Python字符串匹配字符串的方法有多种,包括使用in
关键字、str.find()
方法、str.index()
方法、正则表达式、和str.startswith()
及str.endswith()
方法。其中,使用正则表达式可以实现复杂的字符串匹配。在Python中,可以通过re
模块来使用正则表达式。下面详细介绍这些方法。
一、使用in
关键字
使用in
关键字是最简单和直接的字符串匹配方法。它可以检查一个字符串是否包含另一个字符串。
main_string = "Hello, welcome to the world of Python"
substring = "welcome"
if substring in main_string:
print("Substring found!")
else:
print("Substring not found.")
in
关键字返回一个布尔值,表示是否找到了子字符串。
二、使用str.find()
方法
str.find()
方法返回子字符串在字符串中的最低索引,如果找不到则返回-1。
main_string = "Hello, welcome to the world of Python"
substring = "welcome"
index = main_string.find(substring)
if index != -1:
print(f"Substring found at index {index}")
else:
print("Substring not found.")
三、使用str.index()
方法
str.index()
方法与str.find()
类似,但如果找不到子字符串,会引发ValueError
异常。
main_string = "Hello, welcome to the world of Python"
substring = "welcome"
try:
index = main_string.index(substring)
print(f"Substring found at index {index}")
except ValueError:
print("Substring not found.")
四、使用正则表达式
正则表达式是进行复杂模式匹配的强大工具。在Python中,可以使用re
模块来处理正则表达式。
import re
main_string = "Hello, welcome to the world of Python"
pattern = r"welcome"
if re.search(pattern, main_string):
print("Pattern found!")
else:
print("Pattern not found.")
re.search()
方法返回一个匹配对象,如果没有找到匹配则返回None
。
五、使用str.startswith()
和str.endswith()
方法
这两个方法分别用于检查字符串是否以特定子字符串开头或结尾。
main_string = "Hello, welcome to the world of Python"
if main_string.startswith("Hello"):
print("String starts with 'Hello'")
if main_string.endswith("Python"):
print("String ends with 'Python'")
详细描述正则表达式
正则表达式(Regular Expression,简称regex或regexp)是一种用于匹配字符串的模式。Python的re
模块提供了强大的正则表达式处理能力。正则表达式可以用来检查字符串是否符合某种模式,提取字符串的特定部分,替换字符串中的内容等。
正则表达式的基本语法
- 字符类:使用方括号
[]
定义一个字符类。例如,[a-z]
表示所有小写字母,[0-9]
表示所有数字。 - 预定义字符类:一些常用的字符类有预定义的简写形式。例如,
\d
表示数字,\w
表示字母数字字符,\s
表示空白字符。 - 量词:量词用来指定字符出现的次数。例如,
*
表示零次或多次,+
表示一次或多次,?
表示零次或一次,{m,n}
表示至少m次,至多n次。 - 边界匹配:
^
表示字符串的开始,$
表示字符串的结束。 - 分组和捕获:使用圆括号
()
将部分模式分组,并捕获匹配的内容。可以使用\1
、\2
等来引用捕获的内容。
例子:匹配电子邮件地址
下面是一个简单的正则表达式,用于匹配电子邮件地址:
import re
pattern = r"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$"
email = "example@example.com"
if re.match(pattern, email):
print("Valid email address")
else:
print("Invalid email address")
在这个例子中,^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$
是一个正则表达式模式,用于匹配电子邮件地址。
正则表达式在实际应用中的例子
1、提取电话号码
假设你有一段文本,想要从中提取所有的电话号码,可以使用正则表达式:
import re
text = "Contact us at 123-456-7890 or 987-654-3210"
pattern = r"\d{3}-\d{3}-\d{4}"
phone_numbers = re.findall(pattern, text)
print("Phone numbers found:", phone_numbers)
在这个例子中,\d{3}-\d{3}-\d{4}
是一个正则表达式模式,用于匹配电话号码。
2、替换字符串中的内容
假设你想要将文本中的所有日期格式从MM/DD/YYYY
替换为YYYY-MM-DD
,可以使用正则表达式:
import re
text = "Today's date is 12/31/2023"
pattern = r"(\d{2})/(\d{2})/(\d{4})"
replacement = r"\3-\1-\2"
new_text = re.sub(pattern, replacement, text)
print("Updated text:", new_text)
在这个例子中,(\d{2})/(\d{2})/(\d{4})
是一个正则表达式模式,用于匹配日期格式,\3-\1-\2
是替换字符串,用于将日期格式转换为YYYY-MM-DD
。
3、验证密码强度
假设你想要验证用户输入的密码是否符合强度要求,可以使用正则表达式:
import re
password = "StrongPass1!"
pattern = r"^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$"
if re.match(pattern, password):
print("Password is strong")
else:
print("Password is weak")
在这个例子中,^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$
是一个正则表达式模式,用于验证密码是否至少包含一个小写字母、一个大写字母、一个数字、一个特殊字符,且长度至少为8。
总结
Python中有多种方法可以实现字符串匹配,包括使用in
关键字、str.find()
方法、str.index()
方法、正则表达式、和str.startswith()
及str.endswith()
方法。根据具体需求选择合适的方法,可以帮助你高效地处理字符串匹配问题。正则表达式是最强大和灵活的方法,适用于复杂的字符串匹配和模式识别任务。通过掌握这些方法,你可以在Python编程中更加自如地处理字符串相关的操作。
相关问答FAQs:
如何在Python中使用正则表达式进行字符串匹配?
在Python中,使用re
模块可以方便地进行正则表达式匹配。通过re.search()
、re.match()
和re.findall()
等方法,可以查找字符串中的模式。例如,使用re.search(r'模式', '目标字符串')
可以查找目标字符串中是否包含指定模式。
Python中有哪些常用的方法可以进行字符串比较和匹配?
在Python中,可以使用in
关键字检查一个字符串是否包含另一个字符串。此外,str.startswith()
和str.endswith()
方法可以用来检查一个字符串是否以指定的前缀或后缀开始或结束。对于大小写不敏感的匹配,可以使用str.lower()
或str.upper()
将字符串转换为相同的大小写后再进行比较。
如何处理大小写敏感的字符串匹配问题?
如果需要进行大小写敏感的匹配,可以直接使用字符串的比较操作符,例如使用==
来判断两个字符串是否相等。如果希望忽略大小写,可以将两个字符串都转换为小写或大写后再进行比较,例如:str1.lower() == str2.lower()
。这样可以确保匹配不受大小写的影响。
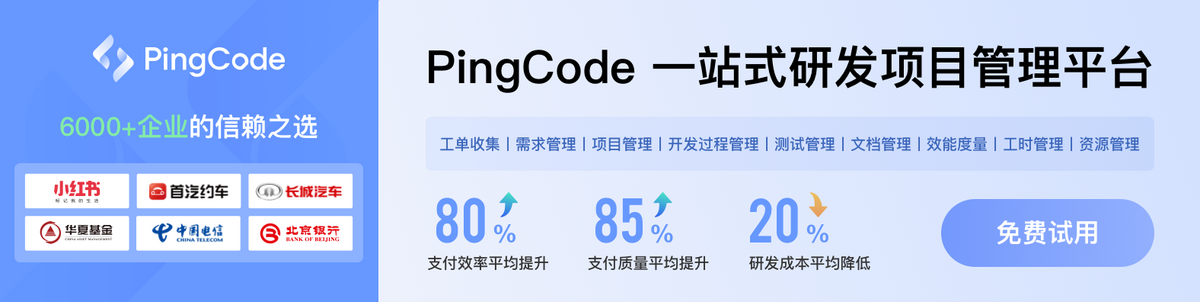