在Python编写用例判断按钮居中,首先需要确定按钮的居中依据,例如相对于窗口、父容器或其他元素。主要步骤包括:获取按钮位置、计算居中位置、比较实际位置与居中位置。
要详细描述其中一点,我们可以讨论“获取按钮位置”。在Python中,通常使用GUI库(如Tkinter、PyQt等)来创建和管理窗口及其组件。我们可以使用这些库提供的方法来获取按钮的实际位置和尺寸,以便进行进一步的计算和判断。
一、使用Tkinter编写用例判断按钮居中
Tkinter是Python的标准GUI库。以下是使用Tkinter编写用例判断按钮是否居中的详细步骤。
1. 创建窗口和按钮
首先,我们需要创建一个窗口并在其中放置一个按钮。
import tkinter as tk
创建主窗口
root = tk.Tk()
root.geometry("400x300") # 设置窗口大小
创建按钮
button = tk.Button(root, text="Click Me")
button.pack(pady=50) # 设置按钮的y轴填充
运行主循环
root.mainloop()
2. 获取按钮位置和尺寸
在Tkinter中,可以使用winfo_x()
和winfo_y()
方法获取按钮的位置,使用winfo_width()
和winfo_height()
方法获取按钮的尺寸。
button.update_idletasks() # 更新按钮的尺寸和位置信息
button_x = button.winfo_x()
button_y = button.winfo_y()
button_width = button.winfo_width()
button_height = button.winfo_height()
print(f"Button position: ({button_x}, {button_y})")
print(f"Button size: ({button_width}, {button_height})")
3. 计算窗口中心位置
窗口的中心位置可以通过获取窗口的宽度和高度来计算。
window_width = root.winfo_width()
window_height = root.winfo_height()
center_x = window_width // 2
center_y = window_height // 2
print(f"Window center: ({center_x}, {center_y})")
4. 判断按钮是否居中
通过比较按钮的位置与窗口中心位置,可以判断按钮是否居中。这里需要考虑按钮的尺寸。
button_center_x = button_x + button_width // 2
button_center_y = button_y + button_height // 2
is_centered_x = abs(button_center_x - center_x) <= 1 # 允许1个像素的误差
is_centered_y = abs(button_center_y - center_y) <= 1
is_centered = is_centered_x and is_centered_y
print(f"Is button centered: {is_centered}")
二、使用PyQt编写用例判断按钮居中
PyQt是另一个流行的Python GUI库。以下是使用PyQt编写用例判断按钮是否居中的详细步骤。
1. 创建窗口和按钮
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
app = QApplication([])
创建主窗口
window = QMainWindow()
window.setGeometry(100, 100, 400, 300) # 设置窗口大小和位置
创建按钮
button = QPushButton("Click Me", window)
button.resize(80, 30) # 设置按钮大小
button.move(160, 135) # 设置按钮位置
window.show()
app.exec_()
2. 获取按钮位置和尺寸
在PyQt中,可以使用geometry()
方法获取按钮的位置和尺寸。
button_geometry = button.geometry()
button_x = button_geometry.x()
button_y = button_geometry.y()
button_width = button_geometry.width()
button_height = button_geometry.height()
print(f"Button position: ({button_x}, {button_y})")
print(f"Button size: ({button_width}, {button_height})")
3. 计算窗口中心位置
窗口的中心位置可以通过获取窗口的宽度和高度来计算。
window_geometry = window.geometry()
window_width = window_geometry.width()
window_height = window_geometry.height()
center_x = window_width // 2
center_y = window_height // 2
print(f"Window center: ({center_x}, {center_y})")
4. 判断按钮是否居中
button_center_x = button_x + button_width // 2
button_center_y = button_y + button_height // 2
is_centered_x = abs(button_center_x - center_x) <= 1
is_centered_y = abs(button_center_y - center_y) <= 1
is_centered = is_centered_x and is_centered_y
print(f"Is button centered: {is_centered}")
三、用例的自动化测试
为了实现自动化测试,可以使用unittest模块来编写测试用例,并断言按钮是否居中。
1. Tkinter测试用例
import unittest
import tkinter as tk
class TestButtonCentered(unittest.TestCase):
def setUp(self):
self.root = tk.Tk()
self.root.geometry("400x300")
self.button = tk.Button(self.root, text="Click Me")
self.button.pack(pady=50)
self.button.update_idletasks()
def test_button_centered(self):
button_x = self.button.winfo_x()
button_y = self.button.winfo_y()
button_width = self.button.winfo_width()
button_height = self.button.winfo_height()
window_width = self.root.winfo_width()
window_height = self.root.winfo_height()
center_x = window_width // 2
center_y = window_height // 2
button_center_x = button_x + button_width // 2
button_center_y = button_y + button_height // 2
is_centered_x = abs(button_center_x - center_x) <= 1
is_centered_y = abs(button_center_y - center_y) <= 1
self.assertTrue(is_centered_x and is_centered_y)
if __name__ == "__main__":
unittest.main()
2. PyQt测试用例
import unittest
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
class TestButtonCentered(unittest.TestCase):
def setUp(self):
self.app = QApplication([])
self.window = QMainWindow()
self.window.setGeometry(100, 100, 400, 300)
self.button = QPushButton("Click Me", self.window)
self.button.resize(80, 30)
self.button.move(160, 135)
self.window.show()
def test_button_centered(self):
button_geometry = self.button.geometry()
button_x = button_geometry.x()
button_y = button_geometry.y()
button_width = button_geometry.width()
button_height = button_geometry.height()
window_geometry = self.window.geometry()
window_width = window_geometry.width()
window_height = window_geometry.height()
center_x = window_width // 2
center_y = window_height // 2
button_center_x = button_x + button_width // 2
button_center_y = button_y + button_height // 2
is_centered_x = abs(button_center_x - center_x) <= 1
is_centered_y = abs(button_center_y - center_y) <= 1
self.assertTrue(is_centered_x and is_centered_y)
if __name__ == "__main__":
unittest.main()
四、总结
在Python中,通过使用Tkinter或PyQt等GUI库,可以创建窗口和按钮,并获取按钮的位置和尺寸。通过计算窗口中心位置并比较按钮的位置,可以判断按钮是否居中。为了实现自动化测试,可以使用unittest模块编写测试用例,并断言按钮是否居中。上述方法可以帮助开发者有效地判断按钮是否在窗口或容器中居中,确保界面布局的美观性和一致性。
相关问答FAQs:
如何在Python中编写用例以验证按钮是否居中?
要验证按钮是否居中,可以使用Selenium库进行自动化测试。首先,您需要获取按钮的位置信息,然后与其父元素的宽度进行比较,从而确认按钮是否在水平方向上居中。以下是一个示例代码段,展示了如何实现这一点:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('您的测试页面URL')
button = driver.find_element_by_id('按钮的ID')
parent = button.find_element_by_xpath('..') # 获取按钮的父元素
# 获取按钮和父元素的宽度
button_width = button.size['width']
parent_width = parent.size['width']
# 计算按钮的左边距
button_location = button.location['x']
parent_location = parent.location['x']
# 判断按钮是否居中
if button_location - parent_location == (parent_width - button_width) / 2:
print("按钮居中")
else:
print("按钮未居中")
driver.quit()
使用Selenium进行界面测试时,如何定位按钮元素?
在Selenium中,可以通过多种方式定位按钮元素。常用的方法包括使用ID、XPath、CSS选择器等。例如,使用按钮的ID可以直接通过driver.find_element_by_id('按钮的ID')
进行定位;若使用XPath,可以使用driver.find_element_by_xpath('//button[text()="按钮文本"]')
。
如果按钮未居中,我该如何调整布局?
如果测试发现按钮未居中,可以通过调整CSS样式来修复。确保按钮的margin
设置为auto
,并且其父元素的display
属性设置为flex
或block
,这样可以确保按钮在父元素中居中显示。此外,检查是否有其他CSS样式影响了按钮的位置。
在编写UI测试用例时,如何确保稳定性和可靠性?
为了确保测试用例的稳定性,可以采取一些最佳实践。例如,使用显式等待来确保元素在操作之前已经加载完成;避免使用硬编码的等待时间,以提升测试的灵活性;将测试用例拆分为更小的功能模块,以便于维护和调试。
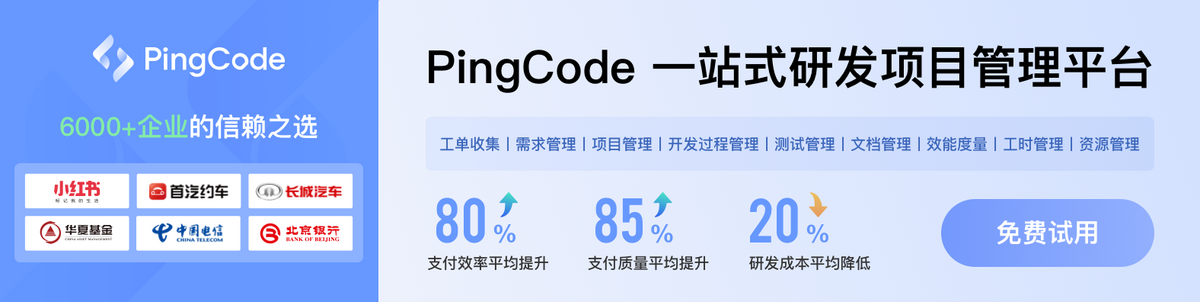