要将PPT文件转换为Word文件,可以使用Python中的一些库,比如python-pptx
、python-docx
和pptx2pdf
。首先,解析PPT文件,提取内容,然后将提取的内容写入Word文档。以下是实现这一过程的详细步骤:提取PPT内容、创建Word文档、写入PPT内容到Word文档。
提取PPT内容:我们可以使用python-pptx
库来读取PPT文件,并提取其中的文本和图像。创建Word文档:接下来,我们使用python-docx
库来创建Word文档,并将提取的PPT内容写入其中。写入PPT内容到Word文档:最后,将提取的文本和图像写入新的Word文档中。
接下来,我们将详细介绍如何实现这些步骤。
一、安装必要的库
在开始之前,您需要安装以下Python库:
pip install python-pptx
pip install python-docx
pip install pptx2pdf
python-pptx
用于读取和操作PPT文件,python-docx
用于创建和操作Word文档,pptx2pdf
用于将PPT转换为PDF(如果需要)。
二、读取PPT文件并提取内容
首先,我们需要读取PPT文件并提取其中的文本和图像。以下是一个示例代码,说明如何使用python-pptx
库来读取PPT文件并提取其中的文本:
from pptx import Presentation
def extract_ppt_content(ppt_file):
presentation = Presentation(ppt_file)
content = []
for slide in presentation.slides:
slide_content = {"text": [], "images": []}
for shape in slide.shapes:
if hasattr(shape, "text"):
slide_content["text"].append(shape.text)
if shape.shape_type == 13: # 13 indicates a picture
slide_content["images"].append(shape.image)
content.append(slide_content)
return content
在这个示例中,我们读取PPT文件并提取每个幻灯片中的文本和图像。提取的内容存储在一个列表中,每个幻灯片的内容作为字典存储。
三、创建Word文档并写入内容
接下来,我们使用python-docx
库来创建Word文档,并将提取的内容写入其中。以下是示例代码:
from docx import Document
from docx.shared import Inches
def create_word_doc(content, output_file):
doc = Document()
for slide_content in content:
for text in slide_content["text"]:
doc.add_paragraph(text)
for image in slide_content["images"]:
image_stream = BytesIO(image.blob)
doc.add_picture(image_stream, width=Inches(6))
doc.add_page_break()
doc.save(output_file)
在这个示例中,我们创建了一个新的Word文档,并将提取的文本和图像写入其中。每个幻灯片的内容之间插入一个分页符。
四、完整示例代码
以下是完整示例代码,将PPT文件转换为Word文件:
from pptx import Presentation
from docx import Document
from docx.shared import Inches
from io import BytesIO
def extract_ppt_content(ppt_file):
presentation = Presentation(ppt_file)
content = []
for slide in presentation.slides:
slide_content = {"text": [], "images": []}
for shape in slide.shapes:
if hasattr(shape, "text"):
slide_content["text"].append(shape.text)
if shape.shape_type == 13: # 13 indicates a picture
slide_content["images"].append(shape.image)
content.append(slide_content)
return content
def create_word_doc(content, output_file):
doc = Document()
for slide_content in content:
for text in slide_content["text"]:
doc.add_paragraph(text)
for image in slide_content["images"]:
image_stream = BytesIO(image.blob)
doc.add_picture(image_stream, width=Inches(6))
doc.add_page_break()
doc.save(output_file)
def ppt_to_word(ppt_file, word_file):
content = extract_ppt_content(ppt_file)
create_word_doc(content, word_file)
Example usage
ppt_file = "example.pptx"
word_file = "output.docx"
ppt_to_word(ppt_file, word_file)
五、处理文本格式
在提取PPT中的文本并写入Word文档时,可能需要保留文本的格式,如字体、颜色和大小。可以使用python-pptx
库提供的方法提取文本格式,并在写入Word文档时应用这些格式。以下是一个示例,说明如何提取和应用文本格式:
from pptx import Presentation
from docx import Document
from docx.shared import Pt, RGBColor
from io import BytesIO
def extract_ppt_content_with_format(ppt_file):
presentation = Presentation(ppt_file)
content = []
for slide in presentation.slides:
slide_content = {"text": [], "images": []}
for shape in slide.shapes:
if hasattr(shape, "text"):
text_frame = shape.text_frame
for paragraph in text_frame.paragraphs:
text_data = {"text": paragraph.text, "font": None, "size": None, "color": None}
if paragraph.runs:
run = paragraph.runs[0]
text_data["font"] = run.font.name
text_data["size"] = run.font.size.pt if run.font.size else None
text_data["color"] = run.font.color.rgb if run.font.color.rgb else None
slide_content["text"].append(text_data)
if shape.shape_type == 13: # 13 indicates a picture
slide_content["images"].append(shape.image)
content.append(slide_content)
return content
def create_word_doc_with_format(content, output_file):
doc = Document()
for slide_content in content:
for text_data in slide_content["text"]:
paragraph = doc.add_paragraph()
run = paragraph.add_run(text_data["text"])
if text_data["font"]:
run.font.name = text_data["font"]
if text_data["size"]:
run.font.size = Pt(text_data["size"])
if text_data["color"]:
run.font.color.rgb = RGBColor(text_data["color"].red, text_data["color"].green, text_data["color"].blue)
for image in slide_content["images"]:
image_stream = BytesIO(image.blob)
doc.add_picture(image_stream, width=Inches(6))
doc.add_page_break()
doc.save(output_file)
def ppt_to_word_with_format(ppt_file, word_file):
content = extract_ppt_content_with_format(ppt_file)
create_word_doc_with_format(content, word_file)
Example usage
ppt_file = "example.pptx"
word_file = "output_with_format.docx"
ppt_to_word_with_format(ppt_file, word_file)
在这个示例中,我们提取了PPT文本的字体、大小和颜色,并在写入Word文档时应用这些格式。
六、处理图像和表格
除了文本和图像,PPT文件中可能还包含表格和其他复杂的内容。我们可以使用python-pptx
库提供的方法来提取这些内容,并使用python-docx
库提供的方法将它们写入Word文档。以下是一个示例,说明如何提取和写入表格:
from pptx import Presentation
from docx import Document
from docx.shared import Inches
from io import BytesIO
def extract_ppt_content_with_tables(ppt_file):
presentation = Presentation(ppt_file)
content = []
for slide in presentation.slides:
slide_content = {"text": [], "images": [], "tables": []}
for shape in slide.shapes:
if hasattr(shape, "text"):
slide_content["text"].append(shape.text)
if shape.shape_type == 13: # 13 indicates a picture
slide_content["images"].append(shape.image)
if shape.shape_type == 19: # 19 indicates a table
table_content = []
table = shape.table
for row in table.rows:
row_content = [cell.text for cell in row.cells]
table_content.append(row_content)
slide_content["tables"].append(table_content)
content.append(slide_content)
return content
def create_word_doc_with_tables(content, output_file):
doc = Document()
for slide_content in content:
for text in slide_content["text"]:
doc.add_paragraph(text)
for image in slide_content["images"]:
image_stream = BytesIO(image.blob)
doc.add_picture(image_stream, width=Inches(6))
for table_content in slide_content["tables"]:
table = doc.add_table(rows=len(table_content), cols=len(table_content[0]))
for i, row_content in enumerate(table_content):
for j, cell_text in enumerate(row_content):
table.cell(i, j).text = cell_text
doc.add_page_break()
doc.save(output_file)
def ppt_to_word_with_tables(ppt_file, word_file):
content = extract_ppt_content_with_tables(ppt_file)
create_word_doc_with_tables(content, word_file)
Example usage
ppt_file = "example.pptx"
word_file = "output_with_tables.docx"
ppt_to_word_with_tables(ppt_file, word_file)
在这个示例中,我们提取了PPT文件中的表格内容,并将其写入Word文档。
七、处理复杂布局
在实际应用中,PPT文件可能包含复杂的布局和内容,如多列文本、嵌套列表和图表。处理这些复杂布局需要更多的代码和逻辑。以下是一些处理复杂布局的建议:
- 多列文本:可以使用
python-pptx
库提供的方法来识别多列文本,并在写入Word文档时创建相应的多列布局。 - 嵌套列表:可以使用递归方法来提取和写入嵌套列表的内容。
- 图表:可以使用
python-pptx
库提供的方法来提取图表数据,并使用python-docx
库提供的方法将其写入Word文档。
以下是一个处理复杂布局的示例代码:
from pptx import Presentation
from docx import Document
from docx.shared import Inches
from io import BytesIO
def extract_ppt_content_complex(ppt_file):
presentation = Presentation(ppt_file)
content = []
for slide in presentation.slides:
slide_content = {"text": [], "images": [], "tables": [], "charts": []}
for shape in slide.shapes:
if hasattr(shape, "text"):
slide_content["text"].append(shape.text)
if shape.shape_type == 13: # 13 indicates a picture
slide_content["images"].append(shape.image)
if shape.shape_type == 19: # 19 indicates a table
table_content = []
table = shape.table
for row in table.rows:
row_content = [cell.text for cell in row.cells]
table_content.append(row_content)
slide_content["tables"].append(table_content)
if shape.shape_type == 3: # 3 indicates a chart
slide_content["charts"].append(shape.chart)
content.append(slide_content)
return content
def create_word_doc_complex(content, output_file):
doc = Document()
for slide_content in content:
for text in slide_content["text"]:
doc.add_paragraph(text)
for image in slide_content["images"]:
image_stream = BytesIO(image.blob)
doc.add_picture(image_stream, width=Inches(6))
for table_content in slide_content["tables"]:
table = doc.add_table(rows=len(table_content), cols=len(table_content[0]))
for i, row_content in enumerate(table_content):
for j, cell_text in enumerate(row_content):
table.cell(i, j).text = cell_text
for chart in slide_content["charts"]:
chart_data = extract_chart_data(chart)
add_chart_to_word(doc, chart_data)
doc.add_page_break()
doc.save(output_file)
def extract_chart_data(chart):
# Extract chart data and return in a suitable format
pass
def add_chart_to_word(doc, chart_data):
# Add chart data to Word document
pass
def ppt_to_word_complex(ppt_file, word_file):
content = extract_ppt_content_complex(ppt_file)
create_word_doc_complex(content, word_file)
Example usage
ppt_file = "example.pptx"
word_file = "output_complex.docx"
ppt_to_word_complex(ppt_file, word_file)
在这个示例中,我们提取了PPT文件中的图表数据,并将其写入Word文档。需要注意的是,提取和写入图表数据的具体实现取决于您的需求和PPT文件的内容。
总结起来,将PPT文件转换为Word文件需要多个步骤,包括提取PPT内容、创建Word文档、处理文本格式、处理图像和表格以及处理复杂布局。通过使用python-pptx
和python-docx
库,您可以实现这一过程,并根据具体需求进行调整和扩展。
相关问答FAQs:
如何使用Python将PPT文件转换为Word文档?
要将PPT转换为Word,您可以使用Python的python-pptx
库读取PPT内容,并利用python-docx
库将内容写入Word文档。这两个库可以轻松处理文件的读取和写入操作。
转换后Word文档中的格式会保持吗?
虽然PPT中的文本和图像可以被提取,但转换后的Word文档可能无法完全保留PPT中的所有格式和布局。建议在转换后检查Word文档,并进行必要的格式调整。
使用Python转换PPT时有什么常见的错误?
常见的错误可能包括文件路径错误、PPT文件损坏或库未正确安装等。确保在运行代码前安装相关库,并检查文件路径是否正确。
是否有现成的工具可以帮助完成PPT到Word的转换?
除了手动编写代码,您还可以寻找一些开源项目或在线工具,这些工具可能已经集成了PPT到Word的转换功能,可以节省您的时间和精力。
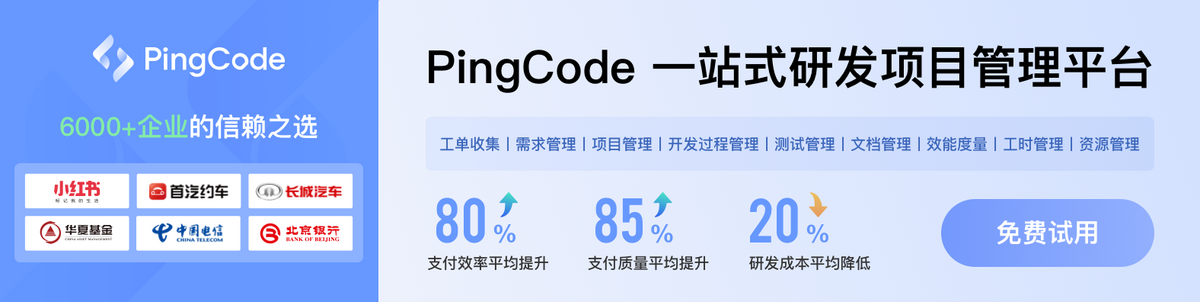