一、坐标在Python中如何写代码
在Python中写代码处理坐标主要有:使用列表、使用元组、使用类、使用numpy库、使用pandas库。 其中,使用类是最灵活和可扩展的方式,适用于复杂的坐标操作。接下来,我会详细介绍如何使用类来处理坐标。
二、使用列表和元组表示坐标
使用列表表示坐标
列表是Python中最常用的数据结构之一。用列表表示坐标的方法非常简单,通常用两个元素的列表来表示二维坐标,用三个元素的列表来表示三维坐标。
# 二维坐标
coordinate_2d = [3, 4]
三维坐标
coordinate_3d = [3, 4, 5]
列表表示坐标的优点是简单易用,但缺点是列表中的元素可以被随意修改,缺乏坐标的语义化表示。
使用元组表示坐标
元组与列表类似,但元组是不可变的,这意味着一旦创建,就不能修改其内容。使用元组表示坐标,可以确保坐标的值不会被意外修改。
# 二维坐标
coordinate_2d = (3, 4)
三维坐标
coordinate_3d = (3, 4, 5)
元组表示坐标比列表更安全,因为它们是不可变的,但同样缺乏语义化表示。
三、使用类表示坐标
使用类表示坐标可以为坐标提供更多的语义化表示和功能扩展。我们可以定义一个坐标类,提供必要的操作方法,如加法、减法、距离计算等。
定义二维坐标类
class Coordinate2D:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Coordinate2D(self.x + other.x, self.y + other.y)
def __sub__(self, other):
return Coordinate2D(self.x - other.x, self.y - other.y)
def distance(self, other):
return ((self.x - other.x) <strong> 2 + (self.y - other.y) </strong> 2) 0.5
def __repr__(self):
return f"Coordinate2D({self.x}, {self.y})"
示例
coord1 = Coordinate2D(3, 4)
coord2 = Coordinate2D(6, 8)
print(coord1 + coord2) # Coordinate2D(9, 12)
print(coord1.distance(coord2)) # 5.0
定义三维坐标类
class Coordinate3D:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __add__(self, other):
return Coordinate3D(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Coordinate3D(self.x - other.x, self.y - other.y, self.z - other.z)
def distance(self, other):
return ((self.x - other.x) <strong> 2 + (self.y - other.y) </strong> 2 + (self.z - other.z) <strong> 2) </strong> 0.5
def __repr__(self):
return f"Coordinate3D({self.x}, {self.y}, {self.z})"
示例
coord1 = Coordinate3D(3, 4, 5)
coord2 = Coordinate3D(6, 8, 10)
print(coord1 + coord2) # Coordinate3D(9, 12, 15)
print(coord1.distance(coord2)) # 7.0710678118654755
四、使用numpy库表示坐标
NumPy是一个强大的数值计算库,支持大规模的多维数组和矩阵运算。使用NumPy数组表示坐标,可以方便地进行向量化操作。
import numpy as np
二维坐标
coordinate_2d = np.array([3, 4])
coordinate_2d_2 = np.array([6, 8])
三维坐标
coordinate_3d = np.array([3, 4, 5])
coordinate_3d_2 = np.array([6, 8, 10])
向量加法
result_2d = coordinate_2d + coordinate_2d_2
result_3d = coordinate_3d + coordinate_3d_2
距离计算
distance_2d = np.linalg.norm(coordinate_2d - coordinate_2d_2)
distance_3d = np.linalg.norm(coordinate_3d - coordinate_3d_2)
print(result_2d) # [ 9 12]
print(result_3d) # [ 9 12 15]
print(distance_2d) # 5.0
print(distance_3d) # 7.0710678118654755
使用NumPy库表示坐标,能够利用其强大的数值计算功能,特别适用于大规模数据的处理。
五、使用pandas库表示坐标
Pandas是一个强大的数据处理和分析库,主要用于处理表格数据。我们可以使用Pandas的DataFrame来表示和操作一组坐标数据。
import pandas as pd
定义二维坐标数据
data_2d = {'x': [3, 6], 'y': [4, 8]}
定义三维坐标数据
data_3d = {'x': [3, 6], 'y': [4, 8], 'z': [5, 10]}
创建DataFrame
df_2d = pd.DataFrame(data_2d)
df_3d = pd.DataFrame(data_3d)
添加新坐标
df_2d.loc[len(df_2d)] = [9, 12]
df_3d.loc[len(df_3d)] = [9, 12, 15]
计算距离
df_2d['distance'] = ((df_2d['x'] - df_2d['x'].shift())<strong>2 + (df_2d['y'] - df_2d['y'].shift())</strong>2)0.5
df_3d['distance'] = ((df_3d['x'] - df_3d['x'].shift())<strong>2 + (df_3d['y'] - df_3d['y'].shift())</strong>2 + (df_3d['z'] - df_3d['z'].shift())<strong>2)</strong>0.5
print(df_2d)
print(df_3d)
使用Pandas库表示坐标,适用于需要对大规模坐标数据进行复杂分析和处理的场景。
六、坐标变换和应用
坐标变换
坐标变换在计算机图形学、机器人学和地理信息系统中非常常见。常见的坐标变换包括旋转、平移和缩放。
import numpy as np
def rotate_2d(coordinate, angle):
"""二维坐标旋转"""
rad = np.deg2rad(angle)
rotation_matrix = np.array([
[np.cos(rad), -np.sin(rad)],
[np.sin(rad), np.cos(rad)]
])
return np.dot(rotation_matrix, coordinate)
def translate_2d(coordinate, translation):
"""二维坐标平移"""
return coordinate + translation
def scale_2d(coordinate, scale_factor):
"""二维坐标缩放"""
return coordinate * scale_factor
示例
coordinate = np.array([3, 4])
rotated = rotate_2d(coordinate, 45)
translated = translate_2d(coordinate, np.array([1, 1]))
scaled = scale_2d(coordinate, 2)
print(rotated) # [ 0.70710678 4.94974747]
print(translated) # [4 5]
print(scaled) # [ 6 8]
坐标应用
坐标在很多实际应用中都有广泛的应用,如图形绘制、路径规划、地理信息系统等。
图形绘制
在计算机图形学中,坐标是绘制图形的基础。我们可以使用matplotlib库来绘制坐标图。
import matplotlib.pyplot as plt
定义二维坐标数据
x = [0, 1, 2, 3, 4]
y = [0, 1, 4, 9, 16]
绘制坐标图
plt.plot(x, y, marker='o')
plt.title('2D Coordinate Plot')
plt.xlabel('X')
plt.ylabel('Y')
plt.grid(True)
plt.show()
路径规划
在机器人学和自动驾驶中,坐标用于路径规划和运动控制。
class PathPlanner:
def __init__(self, start, goal):
self.start = np.array(start)
self.goal = np.array(goal)
self.path = [self.start]
def plan(self):
current = self.start
while np.linalg.norm(current - self.goal) > 1:
direction = (self.goal - current) / np.linalg.norm(self.goal - current)
current = current + direction
self.path.append(current)
self.path.append(self.goal)
def get_path(self):
return np.array(self.path)
示例
planner = PathPlanner([0, 0], [10, 10])
planner.plan()
path = planner.get_path()
print(path)
地理信息系统
在地理信息系统(GIS)中,坐标用于表示地理位置。
import geopandas as gpd
from shapely.geometry import Point
定义地理坐标数据
data = {'city': ['A', 'B', 'C'], 'latitude': [34.0522, 36.7783, 40.7128], 'longitude': [-118.2437, -119.4179, -74.0060]}
创建GeoDataFrame
df = gpd.GeoDataFrame(data, geometry=gpd.points_from_xy(data['longitude'], data['latitude']))
print(df)
七、结论
通过本文的介绍,我们了解了在Python中处理坐标的多种方法,包括使用列表、元组、类、numpy库和pandas库。每种方法都有其优缺点和适用场景。对于简单的坐标操作,可以使用列表或元组;对于复杂的坐标操作,可以定义类来表示坐标;对于大规模数据处理,可以使用numpy和pandas库。同时,我们还介绍了坐标变换和一些实际应用,如图形绘制、路径规划和地理信息系统。希望这些内容对你在Python中处理坐标有所帮助。
相关问答FAQs:
如何在Python中使用坐标进行绘图?
在Python中,您可以使用Matplotlib库来绘制坐标图。安装Matplotlib后,可以通过import matplotlib.pyplot as plt
来导入库。使用plt.plot(x, y)
可以绘制x和y坐标点的图形。为了显示图形,使用plt.show()
。您还可以添加标题、标签和图例,以增强图形的可读性。
Python中有哪些库可以处理坐标数据?
除了Matplotlib,Python中还有其他库可以处理坐标数据。例如,NumPy用于高效的数值计算,Pandas可以处理和分析数据集,而Seaborn则在Matplotlib的基础上提供了更高级的统计图形功能。这些库可以帮助您更好地操作和可视化坐标数据。
如何在Python中读取和处理坐标文件?
如果您有存储坐标的文件,如CSV格式,您可以使用Pandas的pd.read_csv()
函数读取文件。读取后,您可以轻松访问和处理坐标数据,例如筛选、排序或计算统计信息。处理完数据后,可以使用Matplotlib或其他可视化库来展示结果。
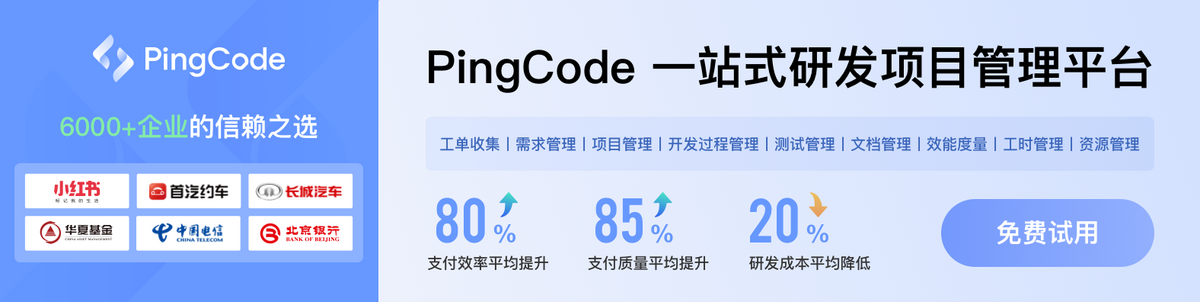