在Python中编辑用户名和密码的核心方法包括使用文件读写操作、数据库操作、哈希密码存储等。 在本文中,我们将详细介绍如何使用Python进行用户名和密码的编辑操作,包括文件操作、数据库操作和密码加密等内容。
一、文件操作
1、使用文件存储用户名和密码
文件存储是一种简单且直接的方式来管理用户名和密码。我们可以使用Python的内置文件操作函数来读写用户名和密码。
def save_credentials(username, password):
with open('credentials.txt', 'a') as file:
file.write(f"{username},{password}\n")
def load_credentials():
credentials = {}
with open('credentials.txt', 'r') as file:
for line in file:
username, password = line.strip().split(',')
credentials[username] = password
return credentials
def update_password(username, new_password):
credentials = load_credentials()
if username in credentials:
credentials[username] = new_password
with open('credentials.txt', 'w') as file:
for user, pwd in credentials.items():
file.write(f"{user},{pwd}\n")
print("Password updated successfully.")
else:
print("Username not found.")
在上述代码中,我们定义了三个函数:save_credentials
、load_credentials
和update_password
。第一个函数用于保存用户名和密码,第二个函数用于加载所有的用户名和密码,第三个函数用于更新指定用户的密码。
2、使用JSON格式存储用户名和密码
除了使用纯文本格式存储用户名和密码外,我们也可以使用JSON格式存储,这样可以更好地组织数据。
import json
def save_credentials_json(username, password):
credentials = load_credentials_json()
credentials[username] = password
with open('credentials.json', 'w') as file:
json.dump(credentials, file)
def load_credentials_json():
try:
with open('credentials.json', 'r') as file:
return json.load(file)
except FileNotFoundError:
return {}
def update_password_json(username, new_password):
credentials = load_credentials_json()
if username in credentials:
credentials[username] = new_password
with open('credentials.json', 'w') as file:
json.dump(credentials, file)
print("Password updated successfully.")
else:
print("Username not found.")
在此代码中,我们使用JSON格式保存和加载用户名和密码。save_credentials_json
用于保存用户名和密码,load_credentials_json
用于加载所有的用户名和密码,update_password_json
用于更新指定用户的密码。
二、数据库操作
1、使用SQLite数据库存储用户名和密码
SQLite是一种轻量级的数据库,非常适合嵌入到应用程序中。我们可以使用SQLite来存储和管理用户名和密码。
import sqlite3
def create_table():
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS users
(username TEXT PRIMARY KEY, password TEXT)''')
connection.commit()
connection.close()
def save_credentials_db(username, password):
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
cursor.execute("INSERT INTO users (username, password) VALUES (?, ?)", (username, password))
connection.commit()
connection.close()
def load_credentials_db():
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
cursor.execute("SELECT username, password FROM users")
credentials = cursor.fetchall()
connection.close()
return {username: password for username, password in credentials}
def update_password_db(username, new_password):
connection = sqlite3.connect('users.db')
cursor = connection.cursor()
cursor.execute("UPDATE users SET password = ? WHERE username = ?", (new_password, username))
connection.commit()
connection.close()
print("Password updated successfully.")
在上述代码中,我们使用SQLite数据库来存储用户名和密码。create_table
用于创建用户表,save_credentials_db
用于保存用户名和密码,load_credentials_db
用于加载所有的用户名和密码,update_password_db
用于更新指定用户的密码。
2、使用MySQL数据库存储用户名和密码
MySQL是一种功能强大的关系型数据库管理系统,适用于更大规模的应用程序。我们可以使用MySQL来存储和管理用户名和密码。
import mysql.connector
def create_table_mysql():
connection = mysql.connector.connect(user='your_username', password='your_password', host='127.0.0.1', database='your_database')
cursor = connection.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS users
(username VARCHAR(255) PRIMARY KEY, password VARCHAR(255))''')
connection.commit()
connection.close()
def save_credentials_mysql(username, password):
connection = mysql.connector.connect(user='your_username', password='your_password', host='127.0.0.1', database='your_database')
cursor = connection.cursor()
cursor.execute("INSERT INTO users (username, password) VALUES (%s, %s)", (username, password))
connection.commit()
connection.close()
def load_credentials_mysql():
connection = mysql.connector.connect(user='your_username', password='your_password', host='127.0.0.1', database='your_database')
cursor = connection.cursor()
cursor.execute("SELECT username, password FROM users")
credentials = cursor.fetchall()
connection.close()
return {username: password for username, password in credentials}
def update_password_mysql(username, new_password):
connection = mysql.connector.connect(user='your_username', password='your_password', host='127.0.0.1', database='your_database')
cursor = connection.cursor()
cursor.execute("UPDATE users SET password = %s WHERE username = %s", (new_password, username))
connection.commit()
connection.close()
print("Password updated successfully.")
在上述代码中,我们使用MySQL数据库来存储用户名和密码。create_table_mysql
用于创建用户表,save_credentials_mysql
用于保存用户名和密码,load_credentials_mysql
用于加载所有的用户名和密码,update_password_mysql
用于更新指定用户的密码。
三、密码加密
1、使用哈希算法加密密码
为了提高密码的安全性,我们可以使用哈希算法对密码进行加密存储。哈希算法可以将密码转换为一串固定长度的字符串,使得即使数据库被泄露,也难以恢复原始密码。
import hashlib
def hash_password(password):
return hashlib.sha256(password.encode()).hexdigest()
def save_credentials_hashed(username, password):
hashed_password = hash_password(password)
save_credentials(username, hashed_password)
def update_password_hashed(username, new_password):
hashed_password = hash_password(new_password)
update_password(username, hashed_password)
在此代码中,我们使用SHA-256哈希算法对密码进行加密存储。hash_password
用于对密码进行哈希,save_credentials_hashed
用于保存用户名和加密后的密码,update_password_hashed
用于更新指定用户的加密密码。
2、使用bcrypt加密密码
bcrypt是一种更为安全的加密算法,适用于存储用户密码。相比于SHA-256,bcrypt具有更高的计算复杂度,使得暴力破解更加困难。
import bcrypt
def hash_password_bcrypt(password):
return bcrypt.hashpw(password.encode(), bcrypt.gensalt())
def check_password_bcrypt(password, hashed):
return bcrypt.checkpw(password.encode(), hashed)
def save_credentials_bcrypt(username, password):
hashed_password = hash_password_bcrypt(password)
save_credentials(username, hashed_password.decode())
def update_password_bcrypt(username, new_password):
hashed_password = hash_password_bcrypt(new_password)
update_password(username, hashed_password.decode())
在此代码中,我们使用bcrypt对密码进行加密存储。hash_password_bcrypt
用于对密码进行bcrypt加密,check_password_bcrypt
用于验证密码,save_credentials_bcrypt
用于保存用户名和加密后的密码,update_password_bcrypt
用于更新指定用户的加密密码。
总结
本文详细介绍了如何在Python中编辑用户名和密码,包括使用文件操作、数据库操作和密码加密等内容。对于文件操作,我们介绍了使用纯文本和JSON格式存储用户名和密码的方法;对于数据库操作,我们介绍了使用SQLite和MySQL数据库存储用户名和密码的方法;对于密码加密,我们介绍了使用SHA-256和bcrypt加密密码的方法。这些方法均可以有效地帮助我们管理和保护用户的敏感信息。
相关问答FAQs:
如何在Python中安全地存储用户名和密码?
在Python中,可以使用加密库如bcrypt
或hashlib
来安全地存储用户名和密码。存储时应将密码进行哈希处理,而不是明文保存。使用bcrypt
可以确保密码的安全性,避免被黑客轻易破解。示例代码如下:
import bcrypt
# 哈希密码
password = b"my_secret_password"
hashed = bcrypt.hashpw(password, bcrypt.gensalt())
# 验证密码
if bcrypt.checkpw(password, hashed):
print("密码匹配")
else:
print("密码不匹配")
如何在Python应用程序中更新用户的登录信息?
在Python中更新用户的登录信息通常涉及数据库操作。首先,获取用户的当前信息,然后进行验证,最后更新数据库中的相应记录。使用sqlite3
或SQLAlchemy
等库,可以轻松实现这一过程。确保在更新时对输入进行验证和清理,以防止SQL注入等安全问题。
有哪些常见的Python库可以帮助我管理用户认证?
有多个Python库可以帮助管理用户认证,常用的包括Flask-Login
、Django Auth
和Authlib
。这些库提供了用户注册、登录、登出和会话管理的功能,简化了用户认证流程,同时也提供了一定的安全性。选择适合您项目需求的库,可以提升开发效率并确保安全性。
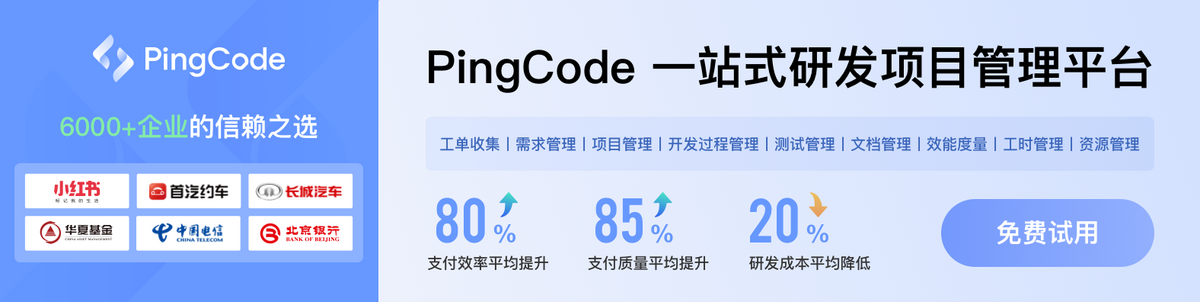