要监控某只股票的价格,可以使用Python编写脚本实现这一功能。主要方法包括使用API获取实时数据、利用定时任务定期检查价格、设置价格阈值并发送通知等。 其中,使用API获取实时数据是最为关键的一步。
一、获取实时股票数据
要监控某只股票的价格,首先需要从某个数据源获取实时股票数据。常用的数据源包括Yahoo Finance、Alpha Vantage、IEX Cloud等。Python中,有多个库可以方便地从这些数据源获取股票数据,如yfinance
、alpha_vantage
、iexfinance
等。
使用yfinance获取股票数据
yfinance
是一个非常流行的Python库,可以轻松地从Yahoo Finance获取股票数据。以下是一个简单的示例,展示如何使用yfinance
获取特定股票的最新价格:
import yfinance as yf
def get_stock_price(ticker):
stock = yf.Ticker(ticker)
price = stock.history(period='1d')['Close'][-1]
return price
ticker = 'AAPL'
print(f"The latest price of {ticker} is {get_stock_price(ticker)}")
在这个示例中,我们使用了yfinance
库的Ticker
类来获取特定股票(例如AAPL,即苹果公司)的历史数据,然后从中提取最新的收盘价。
使用Alpha Vantage获取股票数据
Alpha Vantage提供了一个免费的API来获取股票数据。要使用这个API,你需要先注册一个API密钥。以下是如何使用alpha_vantage
库获取实时股票数据的示例:
from alpha_vantage.timeseries import TimeSeries
def get_stock_price(ticker, api_key):
ts = TimeSeries(key=api_key)
data, _ = ts.get_quote_endpoint(symbol=ticker)
price = data['05. price']
return price
api_key = 'your_alpha_vantage_api_key'
ticker = 'AAPL'
print(f"The latest price of {ticker} is {get_stock_price(ticker, api_key)}")
使用IEX Cloud获取股票数据
IEX Cloud也是一个非常流行的股票数据源。以下是如何使用iexfinance
库获取实时股票数据的示例:
from iexfinance.stocks import Stock
def get_stock_price(ticker, api_key):
stock = Stock(ticker, token=api_key)
price = stock.get_price()
return price
api_key = 'your_iex_cloud_api_key'
ticker = 'AAPL'
print(f"The latest price of {ticker} is {get_stock_price(ticker, api_key)}")
二、定时任务
为了定期检查股票价格,可以使用Python中的schedule
库或time
模块来设置定时任务。
使用schedule库
schedule
库允许你以一种非常直观的方式安排定时任务。以下是一个示例,展示如何每分钟检查一次股票价格:
import schedule
import time
import yfinance as yf
def get_stock_price(ticker):
stock = yf.Ticker(ticker)
price = stock.history(period='1d')['Close'][-1]
print(f"The latest price of {ticker} is {price}")
ticker = 'AAPL'
def job():
get_stock_price(ticker)
schedule.every(1).minutes.do(job)
while True:
schedule.run_pending()
time.sleep(1)
使用time模块
time
模块提供了一种简单的方法来设置定时任务。以下是一个示例,展示如何每分钟检查一次股票价格:
import time
import yfinance as yf
def get_stock_price(ticker):
stock = yf.Ticker(ticker)
price = stock.history(period='1d')['Close'][-1]
print(f"The latest price of {ticker} is {price}")
ticker = 'AAPL'
while True:
get_stock_price(ticker)
time.sleep(60)
三、设置价格阈值并发送通知
为了在股票价格达到特定阈值时发送通知,可以使用Python中的if
语句来检查价格,并使用邮件、短信或其他通知服务来发送通知。
使用smtplib发送邮件通知
smtplib
是Python的一个内置库,可以用来发送邮件。以下是一个示例,展示如何在股票价格低于特定阈值时发送邮件通知:
import smtplib
from email.mime.text import MIMEText
import yfinance as yf
def get_stock_price(ticker):
stock = yf.Ticker(ticker)
price = stock.history(period='1d')['Close'][-1]
return price
def send_email(subject, body):
from_email = 'your_email@example.com'
to_email = 'recipient_email@example.com'
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login(from_email, 'your_email_password')
server.sendmail(from_email, to_email, msg.as_string())
ticker = 'AAPL'
threshold_price = 150
def job():
price = get_stock_price(ticker)
if price < threshold_price:
send_email('Stock Price Alert', f'The price of {ticker} has fallen below {threshold_price}. Current price: {price}')
schedule.every(1).minutes.do(job)
while True:
schedule.run_pending()
time.sleep(1)
使用Twilio发送短信通知
Twilio是一个非常流行的短信通知服务。以下是一个示例,展示如何在股票价格低于特定阈值时发送短信通知:
from twilio.rest import Client
import yfinance as yf
def get_stock_price(ticker):
stock = yf.Ticker(ticker)
price = stock.history(period='1d')['Close'][-1]
return price
def send_sms(body):
account_sid = 'your_twilio_account_sid'
auth_token = 'your_twilio_auth_token'
client = Client(account_sid, auth_token)
message = client.messages.create(
body=body,
from_='+1234567890',
to='+0987654321'
)
ticker = 'AAPL'
threshold_price = 150
def job():
price = get_stock_price(ticker)
if price < threshold_price:
send_sms(f'The price of {ticker} has fallen below {threshold_price}. Current price: {price}')
schedule.every(1).minutes.do(job)
while True:
schedule.run_pending()
time.sleep(1)
四、可视化股票价格
为了更直观地监控股票价格,可以使用Python中的matplotlib
或plotly
库来绘制股票价格的图表。
使用matplotlib绘制股票价格图表
matplotlib
是一个非常流行的绘图库,可以用来绘制各种图表。以下是一个示例,展示如何使用matplotlib
绘制股票价格的折线图:
import matplotlib.pyplot as plt
import yfinance as yf
def plot_stock_price(ticker):
stock = yf.Ticker(ticker)
hist = stock.history(period='1mo')
plt.plot(hist.index, hist['Close'])
plt.title(f'{ticker} Stock Price')
plt.xlabel('Date')
plt.ylabel('Price')
plt.show()
ticker = 'AAPL'
plot_stock_price(ticker)
使用plotly绘制股票价格图表
plotly
是一个非常强大的绘图库,可以用来创建交互式图表。以下是一个示例,展示如何使用plotly
绘制股票价格的折线图:
import plotly.graph_objects as go
import yfinance as yf
def plot_stock_price(ticker):
stock = yf.Ticker(ticker)
hist = stock.history(period='1mo')
fig = go.Figure(data=[go.Scatter(x=hist.index, y=hist['Close'], mode='lines', name=ticker)])
fig.update_layout(title=f'{ticker} Stock Price', xaxis_title='Date', yaxis_title='Price')
fig.show()
ticker = 'AAPL'
plot_stock_price(ticker)
五、综合示例
以下是一个综合示例,展示如何使用yfinance
获取股票数据、schedule
设置定时任务、smtplib
发送邮件通知,并使用matplotlib
绘制股票价格图表:
import schedule
import time
import yfinance as yf
import smtplib
from email.mime.text import MIMEText
import matplotlib.pyplot as plt
def get_stock_price(ticker):
stock = yf.Ticker(ticker)
price = stock.history(period='1d')['Close'][-1]
return price
def send_email(subject, body):
from_email = 'your_email@example.com'
to_email = 'recipient_email@example.com'
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login(from_email, 'your_email_password')
server.sendmail(from_email, to_email, msg.as_string())
def plot_stock_price(ticker):
stock = yf.Ticker(ticker)
hist = stock.history(period='1mo')
plt.plot(hist.index, hist['Close'])
plt.title(f'{ticker} Stock Price')
plt.xlabel('Date')
plt.ylabel('Price')
plt.show()
ticker = 'AAPL'
threshold_price = 150
def job():
price = get_stock_price(ticker)
if price < threshold_price:
send_email('Stock Price Alert', f'The price of {ticker} has fallen below {threshold_price}. Current price: {price}')
plot_stock_price(ticker)
schedule.every(1).minutes.do(job)
while True:
schedule.run_pending()
time.sleep(1)
通过上述步骤,可以实现对某只股票价格的实时监控,并在价格达到特定阈值时发送通知,同时还可以绘制股票价格的图表进行可视化分析。这些方法可以帮助你更好地监控和分析股票价格变化,以便在合适的时机做出投资决策。
相关问答FAQs:
如何使用Python获取实时股票价格?
要获取实时股票价格,可以使用一些流行的库,比如yfinance
和alpaca-trade-api
。这些库提供了方便的接口来抓取股票数据。安装这些库后,通过简单的几行代码就能获取特定股票的最新价格。例如,使用yfinance
库可以这样实现:
import yfinance as yf
stock = yf.Ticker("AAPL")
price = stock.history(period="1d")['Close'][0]
print(f"当前苹果股票价格为: {price}")
如何设置Python监控股票价格变化并发送通知?
您可以使用time
库来定期检查股票价格,并结合smptlib
或其他推送服务(如Slack或Telegram)发送通知。创建一个循环,每隔一定时间获取股票价格,并与之前的价格进行比较。如果发现显著变化,则触发通知。例如:
import time
import yfinance as yf
# 设置需要监控的股票
ticker = "AAPL"
previous_price = None
while True:
stock = yf.Ticker(ticker)
current_price = stock.history(period="1d")['Close'][0]
if previous_price and abs(current_price - previous_price) > 5: # 假设变化超过5美元
print(f"{ticker} 股票价格变化显著: {current_price}")
# 这里可以添加发送通知的代码
previous_price = current_price
time.sleep(60) # 每分钟检查一次
如何通过Python分析历史股票数据?
利用pandas
库可以轻松分析历史股票数据。您可以下载历史数据并执行各种分析,比如计算移动平均线、波动率等。使用yfinance
下载数据后,将其转换为DataFrame
格式,方便进行数据处理和可视化。例如:
import yfinance as yf
import pandas as pd
import matplotlib.pyplot as plt
data = yf.download("AAPL", start="2020-01-01", end="2023-01-01")
data['Close'].plot(title='苹果股票历史价格')
plt.show()
通过这种方式,您可以深入了解股票的价格趋势和市场动态。
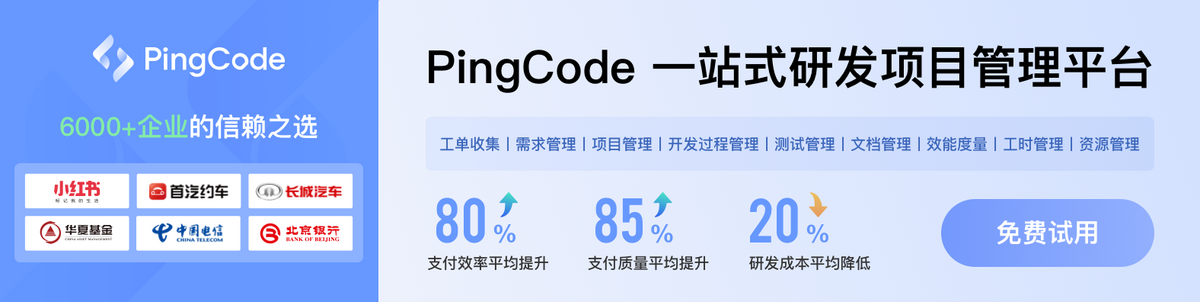