Python可以通过多种方式实现不区分大小写(忽略大小写)操作,常见的方法包括:使用字符串的lower()或upper()方法将字符串转换为小写或大写、使用正则表达式中的re.IGNORECASE标志、在比较字符串时使用casefold()方法等。 其中,使用字符串的lower()方法是最直接和常见的方式,通过将所有字符串转换为小写后再进行比较,可以方便地实现不区分大小写的效果。接下来我们详细介绍这些方法。
一、使用字符串的lower()或upper()方法
Python中的字符串对象提供了lower()
和upper()
方法,可以将字符串转换为小写或大写。通过将两个字符串都转换为小写(或大写)后进行比较,可以实现不区分大小写的效果。
# Example using lower() method
str1 = "Hello"
str2 = "hello"
if str1.lower() == str2.lower():
print("The strings are equal (ignoring case).")
else:
print("The strings are not equal.")
在这个例子中,我们将str1
和str2
都转换为小写,然后进行比较,从而实现了不区分大小写的字符串比较。upper()
方法的使用方式类似:
# Example using upper() method
str1 = "Hello"
str2 = "HELLO"
if str1.upper() == str2.upper():
print("The strings are equal (ignoring case).")
else:
print("The strings are not equal.")
二、使用正则表达式中的re.IGNORECASE标志
Python的正则表达式库re
提供了IGNORECASE
标志,可以在匹配过程中忽略大小写。这对于进行模式匹配时非常有用。
import re
pattern = "hello"
text = "Hello, world!"
Example using re.IGNORECASE
if re.search(pattern, text, re.IGNORECASE):
print("Pattern found (ignoring case).")
else:
print("Pattern not found.")
在这个例子中,re.search()
函数使用了IGNORECASE
标志,因此它在匹配模式时忽略了大小写。
三、使用字符串的casefold()方法
casefold()
方法是一个更强大的方法,它不仅将字符串转换为小写,还处理了某些特定语言的字符,使得比较更加准确。casefold()
方法的使用方式与lower()
方法类似。
# Example using casefold() method
str1 = "Straße"
str2 = "strasse"
if str1.casefold() == str2.casefold():
print("The strings are equal (ignoring case).")
else:
print("The strings are not equal.")
在这个例子中,casefold()
方法正确处理了德语字符,使得str1
和str2
在比较时被视为相等。
四、使用其他方法
除了上述方法,还有其他方式可以实现不区分大小写的操作。例如,在进行字符串排序时,可以使用自定义的键函数,将字符串转换为小写或大写后再进行排序。
# Example using sorted() with a custom key function
words = ["Banana", "apple", "Cherry"]
sorted_words = sorted(words, key=lambda s: s.lower())
print(sorted_words)
在这个例子中,我们使用了sorted()
函数,并传递了一个自定义的键函数lambda s: s.lower()
,将每个字符串转换为小写后再进行排序。
总结
通过上述几种方法,我们可以在Python中方便地实现不区分大小写的字符串操作。具体选择哪种方法取决于具体的应用场景和需求。对于简单的字符串比较,使用lower()
或upper()
方法通常是最直接和有效的方式;对于复杂的模式匹配,可以使用正则表达式中的IGNORECASE
标志;而对于需要处理特定语言字符的场景,casefold()
方法则是最合适的选择。
相关问答FAQs:
如何在Python中实现不区分大小写的字符串比较?
在Python中,可以使用str.lower()
或str.upper()
方法将字符串转换为小写或大写,然后再进行比较。这种方法确保了在比较过程中不会受到大小写的影响。例如,"Hello".lower() == "hello".lower()
将返回True
。
Python的正则表达式如何处理不区分大小写?
使用Python的re
模块时,可以通过在正则表达式中添加re.IGNORECASE
标志来实现不区分大小写的匹配。例如,re.search('pattern', 'Text', re.IGNORECASE)
将会匹配不论'Text'
的大小写如何。
在Python字典中,如何确保键名不区分大小写?
创建一个不区分大小写的字典可以通过继承内置的dict
类,重写__setitem__
和__getitem__
方法来实现。在这些方法中,确保将键转换为统一的大小写形式(如小写)后再进行存储和检索。这样,字典在处理键时就会忽略大小写差异。
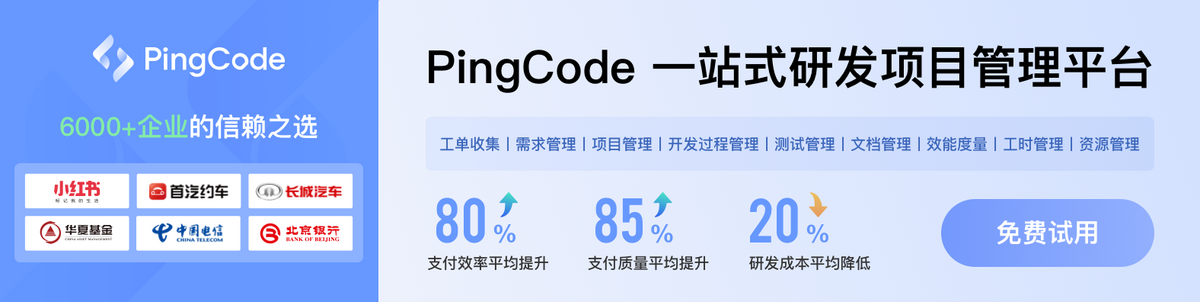