.add_patch(patches.Rectangle((0, 0), 30, 20, color='red'))
# 五角星函数
def draw_star(x_center, y_center, size, color):
star = patches.RegularPolygon((x_center, y_center), numVertices=5, radius=size, orientation=0, color=color)
ax.add_patch(star)
# 绘制大星星
draw_star(5, 15, 3, 'yellow')
# 小星星的位置和旋转角度
small_stars_positions = [(10, 18), (12, 16), (12, 13), (10, 11)]
for i, (x, y) in enumerate(small_stars_positions):
draw_star(x, y, 1, 'yellow')
# 设置轴的范围和隐藏轴
ax.set_xlim(0, 30)
ax.set_ylim(0, 20)
ax.axis('off')
# 显示图像
plt.show()
draw_china_flag()
二、使用Turtle绘制中国国旗
Turtle是Python的标准库之一,适合绘制简单的图形和图像。下面是一个使用Turtle绘制中国国旗的示例。
import turtle
def draw_star(x, y, size, color):
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.begin_fill()
turtle.color(color)
for _ in range(5):
turtle.forward(size)
turtle.right(144)
turtle.end_fill()
def draw_china_flag():
# 设置屏幕
screen = turtle.Screen()
screen.setup(width=600, height=400)
# 设置画笔
turtle.speed(10)
turtle.penup()
turtle.goto(-300, 200)
turtle.pendown()
turtle.begin_fill()
turtle.color('red')
for _ in range(2):
turtle.forward(600)
turtle.right(90)
turtle.forward(400)
turtle.right(90)
turtle.end_fill()
# 绘制大星星
draw_star(-240, 160, 60, 'yellow')
# 小星星的位置和旋转角度
small_stars_positions = [(-140, 180), (-120, 140), (-120, 100), (-140, 60)]
for (x, y) in small_stars_positions:
draw_star(x, y, 20, 'yellow')
# 完成绘制
turtle.hideturtle()
turtle.done()
draw_china_flag()
三、总结
通过上述两种方法,你可以使用Python中的Matplotlib和Turtle库来绘制中国国旗。Matplotlib适合生成高质量的静态图像,而Turtle则适合动态绘制和教学演示。选择合适的工具可以帮助你更好地完成绘图任务。
相关问答FAQs:
如何用Python绘制国旗的基本形状?
在Python中,绘制国旗的基本形状可以使用图形库如Matplotlib或Turtle。使用Matplotlib时,可以通过创建不同的矩形和颜色来表示国旗的不同部分,而使用Turtle库则可以通过简单的绘制命令来创建更复杂的形状和图案。选择合适的库和方法将帮助你更好地实现国旗的设计。
有哪些库可以帮助我绘制复杂的国旗图案?
除了Matplotlib和Turtle,其他一些库如Pygame和PIL(Pillow)也可以用于绘制国旗。Pygame适合用于创建图形界面和游戏,而PIL则适合处理图像和创建图案。根据你的需求,选择合适的库可以提升绘制效率和效果。
在绘制国旗时如何选择颜色和比例?
每个国家的国旗都有特定的颜色和比例。通常可以在网上查找官方的国旗规范以获取准确的信息。在绘制时,可以使用RGB或十六进制颜色代码来定义颜色,并确保按照比例分配各个部分。这将使你的国旗更加真实和具有代表性。
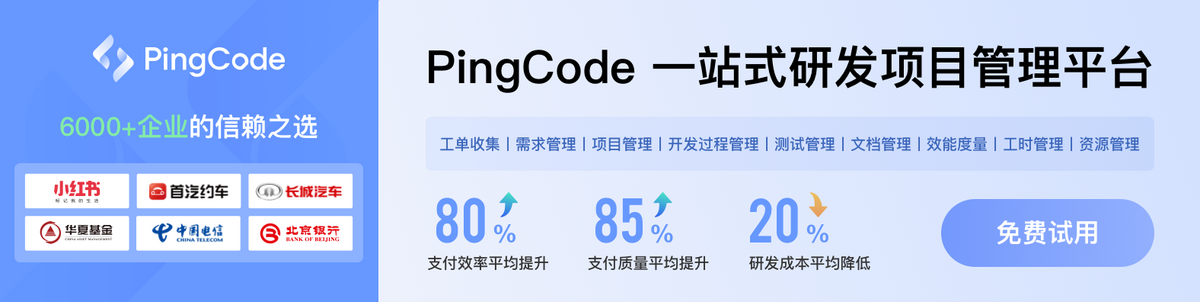