Python将变量转化为字符串的方法包括使用str()函数、repr()函数、格式化字符串(f-strings)等。 其中,最常用的方法是使用str()函数,将变量转化为字符串。str()函数的优点是简单直接,适用于大多数常见的变量类型,如整数、浮点数、布尔值等。下面将详细介绍这些方法及其适用场景。
一、str()函数
str()函数是Python中最常用的将变量转化为字符串的方法。它可以将整数、浮点数、布尔值、列表、元组和字典等数据类型转化为字符串。
# 将整数转化为字符串
num = 123
num_str = str(num)
print(num_str) # 输出:'123'
将浮点数转化为字符串
flt = 45.67
flt_str = str(flt)
print(flt_str) # 输出:'45.67'
将布尔值转化为字符串
boolean = True
bool_str = str(boolean)
print(bool_str) # 输出:'True'
将列表转化为字符串
lst = [1, 2, 3]
lst_str = str(lst)
print(lst_str) # 输出:'[1, 2, 3]'
将字典转化为字符串
dct = {'a': 1, 'b': 2}
dct_str = str(dct)
print(dct_str) # 输出:"{'a': 1, 'b': 2}"
str()函数的优点是它的使用非常简单,而且适用于大多数常见的数据类型。不过在某些情况下,例如自定义对象时,str()函数可能无法满足需求,此时可以考虑使用repr()函数或格式化字符串。
二、repr()函数
repr()函数与str()函数类似,用于将变量转化为字符串。不同的是,repr()函数返回的是一个更标准的字符串表示,通常用于调试或记录日志。它可以显示变量的类型信息,便于开发者了解变量的具体类型。
# 使用repr()函数将整数转化为字符串
num = 123
num_repr = repr(num)
print(num_repr) # 输出:'123'
使用repr()函数将浮点数转化为字符串
flt = 45.67
flt_repr = repr(flt)
print(flt_repr) # 输出:'45.67'
使用repr()函数将布尔值转化为字符串
boolean = True
bool_repr = repr(boolean)
print(bool_repr) # 输出:'True'
使用repr()函数将列表转化为字符串
lst = [1, 2, 3]
lst_repr = repr(lst)
print(lst_repr) # 输出:'[1, 2, 3]'
使用repr()函数将字典转化为字符串
dct = {'a': 1, 'b': 2}
dct_repr = repr(dct)
print(dct_repr) # 输出:"{'a': 1, 'b': 2}"
三、格式化字符串(f-strings)
格式化字符串(f-strings)是Python 3.6引入的一种新的字符串格式化方法。它可以让我们在字符串中嵌入变量,并将其自动转化为字符串。格式化字符串的优点是语法简洁、易读性高,适用于需要将多个变量嵌入到字符串中的场景。
# 使用格式化字符串将整数嵌入到字符串中
num = 123
num_fstr = f'The number is {num}'
print(num_fstr) # 输出:'The number is 123'
使用格式化字符串将浮点数嵌入到字符串中
flt = 45.67
flt_fstr = f'The float is {flt}'
print(flt_fstr) # 输出:'The float is 45.67'
使用格式化字符串将布尔值嵌入到字符串中
boolean = True
bool_fstr = f'The boolean value is {boolean}'
print(bool_fstr) # 输出:'The boolean value is True'
使用格式化字符串将列表嵌入到字符串中
lst = [1, 2, 3]
lst_fstr = f'The list is {lst}'
print(lst_fstr) # 输出:'The list is [1, 2, 3]'
使用格式化字符串将字典嵌入到字符串中
dct = {'a': 1, 'b': 2}
dct_fstr = f'The dictionary is {dct}'
print(dct_fstr) # 输出:'The dictionary is {'a': 1, 'b': 2}'
四、格式化方法
除了str()函数、repr()函数和格式化字符串之外,Python还提供了其他一些字符串格式化方法,例如旧式的百分号(%)格式化和str.format()方法。这些方法在某些情况下也可以用来将变量转化为字符串。
百分号(%)格式化
百分号(%)格式化是一种较旧的字符串格式化方法,它通过在字符串中使用百分号(%)占位符来嵌入变量。
# 使用百分号格式化将整数嵌入到字符串中
num = 123
num_percent = 'The number is %d' % num
print(num_percent) # 输出:'The number is 123'
使用百分号格式化将浮点数嵌入到字符串中
flt = 45.67
flt_percent = 'The float is %.2f' % flt
print(flt_percent) # 输出:'The float is 45.67'
使用百分号格式化将布尔值嵌入到字符串中
boolean = True
bool_percent = 'The boolean value is %s' % boolean
print(bool_percent) # 输出:'The boolean value is True'
使用百分号格式化将列表嵌入到字符串中
lst = [1, 2, 3]
lst_percent = 'The list is %s' % lst
print(lst_percent) # 输出:'The list is [1, 2, 3]'
使用百分号格式化将字典嵌入到字符串中
dct = {'a': 1, 'b': 2}
dct_percent = 'The dictionary is %s' % dct
print(dct_percent) # 输出:'The dictionary is {'a': 1, 'b': 2}'
str.format()方法
str.format()方法是Python 2.7和3.x版本中一种较新的字符串格式化方法。它通过在字符串中使用大括号({})占位符来嵌入变量,并通过format()方法将变量填充到占位符中。
# 使用str.format()方法将整数嵌入到字符串中
num = 123
num_format = 'The number is {}'.format(num)
print(num_format) # 输出:'The number is 123'
使用str.format()方法将浮点数嵌入到字符串中
flt = 45.67
flt_format = 'The float is {:.2f}'.format(flt)
print(flt_format) # 输出:'The float is 45.67'
使用str.format()方法将布尔值嵌入到字符串中
boolean = True
bool_format = 'The boolean value is {}'.format(boolean)
print(bool_format) # 输出:'The boolean value is True'
使用str.format()方法将列表嵌入到字符串中
lst = [1, 2, 3]
lst_format = 'The list is {}'.format(lst)
print(lst_format) # 输出:'The list is [1, 2, 3]'
使用str.format()方法将字典嵌入到字符串中
dct = {'a': 1, 'b': 2}
dct_format = 'The dictionary is {}'.format(dct)
print(dct_format) # 输出:'The dictionary is {'a': 1, 'b': 2}'
五、自定义对象转化为字符串
当我们需要将自定义对象转化为字符串时,可以通过重写类的__str__()和__repr__()方法来实现。str()方法用于定义对象的用户友好字符串表示,repr()方法用于定义对象的标准字符串表示。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f'Person(name={self.name}, age={self.age})'
def __repr__(self):
return f'Person(name={self.name}, age={self.age})'
创建一个Person对象
person = Person('Alice', 30)
使用str()函数将Person对象转化为字符串
person_str = str(person)
print(person_str) # 输出:'Person(name=Alice, age=30)'
使用repr()函数将Person对象转化为字符串
person_repr = repr(person)
print(person_repr) # 输出:'Person(name=Alice, age=30)'
通过重写__str__()和__repr__()方法,我们可以自定义对象的字符串表示形式,从而方便地将自定义对象转化为字符串。
六、总结
Python提供了多种将变量转化为字符串的方法,包括str()函数、repr()函数、格式化字符串(f-strings)、百分号(%)格式化、str.format()方法等。这些方法各有优缺点,适用于不同的场景。str()函数是最常用的方法,适用于大多数常见的数据类型。repr()函数适用于需要标准字符串表示的场景,便于调试和记录日志。格式化字符串(f-strings)语法简洁、易读性高,适用于需要将多个变量嵌入到字符串中的场景。百分号(%)格式化和str.format()方法也是常用的字符串格式化方法,适用于不同的格式化需求。在处理自定义对象时,可以通过重写类的__str__()和__repr__()方法来实现对象的字符串表示。
总之,根据具体的需求选择合适的字符串转化方法,可以提高代码的可读性和可维护性。在实际开发中,建议优先使用格式化字符串(f-strings),因为它语法简洁、易读性高,是Python 3.6及以上版本中推荐的字符串格式化方法。对于需要兼容旧版本Python的项目,可以考虑使用str.format()方法或百分号(%)格式化方法。
相关问答FAQs:
如何在Python中将数字变量转换为字符串?
在Python中,可以使用内置的str()
函数将数字变量转换为字符串。例如,如果你有一个数字变量num = 123
,你可以通过string_num = str(num)
将其转换为字符串。这样,string_num
的值就是'123'
。
Python中的其他字符串转换方法有哪些?
除了使用str()
函数,Python还提供了其他方法进行字符串转换。例如,使用格式化字符串可以将变量插入到字符串中,如formatted_string = f"The number is {num}"
。此外,使用format()
方法也可以实现类似效果,比如formatted_string = "The number is {}".format(num)
。
在Python中如何处理非字符串变量的转换?
对于非字符串类型的变量,如列表、字典等,可以使用json
模块中的dumps()
方法进行转换。比如,import json
后,可以通过json_string = json.dumps(my_list)
将列表转换为字符串。此方法不仅适用于列表,还可以处理字典等复杂数据结构,生成JSON格式的字符串。
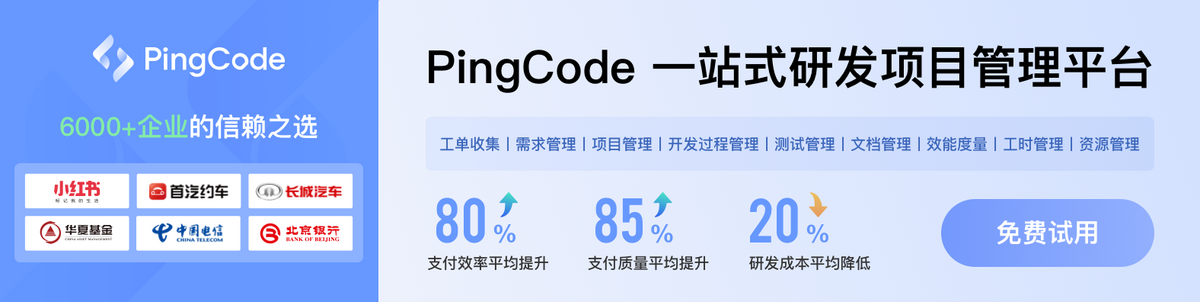