Python对文件进行复制并批量命名的具体操作方法包括:使用shutil库进行文件复制、使用os库进行文件命名、批量处理文件等。我们将详细介绍文件复制、命名和批量处理的具体步骤。 其中,通过使用shutil库进行文件复制是最常用的方法之一。接下来,我们会展开详细描述如何使用Python完成这些操作。
一、文件复制
使用Python进行文件复制最常用的库是shutil。shutil库提供了多种文件操作功能,包括复制文件、复制文件夹等。我们可以使用shutil.copy()函数来完成文件的复制工作。
import shutil
复制文件
shutil.copy('source_file.txt', 'destination_file.txt')
在上述代码中,shutil.copy()函数将source_file.txt文件复制到destination_file.txt位置。如果目标文件已经存在,它将被覆盖。shutil.copy()函数不仅适用于文件复制,还可以用于复制文件的权限、元数据等。
二、文件命名
在文件复制的基础上,我们可以通过os库对文件进行重命名。os库提供了丰富的文件操作功能,包括文件重命名、删除文件、创建目录等。
import os
重命名文件
os.rename('old_name.txt', 'new_name.txt')
在上述代码中,os.rename()函数将old_name.txt文件重命名为new_name.txt文件。如果目标文件已经存在,将会引发FileExistsError错误。因此,我们需要确保目标文件名是唯一的。
三、批量处理文件
为了实现批量处理文件的功能,我们可以结合os库和循环结构来遍历文件夹中的所有文件,并对每个文件进行复制和命名操作。
import os
import shutil
定义源文件夹和目标文件夹
source_folder = 'source_folder'
destination_folder = 'destination_folder'
创建目标文件夹
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
遍历源文件夹中的所有文件
for filename in os.listdir(source_folder):
# 构建源文件路径和目标文件路径
source_file = os.path.join(source_folder, filename)
destination_file = os.path.join(destination_folder, f'copy_{filename}')
# 复制文件并重命名
shutil.copy(source_file, destination_file)
在上述代码中,我们首先定义了源文件夹和目标文件夹,然后遍历源文件夹中的所有文件,并将每个文件复制到目标文件夹中,同时在文件名前添加'copy_'前缀,以实现批量命名的功能。
四、扩展功能:文件筛选和文件计数
在实际应用中,我们可能需要对特定类型的文件进行复制和命名操作,或者需要统计文件的数量。我们可以通过扩展代码来实现这些功能。
- 文件筛选
# 只处理特定类型的文件,例如txt文件
file_extension = '.txt'
for filename in os.listdir(source_folder):
if filename.endswith(file_extension):
source_file = os.path.join(source_folder, filename)
destination_file = os.path.join(destination_folder, f'copy_{filename}')
shutil.copy(source_file, destination_file)
在上述代码中,我们通过判断文件名是否以特定扩展名结尾来筛选文件,只处理符合条件的文件。
- 文件计数
file_count = 0
for filename in os.listdir(source_folder):
if filename.endswith(file_extension):
source_file = os.path.join(source_folder, filename)
destination_file = os.path.join(destination_folder, f'copy_{filename}')
shutil.copy(source_file, destination_file)
file_count += 1
print(f'共处理了{file_count}个文件')
在上述代码中,我们通过计数器变量file_count来统计处理的文件数量,并在处理完成后输出文件数量。
五、异常处理
在文件操作过程中,可能会遇到各种异常情况,例如文件不存在、权限不足等。我们可以通过添加异常处理代码来提高程序的健壮性。
try:
shutil.copy(source_file, destination_file)
except FileNotFoundError:
print(f'文件未找到:{source_file}')
except PermissionError:
print(f'权限不足:{source_file}')
except Exception as e:
print(f'发生错误:{e}')
在上述代码中,我们通过try…except结构捕获并处理可能的异常情况,并输出相应的错误信息。
六、综合示例
下面是一个综合示例,结合文件复制、命名、批量处理、文件筛选、文件计数和异常处理等功能,展示如何使用Python进行文件操作。
import os
import shutil
def copy_and_rename_files(source_folder, destination_folder, file_extension='.txt'):
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
file_count = 0
for filename in os.listdir(source_folder):
if filename.endswith(file_extension):
source_file = os.path.join(source_folder, filename)
destination_file = os.path.join(destination_folder, f'copy_{filename}')
try:
shutil.copy(source_file, destination_file)
file_count += 1
except FileNotFoundError:
print(f'文件未找到:{source_file}')
except PermissionError:
print(f'权限不足:{source_file}')
except Exception as e:
print(f'发生错误:{e}')
print(f'共处理了{file_count}个文件')
调用函数进行文件复制和命名操作
source_folder = 'source_folder'
destination_folder = 'destination_folder'
copy_and_rename_files(source_folder, destination_folder)
在上述代码中,我们将文件复制、命名、批量处理、文件筛选、文件计数和异常处理等功能封装在一个函数中,并通过调用函数来实现文件操作。
通过以上方法,我们可以使用Python轻松实现文件的复制和批量命名操作,并根据需要扩展更多功能,以满足实际应用的需求。Python提供了丰富的库和工具,使得文件操作变得简单高效。
相关问答FAQs:
如何使用Python复制文件并进行批量命名?
使用Python进行文件复制时,可以使用shutil
库中的copy
或copy2
函数来实现。要进行批量命名,可以通过循环遍历文件名并添加后缀或前缀来实现。以下是一个简单的示例代码:
import shutil
import os
source_file = 'source.txt'
for i in range(1, 6):
new_file_name = f'copy_{i}.txt'
shutil.copy(source_file, new_file_name)
这个代码将source.txt
复制五次,并分别命名为copy_1.txt
到copy_5.txt
。
在Python中如何处理文件复制时的错误?
在进行文件复制时,可能会遇到一些错误,例如源文件不存在或目标路径无写入权限。为此,可以使用try...except
语句来捕获异常。示例代码如下:
import shutil
import os
source_file = 'source.txt'
try:
shutil.copy(source_file, 'copy.txt')
except FileNotFoundError:
print("源文件不存在,请检查文件路径。")
except PermissionError:
print("没有权限写入目标文件,请检查权限设置。")
这样可以确保在复制文件时能够妥善处理潜在的错误。
如何在Python中批量复制文件到不同的目录?
要将文件批量复制到不同的目录,可以结合使用os
库和shutil
库。可以通过创建一个目标目录的列表,并在循环中将源文件复制到每个目标目录。示例代码如下:
import shutil
import os
source_file = 'source.txt'
target_dirs = ['dir1', 'dir2', 'dir3']
for dir in target_dirs:
os.makedirs(dir, exist_ok=True) # 确保目标目录存在
shutil.copy(source_file, os.path.join(dir, 'copy.txt'))
这段代码会将source.txt
复制到dir1
、dir2
和dir3
目录中,命名为copy.txt
。
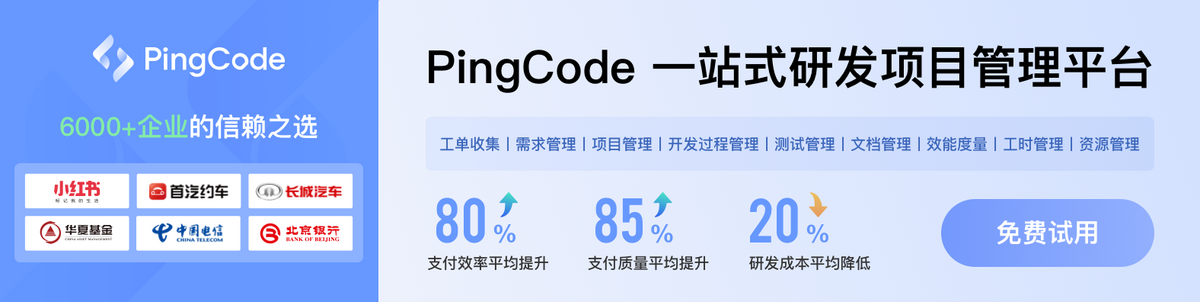