在Python中,查看键是否在字典中的方法有多种,最常用的方法包括:使用in
关键字、get()
方法、keys()
方法等。以下是详细说明:
- 使用
in
关键字: 这是最直接和推荐的方法,语法简单且高效。 - 使用
get()
方法: 此方法可以避免在键不存在时抛出异常,并且可以提供一个默认值。 - 使用
keys()
方法: 此方法可以获取字典所有键的视图,通过判断键是否存在于视图中来确定键的存在性。
使用in
关键字是最常用的方法,因其语法简洁且执行效率高。例如:
my_dict = {'a': 1, 'b': 2, 'c': 3}
if 'a' in my_dict:
print("Key 'a' is present in the dictionary.")
else:
print("Key 'a' is not present in the dictionary.")
一、使用in
关键字
in
关键字是一种直接且高效的方式来检查键是否存在于字典中。使用这种方法不仅语法简单,而且执行速度快。
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
key_to_check = 'age'
if key_to_check in my_dict:
print(f"Key '{key_to_check}' is present in the dictionary.")
else:
print(f"Key '{key_to_check}' is not present in the dictionary.")
在上面的例子中,通过if key_to_check in my_dict:
语句,可以直接判断key_to_check
是否存在于my_dict
字典中。这种方式的优势在于其直观性和高效性。
二、使用get()
方法
get()
方法不仅可以用于获取键对应的值,还可以用于检查键是否存在。与直接访问字典不同,get()
方法不会在键不存在时抛出异常,而是返回一个默认值(默认是None
)。
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
key_to_check = 'age'
value = my_dict.get(key_to_check, 'Key not found')
if value != 'Key not found':
print(f"Key '{key_to_check}' is present in the dictionary with value {value}.")
else:
print(f"Key '{key_to_check}' is not present in the dictionary.")
在上面的例子中,通过my_dict.get(key_to_check, 'Key not found')
方法,可以安全地检查key_to_check
是否存在于my_dict
字典中,并在不存在时返回'Key not found'
。
三、使用keys()
方法
使用keys()
方法可以获取字典所有键的视图,通过判断键是否存在于视图中来确定键的存在性。尽管这种方法不如in
关键字直接,但在某些情况下可能会有用。
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
key_to_check = 'age'
if key_to_check in my_dict.keys():
print(f"Key '{key_to_check}' is present in the dictionary.")
else:
print(f"Key '{key_to_check}' is not present in the dictionary.")
在上面的例子中,通过if key_to_check in my_dict.keys():
语句,可以判断key_to_check
是否存在于my_dict
字典中。不过,这种方法通常不如直接使用in
关键字高效,因为keys()
方法会创建一个包含字典所有键的视图。
四、使用异常处理
虽然不常见,但你也可以使用异常处理来检查键是否存在。这种方法通常用于需要在键不存在时执行特定的操作。
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
key_to_check = 'age'
try:
value = my_dict[key_to_check]
print(f"Key '{key_to_check}' is present in the dictionary with value {value}.")
except KeyError:
print(f"Key '{key_to_check}' is not present in the dictionary.")
在上面的例子中,通过try...except
块,可以捕获键不存在时抛出的KeyError
异常,并在异常处理块中执行特定的操作。
五、性能比较
在选择检查键是否存在的方法时,性能是一个重要的考虑因素。通常,使用in
关键字是最为高效的方法,因为其时间复杂度为O(1)。相比之下,get()
方法和keys()
方法的性能略低,特别是keys()
方法,因为它需要创建一个包含所有键的视图。
import timeit
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
Using 'in' keyword
in_time = timeit.timeit("'age' in my_dict", globals=globals(), number=1000000)
Using 'get()' method
get_time = timeit.timeit("my_dict.get('age')", globals=globals(), number=1000000)
Using 'keys()' method
keys_time = timeit.timeit("'age' in my_dict.keys()", globals=globals(), number=1000000)
print(f"'in' keyword time: {in_time}")
print(f"'get()' method time: {get_time}")
print(f"'keys()' method time: {keys_time}")
通过上述代码,可以对比不同方法在检查键是否存在时的性能差异。通常,in
关键字的执行时间最短,表示其性能最佳。
六、实际应用案例
在实际应用中,检查键是否存在于字典中是一个常见的操作。例如,在处理用户输入、数据清洗、API响应解析等场景中,经常需要检查键的存在性。
用户输入处理
在处理用户输入时,可能需要检查用户提供的键是否存在于预定义的字典中。
user_data = {'username': 'alice', 'email': 'alice@example.com'}
def process_user_data(data):
required_keys = ['username', 'email', 'age']
for key in required_keys:
if key not in data:
print(f"Missing required key: {key}")
return False
print("All required keys are present.")
return True
process_user_data(user_data)
在上面的例子中,通过if key not in data:
语句,可以检查用户提供的数据中是否包含所有必需的键。
数据清洗
在数据清洗过程中,可能需要检查某些键是否存在于数据字典中,以便进行相应的处理。
data = [{'name': 'Alice', 'age': 25}, {'name': 'Bob'}, {'name': 'Charlie', 'age': 30}]
def clean_data(data):
for record in data:
if 'age' not in record:
record['age'] = 'Unknown'
return data
cleaned_data = clean_data(data)
print(cleaned_data)
在上面的例子中,通过if 'age' not in record:
语句,可以检查每个记录中是否包含age
键,并在缺少该键时进行相应的处理。
API响应解析
在解析API响应时,可能需要检查响应数据中是否包含特定的键,以便提取相应的信息。
api_response = {'status': 'success', 'data': {'id': 123, 'name': 'Alice'}}
def parse_response(response):
if 'data' in response and 'id' in response['data']:
user_id = response['data']['id']
print(f"User ID: {user_id}")
else:
print("Missing required data in response.")
parse_response(api_response)
在上面的例子中,通过if 'data' in response and 'id' in response['data']:
语句,可以检查API响应数据中是否包含必需的键,并在缺少键时进行相应的处理。
总结
在Python中,查看键是否在字典中的方法有多种,最常用的方法包括使用in
关键字、get()
方法和keys()
方法。其中,使用in
关键字是最为推荐的方式,因其语法简洁且执行效率高。在实际应用中,根据具体需求选择合适的方法,可以提高代码的健壮性和可读性。
相关问答FAQs:
如何在Python中检查字典的键是否存在?
在Python中,可以使用in
关键字来检查一个键是否存在于字典中。例如,如果有一个字典my_dict
,你可以用if key in my_dict:
的方式来判断key
是否为字典的一个键。这种方法简洁明了,且效率高。
使用哪些方法可以安全地获取字典中的值?
获取字典中的值时,使用get()
方法是一个安全的选择。它允许你传入一个默认值,如果键不存在,则返回该默认值,避免了KeyError的产生。例如,my_dict.get(key, default_value)
可以确保即使键不存在,也不会引发错误。
字典的键可以是哪些类型?
在Python中,字典的键必须是不可变类型。这意味着可以使用字符串、数字和元组作为键,但不能使用列表、字典或其他可变类型。确保选择合适的键类型可以避免潜在的错误。
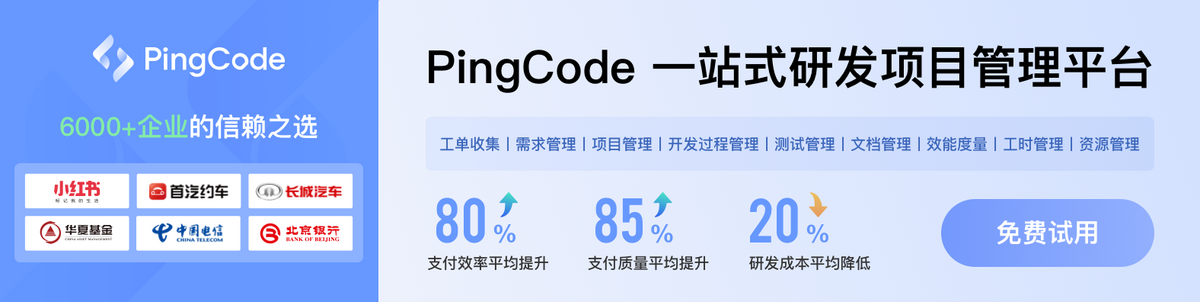