在Python中,比较两个列表的方法有很多,常见的有:使用==运算符、使用集合(set)、使用all()函数、使用列表推导式、使用循环、使用Counter模块。 这些方法各有优缺点,选择哪种方法取决于具体需求。例如,使用==运算符进行比较简单直接,但仅适用于比较元素顺序也相同的列表;使用集合进行比较则适用于比较无序列表;使用Counter模块可以比较列表中元素出现的次数。 接下来,我们将详细介绍这些方法。
一、使用==运算符
这是最简单直接的方法,适用于比较两个列表是否完全相同,包括顺序和内容。
list1 = [1, 2, 3, 4, 5]
list2 = [1, 2, 3, 4, 5]
if list1 == list2:
print("The lists are equal.")
else:
print("The lists are not equal.")
优点:
- 简单直接
- 代码简洁
缺点:
- 只能用于顺序和内容完全相同的列表比较
二、使用集合(set)
集合比较适用于无序列表的比较。通过将列表转换为集合,可以忽略元素的顺序,只比较元素的内容。
list1 = [1, 2, 3, 4, 5]
list2 = [5, 4, 3, 2, 1]
if set(list1) == set(list2):
print("The lists have the same elements.")
else:
print("The lists do not have the same elements.")
优点:
- 忽略顺序,比较内容
- 代码简洁
缺点:
- 无法比较元素的数量和顺序
三、使用all()函数
all()函数可以用于比较两个列表的内容和顺序,适用于逐一比较列表元素。
list1 = [1, 2, 3, 4, 5]
list2 = [1, 2, 3, 4, 5]
if all(a == b for a, b in zip(list1, list2)):
print("The lists are equal.")
else:
print("The lists are not equal.")
优点:
- 适用于比较内容和顺序
- 代码简洁
缺点:
- 只适用于长度相同的列表
四、使用列表推导式
列表推导式可以用于比较两个列表的内容和顺序,适用于逐一比较列表元素。
list1 = [1, 2, 3, 4, 5]
list2 = [1, 2, 3, 4, 5]
if [a == b for a, b in zip(list1, list2)]:
print("The lists are equal.")
else:
print("The lists are not equal.")
优点:
- 适用于比较内容和顺序
- 代码简洁
缺点:
- 只适用于长度相同的列表
五、使用循环
循环可以逐一比较两个列表的内容和顺序,适用于比较列表中的每个元素。
list1 = [1, 2, 3, 4, 5]
list2 = [1, 2, 3, 4, 5]
are_equal = True
for a, b in zip(list1, list2):
if a != b:
are_equal = False
break
if are_equal:
print("The lists are equal.")
else:
print("The lists are not equal.")
优点:
- 适用于比较内容和顺序
- 可以处理长度不同的列表
缺点:
- 代码相对较长
六、使用Counter模块
Counter模块可以用于比较两个列表中元素出现的次数,适用于比较无序列表。
from collections import Counter
list1 = [1, 2, 3, 4, 5]
list2 = [5, 4, 3, 2, 1]
if Counter(list1) == Counter(list2):
print("The lists have the same elements with the same frequency.")
else:
print("The lists do not have the same elements with the same frequency.")
优点:
- 忽略顺序,比较内容和数量
- 代码简洁
缺点:
- 无法比较元素的顺序
详细描述使用Counter模块
Counter模块是Python collections模块中的一个类,用于统计可哈希对象的出现次数。它是一个无序的容器类型,以字典的形式存储元素及其出现的次数。
使用Counter模块比较两个列表时,我们可以将两个列表转换为Counter对象,然后比较这两个Counter对象。这样可以比较两个列表中元素出现的次数,而忽略元素的顺序。
例如:
from collections import Counter
list1 = [1, 2, 3, 4, 5, 5]
list2 = [5, 4, 3, 2, 1, 5]
counter1 = Counter(list1)
counter2 = Counter(list2)
if counter1 == counter2:
print("The lists have the same elements with the same frequency.")
else:
print("The lists do not have the same elements with the same frequency.")
在上述例子中,counter1
和counter2
都是Counter对象,它们存储了列表中每个元素出现的次数。通过比较这两个Counter对象,我们可以确定两个列表中的元素是否相同以及它们的出现次数是否相同。
总结:
在Python中,比较两个列表的方法有很多,常见的有:使用==运算符、使用集合(set)、使用all()函数、使用列表推导式、使用循环、使用Counter模块。选择哪种方法取决于具体需求。例如,使用==运算符适用于比较顺序和内容完全相同的列表;使用集合适用于比较无序列表;使用Counter模块可以比较列表中元素出现的次数。希望本文能帮助你更好地理解和使用这些方法。
相关问答FAQs:
如何在Python中比较两个列表的内容是否相同?
在Python中,可以使用运算符==
来直接比较两个列表。如果两个列表包含相同的元素且顺序相同,则返回True
,否则返回False
。例如,list1 == list2
会比较这两个列表的内容。如果需要比较不考虑顺序,可以使用集合(set)来转换列表,再进行比较,如set(list1) == set(list2)
。
在Python中,如何找出两个列表之间的差异?
要找出两个列表之间的差异,可以使用集合操作。通过将两个列表转换为集合后,可以使用difference()
方法或运算符-
来获取在一个列表中而不在另一个列表中的元素。例如,set(list1).difference(set(list2))
将返回存在于list1
但不在list2
中的元素。相反,使用set(list2).difference(set(list1))
可以找出在list2
中而不在list1
中的元素。
如何使用Python的列表推导式比较两个列表并生成新的列表?
列表推导式提供了一种简洁的方式来比较两个列表并生成新的列表。例如,可以创建一个新的列表,包含存在于list1
但不在list2
中的元素,代码示例为:new_list = [item for item in list1 if item not in list2]
。这种方法不仅高效,而且可以根据具体需求进行自定义,生成不同的比较结果。
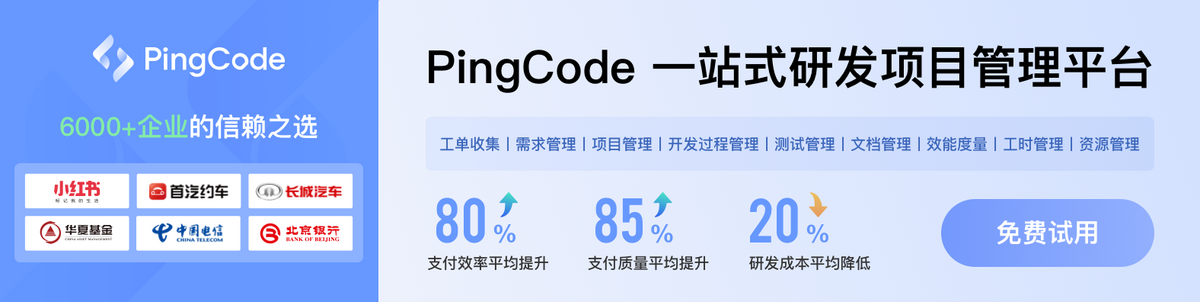