在Python中,要打印出列表的形状,你可以使用多种方法来实现,使用递归函数、使用NumPy库、使用自定义函数。其中,NumPy库方法是较为常用和方便的一种。
一、使用递归函数
递归是一种函数调用自身来解决问题的方法。在Python中,可以使用递归函数来计算并打印列表的形状。
def get_shape(lst):
if isinstance(lst, list):
return [len(lst)] + get_shape(lst[0])
else:
return []
def print_shape(lst):
shape = get_shape(lst)
print("Shape of the list:", shape)
示例
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print_shape(my_list)
在这个示例中,get_shape
函数递归地计算列表的形状,而print_shape
函数则打印出该形状。
二、使用NumPy库
NumPy是一个强大的科学计算库,它提供了许多用于操作数组的函数。在NumPy中,可以使用shape
属性轻松获取数组的形状。
import numpy as np
def print_shape(lst):
np_array = np.array(lst)
print("Shape of the list:", np_array.shape)
示例
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print_shape(my_list)
在这个示例中,我们使用NumPy的array
函数将列表转换为NumPy数组,然后使用shape
属性打印出数组的形状。
三、使用自定义函数
除了递归和NumPy库外,还可以使用自定义函数来计算并打印列表的形状。
def get_shape(lst):
shape = []
while isinstance(lst, list):
shape.append(len(lst))
lst = lst[0] if len(lst) > 0 else []
return shape
def print_shape(lst):
shape = get_shape(lst)
print("Shape of the list:", shape)
示例
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print_shape(my_list)
在这个示例中,get_shape
函数通过循环计算列表的形状,而print_shape
函数则打印出该形状。
四、NumPy库的详细使用
NumPy库是Python中处理数组和矩阵的标准库,具有强大的功能和高效的性能。以下是NumPy库的一些详细使用方法。
1. 安装NumPy
首先,需要安装NumPy库。可以使用以下命令来安装:
pip install numpy
2. 创建NumPy数组
可以使用numpy.array
函数将列表转换为NumPy数组:
import numpy as np
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
np_array = np.array(my_list)
3. 获取数组的形状
可以使用NumPy数组的shape
属性来获取数组的形状:
shape = np_array.shape
print("Shape of the array:", shape)
4. 多维数组
NumPy支持多维数组,可以使用嵌套列表来创建多维数组:
multi_dim_list = [[[1, 2], [3, 4]], [[5, 6], [7, 8]]]
multi_dim_array = np.array(multi_dim_list)
print("Shape of the multi-dimensional array:", multi_dim_array.shape)
五、递归函数的详细使用
递归函数是一种常见的编程技术,它允许函数调用自身来解决问题。以下是一些递归函数的详细使用方法。
1. 基本递归函数
以下是一个基本的递归函数示例:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
print(factorial(5))
2. 递归函数计算列表形状
可以使用递归函数来计算列表的形状,如下所示:
def get_shape(lst):
if isinstance(lst, list):
return [len(lst)] + get_shape(lst[0])
else:
return []
def print_shape(lst):
shape = get_shape(lst)
print("Shape of the list:", shape)
示例
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print_shape(my_list)
3. 递归函数处理多维列表
递归函数可以处理多维列表,计算其形状:
multi_dim_list = [[[1, 2], [3, 4]], [[5, 6], [7, 8]]]
print_shape(multi_dim_list)
六、自定义函数的详细使用
自定义函数是用户定义的函数,可以根据具体需求来实现。以下是一些自定义函数的详细使用方法。
1. 基本自定义函数
以下是一个基本的自定义函数示例:
def add(a, b):
return a + b
print(add(3, 5))
2. 自定义函数计算列表形状
可以使用自定义函数来计算列表的形状,如下所示:
def get_shape(lst):
shape = []
while isinstance(lst, list):
shape.append(len(lst))
lst = lst[0] if len(lst) > 0 else []
return shape
def print_shape(lst):
shape = get_shape(lst)
print("Shape of the list:", shape)
示例
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print_shape(my_list)
3. 自定义函数处理多维列表
自定义函数可以处理多维列表,计算其形状:
multi_dim_list = [[[1, 2], [3, 4]], [[5, 6], [7, 8]]]
print_shape(multi_dim_list)
七、总结
通过以上方法,可以在Python中轻松计算并打印出列表的形状。无论是使用递归函数、NumPy库,还是自定义函数,都可以根据具体需求来选择合适的方法。NumPy库方法是最为常用和方便的一种,适用于处理大多数情况。此外,递归函数和自定义函数也具有其独特的优势,可以根据具体需求来选择。
相关问答FAQs:
如何在Python中获取列表的维度信息?
在Python中,列表本身不直接提供维度信息。如果你想查看一个嵌套列表的维度,可以使用递归函数来计算其维度。例如,对于一个二维列表,可以通过检查每个子列表的长度来判断。对于更高维的列表,你需要递归地深入每一层。
在Python中打印列表的内容时,有什么格式化的技巧?
使用pprint
模块可以更加美观地打印出列表的内容,尤其是当列表包含嵌套结构时。pprint.pprint()
函数会自动调整格式,使得输出更易读。此外,可以使用json.dumps()
方法将列表转换为JSON格式字符串,以便于查看。
如何检查Python列表是否为空或包含元素?
可以使用if not my_list:
来判断一个列表是否为空。如果列表包含元素,条件为假;如果列表为空,条件为真。这种方法很直观,适合快速检查列表状态。
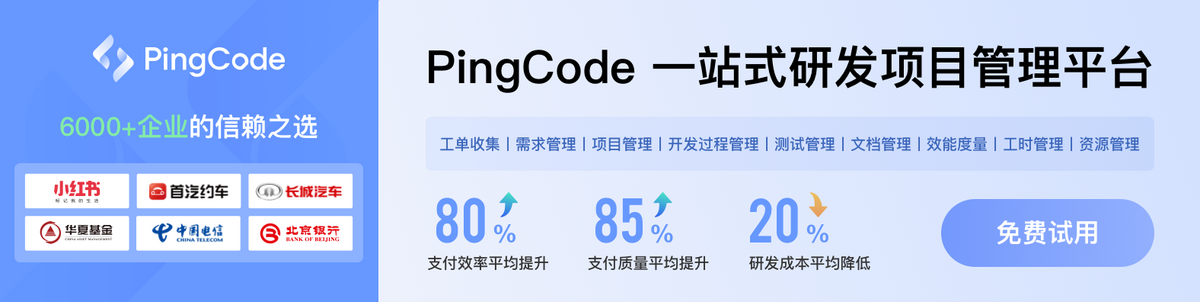