在Python中,自定义画笔形状的方法有多种。使用PIL库的ImageDraw模块、借助matplotlib库、使用Pygame库。通过这些库,我们可以创建图像并在其中自定义画笔形状。下面将详细介绍如何使用这三种方法中的一种,即PIL库的ImageDraw模块来自定义画笔形状。
PIL库的ImageDraw模块
PIL(Python Imaging Library)是一个强大的图像处理库,它的ImageDraw模块可以用来绘制图像,并支持自定义画笔形状。下面是一个使用PIL库自定义画笔形状的示例:
from PIL import Image, ImageDraw, ImageFilter
创建一个空白图像,模式为RGBA
image = Image.new("RGBA", (500, 500), (255, 255, 255, 0))
创建一个画笔形状
brush_size = 20
brush_shape = Image.new("L", (brush_size, brush_size), 0)
draw = ImageDraw.Draw(brush_shape)
draw.ellipse((0, 0, brush_size, brush_size), fill=255)
创建一个画布
canvas = ImageDraw.Draw(image)
自定义画笔形状
def custom_brush(draw, position, brush_shape):
mask = brush_shape.resize((brush_size, brush_size), Image.ANTIALIAS)
mask = mask.filter(ImageFilter.GaussianBlur(1))
draw.bitmap(position, brush_shape, mask=mask)
使用自定义画笔形状绘制图像
for i in range(50, 450, 50):
for j in range(50, 450, 50):
custom_brush(canvas, (i, j), brush_shape)
显示图像
image.show()
二、借助matplotlib库
matplotlib是一个广泛使用的绘图库,它也支持自定义画笔形状。下面是一个使用matplotlib库自定义画笔形状的示例:
import matplotlib.pyplot as plt
import numpy as np
自定义画笔形状
def custom_brush(x, y, size=20):
t = np.linspace(0, 2*np.pi, 100)
x_brush = x + size * np.sin(t)
y_brush = y + size * np.cos(t)
return x_brush, y_brush
创建图像
fig, ax = plt.subplots()
使用自定义画笔形状绘制图像
for i in range(50, 450, 50):
for j in range(50, 450, 50):
x_brush, y_brush = custom_brush(i, j, size=20)
ax.fill(x_brush, y_brush, "b")
显示图像
plt.show()
三、使用Pygame库
Pygame是一个专门用于游戏开发的库,它也支持自定义画笔形状。下面是一个使用Pygame库自定义画笔形状的示例:
import pygame
import sys
初始化Pygame
pygame.init()
创建窗口
window_size = (500, 500)
screen = pygame.display.set_mode(window_size)
设置背景颜色
background_color = (255, 255, 255)
screen.fill(background_color)
自定义画笔形状
brush_size = 20
brush_color = (0, 0, 255)
def custom_brush(surface, position, brush_size, brush_color):
pygame.draw.circle(surface, brush_color, position, brush_size)
主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.MOUSEMOTION:
mouse_position = pygame.mouse.get_pos()
custom_brush(screen, mouse_position, brush_size, brush_color)
pygame.display.flip()
pygame.quit()
sys.exit()
以上示例展示了如何使用PIL库、matplotlib库和Pygame库来自定义画笔形状。通过这些方法,你可以根据需要定制画笔形状,并在图像中进行绘制。
相关问答FAQs:
如何在Python中创建自定义画笔形状?
在Python中,可以使用多种图形库来创建自定义画笔形状。常用的库包括Pygame、Turtle和Matplotlib。以Pygame为例,可以通过加载自定义图片作为画笔形状,或者绘制一个多边形来实现。您只需定义画笔的形状,并在绘制时应用该形状即可。
自定义画笔形状对绘图效果有什么影响?
自定义画笔形状可以极大地丰富绘图效果,带来更具创意的作品。通过不同的形状,您可以实现各种艺术效果,比如使用星形画笔创建星星的效果,或者使用圆形画笔实现柔和的渐变效果。灵活运用自定义画笔形状,让您的绘图更加生动和个性化。
在使用自定义画笔时,有哪些常见的错误需要注意?
在使用自定义画笔时,常见的错误包括画笔形状未正确加载、坐标计算错误、以及在绘制时未正确更新画布。确保您的画笔形状文件路径正确,坐标系统与画布一致,并在每次绘制后调用更新函数,以确保绘图能够正确显示。注意调试和测试,可以避免这些常见问题。
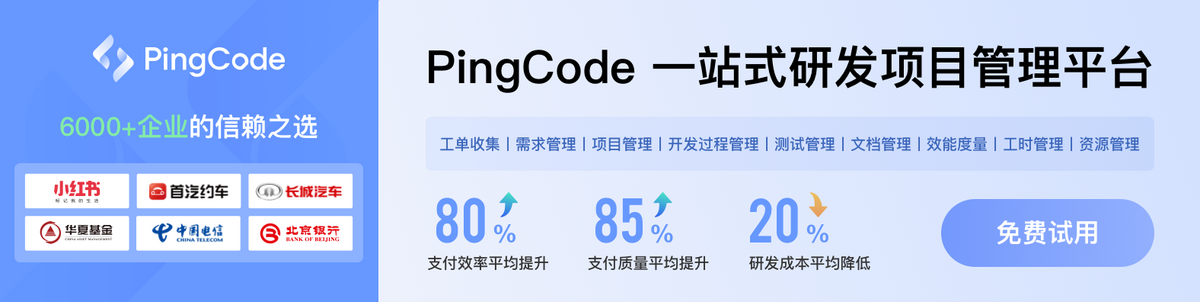