在Python中删除指定位置的图片,可以使用os模块、shutil模块、路径库等方法。 其中,os.remove()方法是最常用的一种,因为它简单直接。下面我将详细介绍如何使用这些方法,并提供具体的代码示例。
删除文件的操作是系统级的操作,因此在进行这些操作之前,请务必确保你有删除文件的权限,并且已经备份好重要的文件。
一、使用os模块删除文件
os模块提供了一个简单的方法来删除文件,那就是os.remove()函数。它接受一个文件路径作为参数,并删除该文件。
示例代码:
import os
指定图片路径
image_path = 'path/to/your/image.jpg'
删除图片
try:
os.remove(image_path)
print(f"{image_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{image_path} not found.")
except PermissionError:
print(f"Permission denied to delete {image_path}.")
except Exception as e:
print(f"Error occurred while deleting {image_path}: {e}")
二、使用shutil模块删除文件
shutil模块提供了更高级的文件操作,包括删除文件。shutil.rmtree()可以删除一个目录及其所有内容。
示例代码:
import shutil
指定图片路径
image_path = 'path/to/your/image.jpg'
删除图片
try:
shutil.rmtree(image_path)
print(f"{image_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{image_path} not found.")
except PermissionError:
print(f"Permission denied to delete {image_path}.")
except Exception as e:
print(f"Error occurred while deleting {image_path}: {e}")
注意:shutil.rmtree()通常用于删除目录及其内容,而不是单个文件。
三、使用pathlib库删除文件
pathlib库提供了一个面向对象的方法来处理文件路径。它在Python 3.4中引入,提供了一些方便的方法来操作文件和目录。
示例代码:
from pathlib import Path
指定图片路径
image_path = Path('path/to/your/image.jpg')
删除图片
try:
image_path.unlink()
print(f"{image_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{image_path} not found.")
except PermissionError:
print(f"Permission denied to delete {image_path}.")
except Exception as e:
print(f"Error occurred while deleting {image_path}: {e}")
四、删除目录中的所有图片
有时候我们可能需要删除目录中的所有图片,而不仅仅是某一张图片。这可以通过遍历目录并删除所有图片文件来实现。
示例代码:
import os
指定目录路径
directory_path = 'path/to/your/directory'
获取目录中的所有文件
files = os.listdir(directory_path)
删除目录中的所有图片
for file in files:
if file.endswith('.jpg') or file.endswith('.png'):
file_path = os.path.join(directory_path, file)
try:
os.remove(file_path)
print(f"{file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"{file_path} not found.")
except PermissionError:
print(f"Permission denied to delete {file_path}.")
except Exception as e:
print(f"Error occurred while deleting {file_path}: {e}")
五、在GUI应用中删除图片
在某些情况下,我们可能需要在GUI应用中删除图片。这可以使用Tkinter或PyQt等GUI库来实现。
Tkinter示例代码:
import os
import tkinter as tk
from tkinter import filedialog, messagebox
def delete_image():
file_path = filedialog.askopenfilename()
if file_path:
try:
os.remove(file_path)
messagebox.showinfo("Success", f"{file_path} has been deleted successfully.")
except FileNotFoundError:
messagebox.showerror("Error", f"{file_path} not found.")
except PermissionError:
messagebox.showerror("Error", f"Permission denied to delete {file_path}.")
except Exception as e:
messagebox.showerror("Error", f"Error occurred while deleting {file_path}: {e}")
root = tk.Tk()
root.title("Delete Image")
root.geometry("300x200")
delete_button = tk.Button(root, text="Delete Image", command=delete_image)
delete_button.pack(pady=20)
root.mainloop()
总结
删除文件是一个常见的操作,Python提供了多种方法来删除文件或目录中的文件,包括os模块、shutil模块、pathlib库等。 每种方法都有其优缺点,选择合适的方法可以提高代码的可读性和效率。在使用这些方法时,请务必确保你有删除文件的权限,并且已经备份好重要的文件。
无论是单个文件的删除,还是目录中所有图片的删除,Python都有相应的解决方案。希望这篇文章能帮助你更好地理解和使用Python进行文件删除操作。
相关问答FAQs:
如何在Python中删除特定路径下的图片文件?
在Python中,可以使用os
模块中的remove()
函数删除指定路径的图片文件。首先,确保您提供的路径是正确的,并且该文件确实存在。以下是一个简单的示例代码:
import os
# 指定图片的路径
image_path = 'path/to/your/image.jpg'
# 删除图片
if os.path.exists(image_path):
os.remove(image_path)
print(f"{image_path} 已成功删除。")
else:
print("指定的文件不存在。")
使用此方法时,请务必小心,因为删除的文件无法恢复。
如何通过条件判断删除多张图片?
如果您需要根据特定条件删除多张图片,可以使用os
模块结合文件名或文件类型进行筛选。以下是示例代码,删除指定目录下所有.jpg格式的图片:
import os
# 指定目录
directory = 'path/to/your/images/'
# 遍历目录中的文件
for filename in os.listdir(directory):
if filename.endswith('.jpg'):
file_path = os.path.join(directory, filename)
os.remove(file_path)
print(f"{file_path} 已成功删除。")
这种方法可以帮助您批量管理图片文件。
如何确保删除图片文件的安全性?
为了确保删除操作的安全性,建议在执行删除前进行确认。例如,可以提示用户确认是否继续删除操作。以下是一个示例代码:
import os
image_path = 'path/to/your/image.jpg'
# 确认删除
confirm = input(f"您确定要删除 {image_path} 吗? (y/n): ")
if confirm.lower() == 'y':
if os.path.exists(image_path):
os.remove(image_path)
print(f"{image_path} 已成功删除。")
else:
print("指定的文件不存在。")
else:
print("删除操作已取消。")
这种方法能够有效避免误删文件的情况。
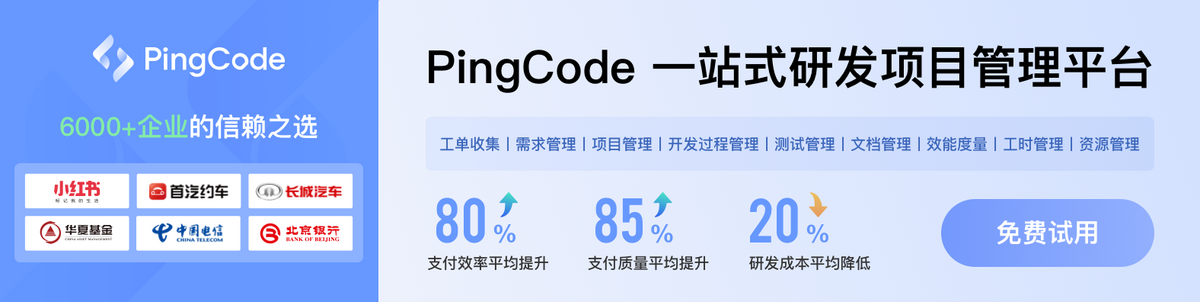