在Python中将图片存入数据库的主要方法有:将图片转换为二进制数据、使用合适的数据库模块连接数据库、编写SQL语句进行数据插入。 在这三者中,将图片转换为二进制数据是最关键的一步,因为数据库一般无法直接存储图像文件,需要将其转换成二进制格式。本文将详细介绍如何在Python中实现这一过程,并给出实际代码示例。
一、将图片转换为二进制数据
在Python中,可以使用open
函数读取文件并将其转换为二进制数据。具体步骤如下:
- 使用
open
函数以二进制模式打开图片文件。 - 使用
read
方法读取文件内容。 - 将读取的内容赋值给一个变量,作为二进制数据。
def convert_image_to_binary(file_path):
with open(file_path, 'rb') as file:
binary_data = file.read()
return binary_data
二、连接数据库
在Python中可以使用多种数据库模块,如sqlite3
、pymysql
、psycopg2
等。本文以sqlite3
为例进行说明。
- 导入
sqlite3
模块。 - 使用
connect
方法连接到数据库。 - 使用
cursor
方法创建一个游标对象。
import sqlite3
def connect_to_database(db_name):
connection = sqlite3.connect(db_name)
cursor = connection.cursor()
return connection, cursor
三、编写SQL语句并插入数据
编写SQL语句插入二进制数据,具体步骤如下:
- 编写
INSERT
语句,使用占位符?
来表示二进制数据的位置。 - 使用
execute
方法执行SQL语句,将二进制数据插入数据库。 - 提交事务并关闭连接。
def insert_image_to_database(cursor, table_name, image_data):
sql = f"INSERT INTO {table_name} (image_column) VALUES (?)"
cursor.execute(sql, (image_data,))
四、完整示例代码
以下是将上述步骤整合到一起的完整示例代码:
import sqlite3
def convert_image_to_binary(file_path):
with open(file_path, 'rb') as file:
binary_data = file.read()
return binary_data
def connect_to_database(db_name):
connection = sqlite3.connect(db_name)
cursor = connection.cursor()
return connection, cursor
def create_table(cursor, table_name):
cursor.execute(f"CREATE TABLE IF NOT EXISTS {table_name} (id INTEGER PRIMARY KEY, image_column BLOB)")
def insert_image_to_database(cursor, table_name, image_data):
sql = f"INSERT INTO {table_name} (image_column) VALUES (?)"
cursor.execute(sql, (image_data,))
def main():
db_name = 'image_database.db'
table_name = 'images'
image_path = 'path_to_image.jpg'
connection, cursor = connect_to_database(db_name)
create_table(cursor, table_name)
image_data = convert_image_to_binary(image_path)
insert_image_to_database(cursor, table_name, image_data)
connection.commit()
connection.close()
if __name__ == "__main__":
main()
五、错误处理与优化
在实际应用中,还需要考虑错误处理和代码优化。例如:
- 错误处理:在数据库连接、文件读取等过程中可能会发生错误,应该添加适当的错误处理机制。
- 代码优化:可以将常用操作封装成函数,减少代码冗余。
以下是优化后的代码示例:
import sqlite3
def convert_image_to_binary(file_path):
try:
with open(file_path, 'rb') as file:
binary_data = file.read()
return binary_data
except Exception as e:
print(f"Error converting image to binary: {e}")
return None
def connect_to_database(db_name):
try:
connection = sqlite3.connect(db_name)
cursor = connection.cursor()
return connection, cursor
except Exception as e:
print(f"Error connecting to database: {e}")
return None, None
def create_table(cursor, table_name):
try:
cursor.execute(f"CREATE TABLE IF NOT EXISTS {table_name} (id INTEGER PRIMARY KEY, image_column BLOB)")
except Exception as e:
print(f"Error creating table: {e}")
def insert_image_to_database(cursor, table_name, image_data):
try:
sql = f"INSERT INTO {table_name} (image_column) VALUES (?)"
cursor.execute(sql, (image_data,))
except Exception as e:
print(f"Error inserting image to database: {e}")
def main():
db_name = 'image_database.db'
table_name = 'images'
image_path = 'path_to_image.jpg'
connection, cursor = connect_to_database(db_name)
if connection and cursor:
create_table(cursor, table_name)
image_data = convert_image_to_binary(image_path)
if image_data:
insert_image_to_database(cursor, table_name, image_data)
connection.commit()
connection.close()
if __name__ == "__main__":
main()
六、总结
本文详细介绍了如何在Python中将图片存入数据库的实现方法,包括将图片转换为二进制数据、连接数据库、编写SQL语句并插入数据等步骤。通过实际代码示例,展示了如何将这些步骤整合到一起,实现图片的存储。同时,还对代码进行了优化,添加了错误处理机制。希望本文对你有所帮助,能够顺利实现图片的存储。
相关问答FAQs:
如何在Python中将图片转换为可存储的格式?
在将图片存入数据库之前,首先需要将其转换为合适的格式。通常,使用Python的PIL(Pillow)库来处理图像,可以将图片打开并转换为字节流。可以使用io.BytesIO
将图像保存为字节数据。例如:
from PIL import Image
import io
image = Image.open('path_to_image.jpg')
byte_array = io.BytesIO()
image.save(byte_array, format='JPEG')
byte_data = byte_array.getvalue()
在数据库中存储图片时,应该选择哪种数据类型?
在数据库中存储图片时,通常使用BLOB(Binary Large Object)数据类型。这种类型允许存储大量的二进制数据,非常适合存放图像文件。此外,还可以考虑将图片的元数据(如文件名、类型和大小)存储在其他字段中,以便后续检索和管理。
如何在Python中从数据库中检索并显示图片?
从数据库中检索存储的图片时,首先需要获取BLOB数据并将其转换回图像格式。可以使用PIL
库将字节数据转换为图像。示例代码如下:
import sqlite3
from PIL import Image
import io
# 假设已经连接到数据库并获取了图像字节数据
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
cursor.execute("SELECT image_column FROM images WHERE id = ?", (image_id,))
image_data = cursor.fetchone()[0]
# 将字节数据转换为图像
image = Image.open(io.BytesIO(image_data))
image.show()
这段代码将检索特定ID的图像并显示出来。
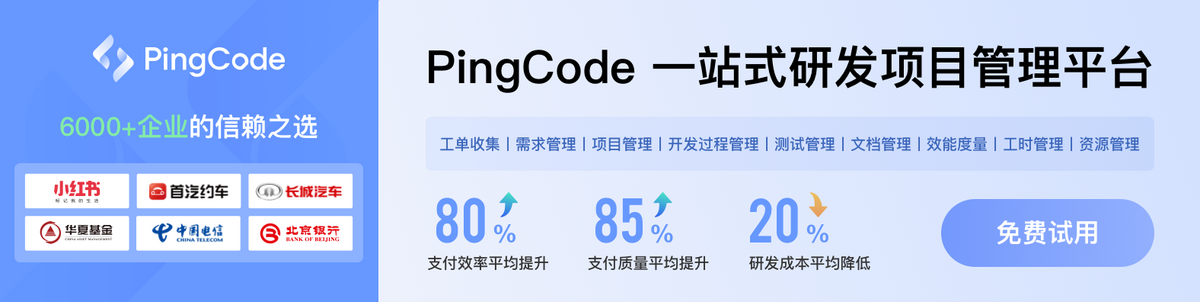