如何利用python统计英文文章词频
使用Python统计英文文章词频的方法有很多,常用的包括:使用collections模块的Counter类、使用nltk库进行文本处理、使用pandas库进行数据处理、使用正则表达式进行文本清洗。在这篇文章中,我们将详细探讨如何利用这些方法来统计英文文章的词频,并给出具体的代码示例。
一、使用collections模块的Counter类
collections模块是Python标准库中的一个强大模块,其中的Counter类可以非常方便地用来统计词频。以下是具体步骤:
1. 导入必要的模块
from collections import Counter
import re
2. 读取文本文件内容
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
3. 清洗文本数据
def clean_text(text):
text = text.lower()
text = re.sub(r'[^a-z\s]', '', text)
return text
4. 统计词频
def count_words(text):
words = text.split()
word_counts = Counter(words)
return word_counts
5. 将结果打印出来
file_path = 'example.txt'
text = read_file(file_path)
cleaned_text = clean_text(text)
word_counts = count_words(cleaned_text)
for word, count in word_counts.items():
print(f'{word}: {count}')
二、使用nltk库进行文本处理
nltk(Natural Language Toolkit)是一个强大的自然语言处理库,可以用来进行文本清洗、分词、词频统计等操作。以下是具体步骤:
1. 导入必要的模块
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
2. 下载必要的资源
nltk.download('punkt')
nltk.download('stopwords')
3. 读取文本文件内容
file_path = 'example.txt'
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
4. 清洗文本数据
text = text.lower()
text = re.sub(r'[^a-z\s]', '', text)
5. 分词并去除停用词
stop_words = set(stopwords.words('english'))
words = word_tokenize(text)
filtered_words = [word for word in words if word not in stop_words]
6. 统计词频
word_counts = Counter(filtered_words)
for word, count in word_counts.items():
print(f'{word}: {count}')
三、使用pandas库进行数据处理
pandas是一个强大的数据处理和分析库,可以用来处理文本数据并进行词频统计。以下是具体步骤:
1. 导入必要的模块
import pandas as pd
import re
from collections import Counter
2. 读取文本文件内容
file_path = 'example.txt'
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
3. 清洗文本数据
text = text.lower()
text = re.sub(r'[^a-z\s]', '', text)
4. 分词
words = text.split()
5. 统计词频并创建DataFrame
word_counts = Counter(words)
df = pd.DataFrame(word_counts.items(), columns=['Word', 'Frequency'])
6. 排序并打印结果
df = df.sort_values(by='Frequency', ascending=False)
print(df)
四、使用正则表达式进行文本清洗
正则表达式是处理文本数据的强大工具,可以用来清洗文本并进行分词。以下是具体步骤:
1. 导入必要的模块
import re
from collections import Counter
2. 读取文本文件内容
file_path = 'example.txt'
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
3. 清洗文本数据
text = text.lower()
text = re.sub(r'[^a-z\s]', '', text)
4. 分词并统计词频
words = text.split()
word_counts = Counter(words)
for word, count in word_counts.items():
print(f'{word}: {count}')
五、综合示例
我们可以综合使用上述方法来创建一个更完整的词频统计工具。以下是一个示例:
import re
from collections import Counter
import pandas as pd
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
下载必要的资源
nltk.download('punkt')
nltk.download('stopwords')
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
def clean_text(text):
text = text.lower()
text = re.sub(r'[^a-z\s]', '', text)
return text
def remove_stop_words(text):
stop_words = set(stopwords.words('english'))
words = word_tokenize(text)
filtered_words = [word for word in words if word not in stop_words]
return filtered_words
def count_words(words):
word_counts = Counter(words)
return word_counts
def main(file_path):
text = read_file(file_path)
cleaned_text = clean_text(text)
filtered_words = remove_stop_words(cleaned_text)
word_counts = count_words(filtered_words)
df = pd.DataFrame(word_counts.items(), columns=['Word', 'Frequency'])
df = df.sort_values(by='Frequency', ascending=False)
print(df)
file_path = 'example.txt'
main(file_path)
以上的综合示例展示了如何使用Python的标准库和第三方库来读取文本、清洗文本、分词、去除停用词和统计词频。通过这种方式,我们可以更方便地分析和处理文本数据。
六、扩展:可视化词频统计结果
为了更好地展示词频统计结果,我们可以使用matplotlib库将结果进行可视化。以下是具体步骤:
1. 导入必要的模块
import matplotlib.pyplot as plt
2. 绘制词频统计结果
def plot_word_counts(word_counts):
top_words = word_counts.most_common(10)
words, counts = zip(*top_words)
plt.figure(figsize=(10, 6))
plt.bar(words, counts)
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.title('Top 10 Words by Frequency')
plt.show()
3. 在main函数中调用绘制函数
def main(file_path):
text = read_file(file_path)
cleaned_text = clean_text(text)
filtered_words = remove_stop_words(cleaned_text)
word_counts = count_words(filtered_words)
df = pd.DataFrame(word_counts.items(), columns=['Word', 'Frequency'])
df = df.sort_values(by='Frequency', ascending=False)
print(df)
plot_word_counts(word_counts)
通过这种方式,我们可以更直观地展示词频统计结果,帮助我们更好地理解文本数据的特点和规律。
七、总结
在这篇文章中,我们详细介绍了如何利用Python统计英文文章的词频。我们探讨了使用collections模块的Counter类、nltk库、pandas库和正则表达式进行文本处理的方法,并给出了具体的代码示例。最后,我们还展示了如何使用matplotlib库将词频统计结果进行可视化。
通过这些方法,我们可以更方便地分析和处理文本数据,揭示文本数据中的隐藏信息和规律。希望这篇文章对你有所帮助,如果你有任何问题或建议,欢迎在下方留言。
相关问答FAQs:
如何使用Python来计算一篇英文文章中各个单词的出现频率?
使用Python计算词频通常需要借助于内置的字符串处理功能和一些额外的库,如collections中的Counter。首先,可以通过读取文本文件或直接输入字符串来获取文章内容。接着,利用split()方法将文本拆分为单词,最后使用Counter来统计每个单词的频率。示例代码如下:
from collections import Counter
import re
# 读取文件
with open('article.txt', 'r') as file:
text = file.read().lower() # 转为小写以避免重复
# 使用正则表达式去除标点符号
words = re.findall(r'\b\w+\b', text)
# 统计词频
word_counts = Counter(words)
# 输出结果
for word, count in word_counts.items():
print(f'{word}: {count}')
在统计词频时,如何处理常见的停用词?
停用词是指在文本分析中常常被忽略的单词,如“the”、“is”、“in”等。为了提高统计结果的相关性,可以在统计前将这些词从文本中移除。可以使用NLTK库提供的停用词列表,或者自定义一个停用词列表。示例中,您可以在统计前过滤掉这些词:
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word not in stop_words]
word_counts = Counter(filtered_words)
如何将词频统计结果可视化?
可视化词频统计结果能够帮助更直观地理解数据。可以使用Matplotlib或WordCloud库来实现。Matplotlib适合绘制条形图,而WordCloud可以生成词云图。以下是一个使用WordCloud库的示例:
from wordcloud import WordCloud
import matplotlib.pyplot as plt
wordcloud = WordCloud(width=800, height=400, background_color='white').generate_from_frequencies(word_counts)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
通过这种方式,您可以将词频数据以更加生动的形式呈现出来,增强对文本内容的理解。
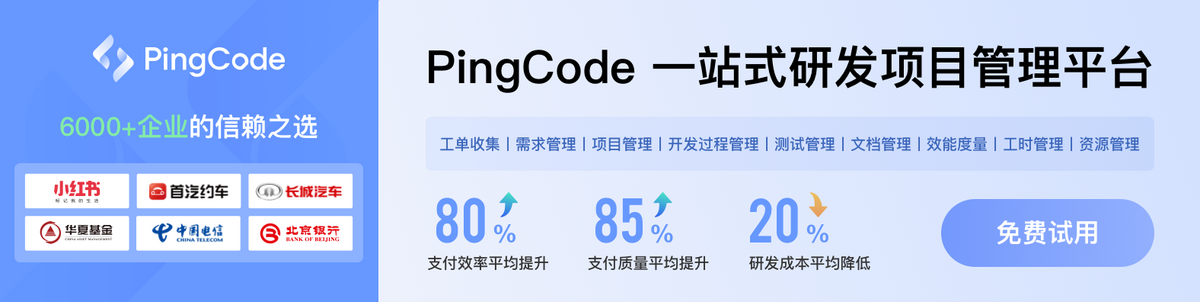