用Python打开数据库连接的步骤为:选择合适的数据库驱动、安装数据库驱动库、编写连接代码、处理连接异常。 下面具体讲解如何用Python打开数据库连接。
一、选择合适的数据库驱动
Python支持多种数据库连接,包括MySQL、PostgreSQL、SQLite、Oracle等。选择合适的数据库驱动是成功连接数据库的第一步。常用的数据库驱动有:
- MySQL: 使用
mysql-connector-python
或PyMySQL
- PostgreSQL: 使用
psycopg2
- SQLite: 使用Python自带的
sqlite3
- Oracle: 使用
cx_Oracle
二、安装数据库驱动库
在连接数据库之前,需要安装相应的数据库驱动库。可以使用pip
命令来安装。例如,安装mysql-connector-python
:
pip install mysql-connector-python
安装psycopg2
:
pip install psycopg2
安装cx_Oracle
:
pip install cx_Oracle
对于SQLite,不需要额外安装,因为Python内置了sqlite3
库。
三、编写连接代码
连接数据库的代码一般包括四个步骤:创建连接、创建游标、执行SQL语句、关闭连接。
1. 创建连接
import mysql.connector
创建连接
conn = mysql.connector.connect(
host='localhost',
user='username',
password='password',
database='database_name'
)
对于PostgreSQL:
import psycopg2
创建连接
conn = psycopg2.connect(
host="localhost",
database="database_name",
user="username",
password="password"
)
对于SQLite:
import sqlite3
创建连接
conn = sqlite3.connect('database.db')
对于Oracle:
import cx_Oracle
创建连接
conn = cx_Oracle.connect('username/password@localhost:1521/orcl')
2. 创建游标
cursor = conn.cursor()
3. 执行SQL语句
cursor.execute("SELECT * FROM table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
4. 关闭连接
cursor.close()
conn.close()
四、处理连接异常
在实际应用中,数据库连接可能会出现各种异常情况,如连接超时、认证失败等。因此,需要使用异常处理来确保程序的稳健性。
import mysql.connector
from mysql.connector import Error
try:
conn = mysql.connector.connect(
host='localhost',
user='username',
password='password',
database='database_name'
)
if conn.is_connected():
print("Successfully connected to the database")
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
except Error as e:
print(f"Error: {e}")
finally:
if conn.is_connected():
cursor.close()
conn.close()
print("The connection is closed")
对于PostgreSQL:
import psycopg2
from psycopg2 import OperationalError
try:
conn = psycopg2.connect(
host="localhost",
database="database_name",
user="username",
password="password"
)
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
except OperationalError as e:
print(f"The error '{e}' occurred")
finally:
if conn:
cursor.close()
conn.close()
print("The connection is closed")
对于SQLite:
import sqlite3
try:
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
except sqlite3.Error as e:
print(f"Error: {e}")
finally:
if conn:
cursor.close()
conn.close()
print("The connection is closed")
对于Oracle:
import cx_Oracle
try:
conn = cx_Oracle.connect('username/password@localhost:1521/orcl')
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
except cx_Oracle.DatabaseError as e:
print(f"Error: {e}")
finally:
if conn:
cursor.close()
conn.close()
print("The connection is closed")
五、使用连接池
在高并发的场景下,频繁创建和销毁数据库连接会带来很大的开销。使用连接池可以有效地提高性能。以下是如何使用连接池的例子。
MySQL连接池
import mysql.connector
from mysql.connector import pooling
dbconfig = {
"database": "database_name",
"user": "username",
"password": "password",
"host": "localhost"
}
try:
connection_pool = mysql.connector.pooling.MySQLConnectionPool(
pool_name="mypool",
pool_size=5,
dbconfig
)
conn = connection_pool.get_connection()
if conn.is_connected():
print("Successfully connected to the database using connection pool")
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
finally:
if conn.is_connected():
cursor.close()
conn.close()
print("The connection is closed")
PostgreSQL连接池
import psycopg2
from psycopg2 import pool
try:
connection_pool = psycopg2.pool.SimpleConnectionPool(1, 20,
user="username",
password="password",
host="localhost",
port="5432",
database="database_name")
if connection_pool:
print("Connection pool created successfully")
conn = connection_pool.getconn()
if conn:
print("Successfully connected to the database using connection pool")
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
finally:
if conn:
cursor.close()
connection_pool.putconn(conn)
print("The connection is returned to the pool")
SQLite连接池
SQLite不支持连接池,因为它是一个轻量级的数据库,通常用于单线程和小型项目。如果需要使用连接池,可以考虑使用外部库如sqlite-pool
.
Oracle连接池
import cx_Oracle
try:
pool = cx_Oracle.SessionPool("username", "password", "localhost:1521/orcl", min=2, max=5, increment=1, threaded=True)
conn = pool.acquire()
print("Successfully connected to the database using connection pool")
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
finally:
if conn:
cursor.close()
pool.release(conn)
print("The connection is returned to the pool")
六、使用ORM
使用ORM(对象关系映射)可以简化数据库操作。常用的ORM库有SQLAlchemy和Django ORM。
使用SQLAlchemy
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
DATABASE_URL = "mysql+mysqlconnector://username:password@localhost:3306/database_name"
engine = create_engine(DATABASE_URL)
Session = sessionmaker(bind=engine)
session = Session()
result = session.execute("SELECT * FROM table_name")
for row in result:
print(row)
session.close()
使用Django ORM
首先,需要安装Django:
pip install django
然后创建一个Django项目,并配置数据库连接。在settings.py
中配置数据库:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'database_name',
'USER': 'username',
'PASSWORD': 'password',
'HOST': 'localhost',
'PORT': '3306',
}
}
使用Django ORM进行查询:
from django.db import models
class MyModel(models.Model):
field1 = models.CharField(max_length=100)
field2 = models.IntegerField()
results = MyModel.objects.all()
for result in results:
print(result.field1, result.field2)
总结,使用Python连接数据库涉及选择合适的数据库驱动、安装驱动库、编写连接代码、处理异常、使用连接池及ORM等步骤。通过这些步骤,可以高效、稳健地进行数据库操作。
相关问答FAQs:
如何选择合适的数据库库来连接Python?
选择合适的数据库库主要取决于你所使用的数据库类型。常见的数据库包括MySQL、PostgreSQL、SQLite、MongoDB等。对于关系型数据库,使用MySQL Connector
或psycopg2
(用于PostgreSQL)是常见的选择。如果你使用的是SQLite,内置的sqlite3
库就能满足需求。而对于非关系型数据库,例如MongoDB,可以使用PyMongo
库。确保选择的库与数据库类型兼容,并检查其文档以获取详细的使用方法。
在Python中如何处理数据库连接错误?
处理数据库连接错误是确保应用程序稳定性的重要部分。在打开连接时,使用try...except
语句来捕获异常。常见的异常包括连接超时、认证失败等。在捕获到异常后,可以记录错误信息并采取相应的措施,比如重试连接或提示用户检查数据库配置。利用Python的logging
模块可以帮助记录错误信息,便于后续的故障排查。
如何在Python中安全地关闭数据库连接?
确保数据库连接被正确关闭是一个良好的编程习惯。使用with
语句可以自动管理连接的打开与关闭,避免连接泄露。例如,在使用sqlite3
库时,可以这样写:with sqlite3.connect('database.db') as conn:
。在with
块结束时,连接会自动关闭。如果不使用with
,务必在完成操作后调用connection.close()
方法来手动关闭连接,确保资源得到释放。
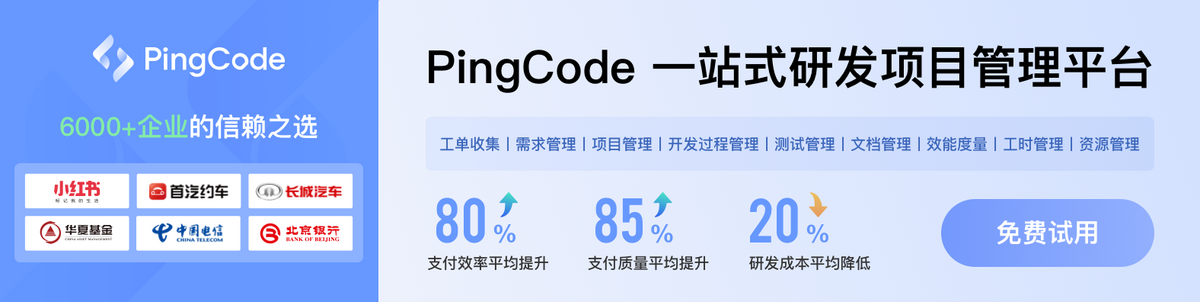