在Python中匹配字符串长度的方法有多种,包括使用内置函数、正则表达式等。可以通过len()函数、使用正则表达式、利用列表解析、借助filter函数等方法来匹配字符串长度。其中,使用len()函数是最直接和常用的方法,适合大多数情况下的字符串长度匹配需求。例如,可以使用len()函数来检查一个字符串的长度是否符合特定的要求,从而决定是否执行后续操作。
一、使用len()函数
使用内置的len()函数是Python中最简单、最常用的方法来匹配字符串长度。len()函数返回字符串的字符数,可以用于条件判断、比较等操作。
my_string = "Hello, World!"
if len(my_string) == 13:
print("The string length is 13.")
else:
print("The string length is not 13.")
在上面的示例中,len()函数返回字符串的长度13,通过条件判断可以匹配指定的长度。
二、使用正则表达式
正则表达式(Regular Expression, regex)是一种强大的字符串匹配工具,可以用来匹配特定长度的字符串。Python的re模块提供了正则表达式的支持。
import re
pattern = r'^.{5}$' # 匹配长度为5的字符串
my_string = "Hello"
if re.match(pattern, my_string):
print("The string length is 5.")
else:
print("The string length is not 5.")
在这个示例中,正则表达式模式^.{5}$
表示匹配任意长度为5的字符串。通过re.match()函数来匹配字符串长度。
三、列表解析
列表解析(List Comprehension)是一种简洁的方式来创建和过滤列表。通过列表解析,可以筛选出符合特定长度的字符串。
strings = ["apple", "banana", "cherry", "date"]
length = 5
matched_strings = [s for s in strings if len(s) == length]
print(matched_strings) # 输出 ['apple']
在这个示例中,通过列表解析筛选出长度为5的字符串。
四、使用filter函数
filter()函数用于过滤序列,过滤掉不符合条件的元素,返回一个迭代器对象。结合lambda函数,可以用于匹配字符串长度。
strings = ["apple", "banana", "cherry", "date"]
length = 5
matched_strings = list(filter(lambda s: len(s) == length, strings))
print(matched_strings) # 输出 ['apple']
在这个示例中,filter()函数过滤出长度为5的字符串。
五、使用自定义函数
有时需要根据特定需求编写自定义函数来匹配字符串长度。自定义函数可以灵活地处理不同的匹配需求。
def is_length(string, length):
return len(string) == length
my_string = "Hello"
length = 5
if is_length(my_string, length):
print("The string length is 5.")
else:
print("The string length is not 5.")
在这个示例中,is_length()函数用于检查字符串是否具有指定的长度。
六、结合其他字符串操作
可以结合其他字符串操作来匹配字符串长度,例如字符串切片、格式化等。
my_string = "Hello, World!"
substring = my_string[:5] # 截取前5个字符
if len(substring) == 5:
print("The substring length is 5.")
else:
print("The substring length is not 5.")
在这个示例中,通过字符串切片截取前5个字符,并匹配其长度。
七、匹配多种长度
有时需要匹配多种长度的字符串,可以使用集合、列表等数据结构来表示多种长度,并进行匹配。
allowed_lengths = {5, 7, 9}
my_string = "cherry"
if len(my_string) in allowed_lengths:
print("The string length is allowed.")
else:
print("The string length is not allowed.")
在这个示例中,通过集合allowed_lengths表示允许的字符串长度,并进行匹配。
八、匹配字符串列表中的每个字符串长度
在处理字符串列表时,可能需要对每个字符串进行长度匹配。可以使用循环、列表解析等方法来实现。
strings = ["apple", "banana", "cherry", "date"]
length = 5
for s in strings:
if len(s) == length:
print(f"The string '{s}' length is {length}.")
else:
print(f"The string '{s}' length is not {length}.")
在这个示例中,通过循环遍历字符串列表,并匹配每个字符串的长度。
九、与其他条件结合
可以将字符串长度匹配与其他条件结合使用,以满足复杂的匹配需求。
my_string = "Hello, World!"
if len(my_string) > 5 and my_string.startswith("Hello"):
print("The string length is greater than 5 and starts with 'Hello'.")
else:
print("The string length is not greater than 5 or does not start with 'Hello'.")
在这个示例中,将字符串长度匹配与字符串开头匹配结合使用。
十、匹配Unicode字符串长度
对于包含Unicode字符的字符串,len()函数可以正确计算字符数,而不是字节数。
my_string = "你好,世界!"
if len(my_string) == 5:
print("The Unicode string length is 5.")
else:
print("The Unicode string length is not 5.")
在这个示例中,len()函数正确计算了包含Unicode字符的字符串长度。
十一、使用第三方库
有些第三方库提供了更高级的字符串操作功能,可以用于匹配字符串长度。例如,使用numpy库处理大规模字符串数据。
import numpy as np
strings = np.array(["apple", "banana", "cherry", "date"])
length = 5
matched_strings = strings[np.vectorize(len)(strings) == length]
print(matched_strings) # 输出 ['apple']
在这个示例中,通过numpy库处理大规模字符串数据,并匹配字符串长度。
十二、性能优化
在处理大量字符串时,性能优化是一个重要考虑因素。可以通过并行处理、多线程等方法提高匹配效率。
from concurrent.futures import ThreadPoolExecutor
strings = ["apple", "banana", "cherry", "date"]
length = 5
def match_length(s):
return len(s) == length
with ThreadPoolExecutor() as executor:
results = list(executor.map(match_length, strings))
print(results) # 输出 [True, False, False, False]
在这个示例中,通过ThreadPoolExecutor并行处理字符串长度匹配,提高处理效率。
通过以上方法,您可以在Python中灵活地匹配字符串长度,满足不同场景的需求。不同的方法有各自的适用场景,选择合适的方法可以提高代码的可读性和性能。
相关问答FAQs:
在 Python 中,如何检查字符串的长度是否符合特定条件?
您可以使用 len()
函数来获取字符串的长度,并通过比较操作符来检查其是否符合特定条件。例如,若要检查字符串是否至少有5个字符,可以使用以下代码:
my_string = "Hello"
if len(my_string) >= 5:
print("字符串长度符合要求")
else:
print("字符串长度不符合要求")
如何在 Python 中匹配字符串长度范围?
可以结合 len()
函数和逻辑运算符来判断字符串长度是否在某个范围内。例如,若要检查字符串长度是否在3到8个字符之间,可以这样做:
my_string = "Python"
if 3 <= len(my_string) <= 8:
print("字符串长度在范围内")
else:
print("字符串长度不在范围内")
在处理用户输入时,如何确保字符串长度的有效性?
为了确保用户输入的字符串长度有效,您可以使用 input()
函数获取字符串,并结合 len()
进行验证。例如,以下代码强制要求用户输入一个长度在4到10之间的字符串:
user_input = input("请输入一个字符串(长度需在4到10之间):")
if 4 <= len(user_input) <= 10:
print("输入有效")
else:
print("输入无效,请确保字符串长度在4到10之间")
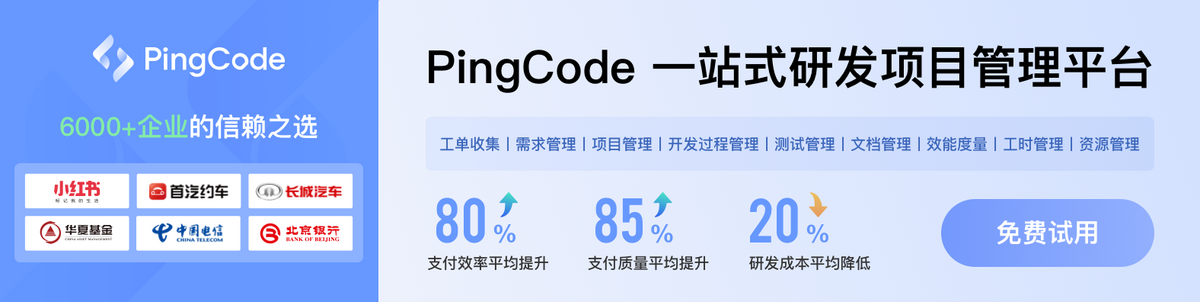