用Python写一个秒杀程序的核心要点包括:实现高效并发、减少延迟、确保数据一致性。这些都需要通过合理的代码结构、利用多线程或异步编程、以及优化网络请求来实现。下面将详细阐述如何从各个方面来实现一个秒杀程序。
一、需求分析与准备
在编写秒杀程序之前,首先需要明确秒杀的具体需求,并准备好相应的环境和工具。秒杀程序通常需要处理大量的并发请求,因此在编写之前需要做出以下准备:
- 需求明确:确定秒杀的商品、时间、用户参与方式等。
- 测试环境:搭建一个模拟秒杀场景的测试环境,确保程序在真实环境下能够正常运行。
- 依赖库:安装必要的Python库,例如requests、threading、asyncio等。
二、实现高效并发
高效并发是秒杀程序的核心,Python提供了多线程、多进程和异步编程三种方式来实现并发。
1. 多线程
多线程可以在一定程度上提高程序的并发处理能力,但由于Python的GIL(全局解释器锁),在CPU密集型任务中并不高效。对于秒杀这种I/O密集型任务,多线程仍然是一种可行的选择。
import threading
import requests
def seckill_task(url, params):
response = requests.post(url, data=params)
print(response.text)
threads = []
for i in range(100):
t = threading.Thread(target=seckill_task, args=('http://example.com/seckill', {'product_id': 1, 'user_id': i}))
threads.append(t)
t.start()
for t in threads:
t.join()
2. 多进程
多进程能够绕过GIL限制,适用于CPU密集型任务。然而,秒杀程序更多是网络I/O操作,因此多进程的优势不明显,但仍可以考虑。
from multiprocessing import Process
import requests
def seckill_task(url, params):
response = requests.post(url, data=params)
print(response.text)
processes = []
for i in range(100):
p = Process(target=seckill_task, args=('http://example.com/seckill', {'product_id': 1, 'user_id': i}))
processes.append(p)
p.start()
for p in processes:
p.join()
3. 异步编程
异步编程能够更高效地处理I/O密集型任务。Python的asyncio库提供了强大的异步编程功能,非常适合秒杀程序。
import asyncio
import aiohttp
async def seckill_task(session, url, params):
async with session.post(url, data=params) as response:
print(await response.text())
async def main():
async with aiohttp.ClientSession() as session:
tasks = []
for i in range(100):
task = asyncio.create_task(seckill_task(session, 'http://example.com/seckill', {'product_id': 1, 'user_id': i}))
tasks.append(task)
await asyncio.gather(*tasks)
asyncio.run(main())
三、减少延迟
减少延迟是秒杀程序成功的关键,以下是几种常见的优化方法:
1. 优化网络请求
使用高效的HTTP库,例如requests、aiohttp等,并尽量减少网络请求的次数和数据传输量。
import requests
def optimized_request(url, params):
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.post(url, data=params, headers=headers, timeout=1)
return response.text
2. 缓存机制
利用缓存机制减少服务器的负载和响应时间。例如,可以将用户信息、商品信息等缓存到内存中,避免频繁查询数据库。
import time
import functools
cache = {}
def cache_result(timeout):
def decorator(func):
@functools.wraps(func)
def wrapper(*args, kwargs):
key = (args, tuple(kwargs.items()))
if key in cache and time.time() - cache[key]['time'] < timeout:
return cache[key]['result']
result = func(*args, kwargs)
cache[key] = {'result': result, 'time': time.time()}
return result
return wrapper
return decorator
@cache_result(timeout=60)
def get_product_info(product_id):
# 模拟查询数据库
time.sleep(1)
return {'product_id': product_id, 'name': 'Product Name'}
print(get_product_info(1))
print(get_product_info(1))
四、确保数据一致性
秒杀程序需要确保数据的一致性,避免超卖或库存不足的情况。以下是几种常见的方法:
1. 乐观锁
乐观锁通过版本号或时间戳来控制并发更新,适用于读多写少的场景。
import threading
class OptimisticLock:
def __init__(self):
self.version = 0
self.lock = threading.Lock()
def update(self, update_func):
with self.lock:
current_version = self.version
result = update_func()
if current_version != self.version:
raise Exception("Version conflict")
self.version += 1
return result
optimistic_lock = OptimisticLock()
def update_inventory():
# 模拟更新库存
print("Updating inventory")
return True
try:
optimistic_lock.update(update_inventory)
except Exception as e:
print(e)
2. 悲观锁
悲观锁通过加锁的方式控制并发更新,适用于写多读少的场景。
import threading
class PessimisticLock:
def __init__(self):
self.lock = threading.Lock()
def update(self, update_func):
with self.lock:
return update_func()
pessimistic_lock = PessimisticLock()
def update_inventory():
# 模拟更新库存
print("Updating inventory")
return True
pessimistic_lock.update(update_inventory)
3. 分布式锁
在分布式系统中,可以使用分布式锁来控制并发更新,例如使用Redis的setnx命令。
import redis
import time
class DistributedLock:
def __init__(self, redis_client, lock_key, timeout=10):
self.redis_client = redis_client
self.lock_key = lock_key
self.timeout = timeout
def acquire(self):
while not self.redis_client.setnx(self.lock_key, time.time() + self.timeout):
lock_value = self.redis_client.get(self.lock_key)
if lock_value and float(lock_value) < time.time():
self.redis_client.delete(self.lock_key)
time.sleep(0.1)
def release(self):
self.redis_client.delete(self.lock_key)
redis_client = redis.StrictRedis(host='localhost', port=6379, db=0)
distributed_lock = DistributedLock(redis_client, 'seckill_lock')
def update_inventory():
# 模拟更新库存
print("Updating inventory")
return True
distributed_lock.acquire()
try:
update_inventory()
finally:
distributed_lock.release()
五、日志与监控
在秒杀活动中,日志和监控非常重要,可以帮助我们及时发现和解决问题。以下是常见的日志和监控工具:
1. 日志
使用Python的logging库记录关键操作和错误信息。
import logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def seckill_task(url, params):
try:
response = requests.post(url, data=params)
logging.info('Seckill success: %s', response.text)
except Exception as e:
logging.error('Seckill failed: %s', e)
seckill_task('http://example.com/seckill', {'product_id': 1, 'user_id': 1})
2. 监控
使用Prometheus、Grafana等监控工具对系统进行实时监控,及时发现和解决问题。
# prometheus.yml
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'seckill'
static_configs:
- targets: ['localhost:8000']
from prometheus_client import start_http_server, Summary
REQUEST_TIME = Summary('request_processing_seconds', 'Time spent processing request')
@REQUEST_TIME.time()
def process_request():
time.sleep(1)
if __name__ == '__main__':
start_http_server(8000)
while True:
process_request()
六、总结
通过上述步骤,我们可以使用Python编写一个高效的秒杀程序。首先,明确需求并准备环境;其次,实现高效并发;然后,减少延迟;最后,确保数据一致性,并使用日志和监控工具对系统进行监控。通过这些步骤,我们能够有效地应对秒杀活动中的高并发和高压力,确保系统的稳定性和数据的一致性。希望本文对您编写秒杀程序有所帮助。
相关问答FAQs:
如何用Python编写一个高效的秒杀程序?
编写一个秒杀程序需要考虑多个方面,包括并发处理、网络请求优化和数据存储等。使用Python的asyncio
库和aiohttp
库可以实现高效的异步请求,从而提升秒杀的成功率。同时,合理设计程序的结构和逻辑也非常关键,确保能够快速响应用户请求。
在秒杀程序中如何处理用户请求并发?
处理用户请求并发是秒杀程序的核心。可以使用多线程或多进程来实现并发处理,Python中的threading
和multiprocessing
库都可以帮助你实现这一功能。此外,使用队列(如queue.Queue
)来管理请求,可以有效防止过载和保证请求的顺序处理。
如何优化Python秒杀程序的网络请求效率?
优化网络请求效率可以通过减少请求的延迟和提高请求的并发量来实现。使用aiohttp
库进行异步请求是一个有效的方法。同时,可以考虑使用连接池,缓存常用数据,或者使用CDN(内容分发网络)加速资源加载,确保秒杀程序在高并发情况下仍能高效运行。
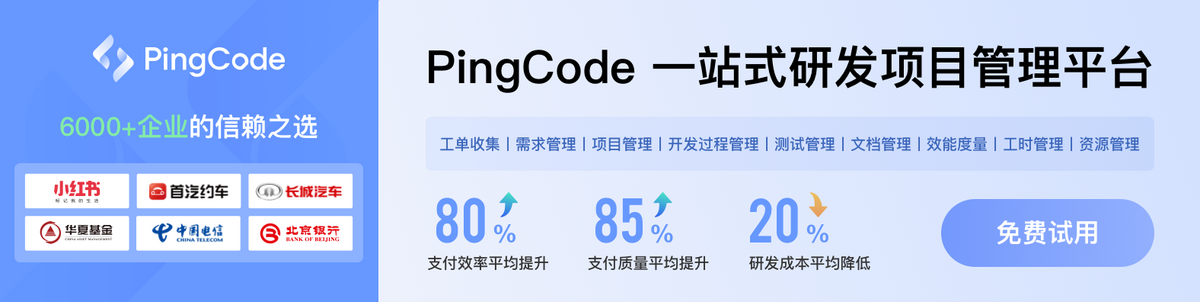