在Python中,判断字符串大小的方法有多种,可以使用运算符比较、使用内置函数、使用正则表达式等。最常用和直接的方法是使用运算符进行比较,这种方法简单直观,适用于大多数场景。下面将详细展开这种方法,并介绍其他方法和注意事项。
一、使用运算符比较
在Python中,可以使用常见的比较运算符(如 <
, <=
, >
, >=
, ==
, !=
等)来比较字符串的大小。这些运算符是基于字符串中字符的Unicode码点(ASCII码)进行比较的。
str1 = "apple"
str2 = "banana"
if str1 < str2:
print(f"'{str1}' is less than '{str2}'")
elif str1 > str2:
print(f"'{str1}' is greater than '{str2}'")
else:
print(f"'{str1}' is equal to '{str2}'")
在这个例子中,Python会比较字符串中每个字符的Unicode码点,直到找到第一个不同的字符为止。比如,"apple" 的第一个字符 'a' 的Unicode码点小于 "banana" 的第一个字符 'b' 的Unicode码点,因此 "apple" 小于 "banana"。
二、使用内置函数
有些内置函数也可以帮助判断字符串大小,比如 ord()
和 cmp()
(在Python 3中已移除,需要使用functools.cmp_to_key()
)。
1. 使用 ord()
函数
ord()
函数可以将字符转换为Unicode码点,可以用于逐字符比较。
str1 = "apple"
str2 = "banana"
def compare_strings(s1, s2):
for c1, c2 in zip(s1, s2):
if ord(c1) < ord(c2):
return -1
elif ord(c1) > ord(c2):
return 1
if len(s1) < len(s2):
return -1
elif len(s1) > len(s2):
return 1
else:
return 0
result = compare_strings(str1, str2)
if result == -1:
print(f"'{str1}' is less than '{str2}'")
elif result == 1:
print(f"'{str1}' is greater than '{str2}'")
else:
print(f"'{str1}' is equal to '{str2}'")
2. 使用 functools.cmp_to_key()
在Python 3中,cmp()
函数被移除了,但可以使用 functools.cmp_to_key()
来实现类似的功能。
import functools
str1 = "apple"
str2 = "banana"
def compare_strings(s1, s2):
if s1 < s2:
return -1
elif s1 > s2:
return 1
else:
return 0
key_func = functools.cmp_to_key(compare_strings)
sorted_strings = sorted([str1, str2], key=key_func)
print(f"Sorted strings: {sorted_strings}")
三、使用正则表达式
正则表达式通常用于字符串搜索和匹配,但也可以用于比较特定模式的字符串。
import re
pattern = re.compile(r'^[a-zA-Z]+$')
str1 = "apple"
str2 = "banana"
if pattern.match(str1) and pattern.match(str2):
if str1 < str2:
print(f"'{str1}' is less than '{str2}'")
elif str1 > str2:
print(f"'{str1}' is greater than '{str2}'")
else:
print(f"'{str1}' is equal to '{str2}'")
else:
print("One of the strings does not match the pattern")
四、注意事项
1. 区分大小写
在比较字符串时,默认是区分大小写的。如果需要忽略大小写,可以将字符串转换为相同的大小写。
str1 = "Apple"
str2 = "banana"
if str1.lower() < str2.lower():
print(f"'{str1}' is less than '{str2}'")
elif str1.lower() > str2.lower():
print(f"'{str1}' is greater than '{str2}'")
else:
print(f"'{str1}' is equal to '{str2}'")
2. 非字母字符
字符串中可能包含非字母字符,如数字和符号,这些字符的Unicode码点与字母字符不同。在比较字符串时,这些字符也会参与比较。
str1 = "apple1"
str2 = "apple2"
if str1 < str2:
print(f"'{str1}' is less than '{str2}'")
elif str1 > str2:
print(f"'{str1}' is greater than '{str2}'")
else:
print(f"'{str1}' is equal to '{str2}'")
3. 国际化字符
对于包含国际化字符的字符串,比较时要特别注意字符的Unicode码点。
str1 = "mañana"
str2 = "manzana"
if str1 < str2:
print(f"'{str1}' is less than '{str2}'")
elif str1 > str2:
print(f"'{str1}' is greater than '{str2}'")
else:
print(f"'{str1}' is equal to '{str2}'")
五、总结
在Python中判断字符串大小的方法有很多,最常用的是直接使用比较运算符。这种方法简单直观,适用于大多数场景。其他方法如使用内置函数和正则表达式也可以根据具体需求进行选择。在实际应用中,还需要注意字符串的大小写、非字母字符和国际化字符对比较结果的影响。掌握这些方法和注意事项,可以帮助我们在不同场景下正确判断字符串的大小。
相关问答FAQs:
如何在Python中比较两个字符串的大小?
在Python中,可以使用比较运算符直接比较两个字符串的大小。字符串的比较是基于字母的字典顺序进行的。例如,使用“<”、“>”、“==”等运算符可以判断字符串的相对顺序。示例代码如下:
str1 = "apple"
str2 = "banana"
if str1 < str2:
print(f"{str1} 在字典顺序中排在 {str2} 之前。")
Python中的字符串比较是否区分大小写?
是的,Python中的字符串比较是区分大小写的。在进行比较时,大写字母被认为小于小写字母,因此“Apple”会被认为小于“apple”。如果希望进行不区分大小写的比较,可以使用.lower()
或.upper()
方法将字符串转换为相同的大小写进行比较。示例代码如下:
if str1.lower() < str2.lower():
print(f"{str1} 在不区分大小写的情况下排在 {str2} 之前。")
如何获取字符串的排序顺序?
如果想要获取多个字符串的排序顺序,可以使用Python的内置sorted()
函数。这个函数会返回一个新的列表,其中包含按照字典顺序排列的字符串。示例代码如下:
strings = ["banana", "apple", "cherry"]
sorted_strings = sorted(strings)
print(sorted_strings) # 输出 ['apple', 'banana', 'cherry']
这种方法可以帮助你快速得到任何字符串列表的有序版本。
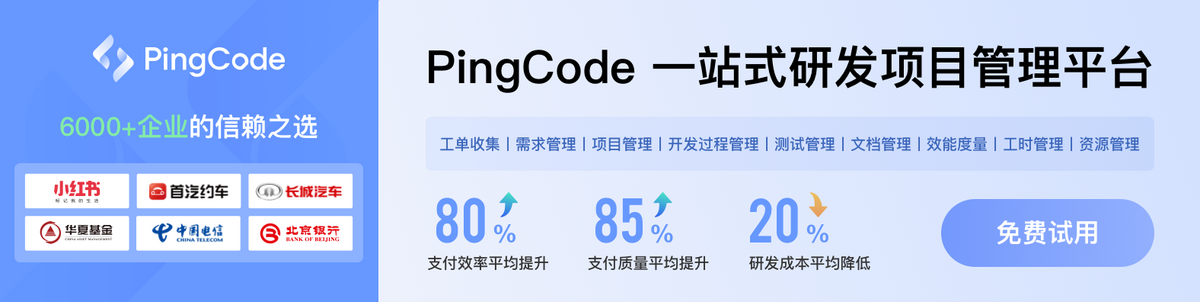