在Python中获取十字光标的值可以使用Tkinter库、pygame库、以及OpenCV库等。下面将详细介绍如何使用这些库来获取十字光标的值,并提供相关示例代码。
一、Tkinter库
Tkinter是Python的标准GUI库,可以轻松创建和管理图形用户界面。通过Tkinter,我们可以创建一个窗口,并在窗口中捕获鼠标事件,以获取十字光标的位置。
创建窗口和绑定鼠标事件
首先,我们需要创建一个Tkinter窗口,并绑定鼠标事件来获取鼠标的位置。
import tkinter as tk
def on_mouse_move(event):
x, y = event.x, event.y
print(f"Mouse position: ({x}, {y})")
root = tk.Tk()
root.title("Tkinter Mouse Position")
canvas = tk.Canvas(root, width=400, height=400)
canvas.pack()
canvas.bind("<Motion>", on_mouse_move)
root.mainloop()
在这个示例中,我们创建了一个Tkinter窗口,并在画布上绑定了一个鼠标移动事件。当鼠标在画布上移动时,on_mouse_move
函数将被调用,并打印鼠标的当前位置。
显示十字光标
为了显示十字光标,我们可以在画布上绘制一个十字线,并更新其位置。
import tkinter as tk
def on_mouse_move(event):
x, y = event.x, event.y
canvas.coords(crosshair_h, 0, y, 400, y)
canvas.coords(crosshair_v, x, 0, x, 400)
print(f"Mouse position: ({x}, {y})")
root = tk.Tk()
root.title("Tkinter Crosshair")
canvas = tk.Canvas(root, width=400, height=400)
canvas.pack()
crosshair_h = canvas.create_line(0, 0, 0, 0, fill="red")
crosshair_v = canvas.create_line(0, 0, 0, 0, fill="red")
canvas.bind("<Motion>", on_mouse_move)
root.mainloop()
在这个示例中,我们在画布上创建了两条线,分别表示十字光标的水平线和垂直线。然后在on_mouse_move
函数中更新这两条线的位置,以显示十字光标。
二、pygame库
Pygame是一个跨平台的Python模块,用于开发游戏。我们可以使用pygame来创建一个窗口,并获取鼠标的位置。
创建窗口和获取鼠标位置
首先,我们需要创建一个pygame窗口,并获取鼠标的位置。
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption("Pygame Mouse Position")
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
x, y = pygame.mouse.get_pos()
print(f"Mouse position: ({x}, {y})")
screen.fill((255, 255, 255))
pygame.display.flip()
pygame.quit()
在这个示例中,我们创建了一个pygame窗口,并在主循环中获取鼠标的位置。
显示十字光标
为了显示十字光标,我们可以在窗口中绘制一个十字线,并更新其位置。
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption("Pygame Crosshair")
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
x, y = pygame.mouse.get_pos()
print(f"Mouse position: ({x}, {y})")
screen.fill((255, 255, 255))
pygame.draw.line(screen, (255, 0, 0), (0, y), (400, y))
pygame.draw.line(screen, (255, 0, 0), (x, 0), (x, 400))
pygame.display.flip()
pygame.quit()
在这个示例中,我们在窗口中绘制了两条线,分别表示十字光标的水平线和垂直线。然后在主循环中更新这两条线的位置,以显示十字光标。
三、OpenCV库
OpenCV是一个开源计算机视觉库,可以用于图像处理和计算机视觉任务。我们可以使用OpenCV来创建一个窗口,并获取鼠标的位置。
创建窗口和绑定鼠标事件
首先,我们需要创建一个OpenCV窗口,并绑定鼠标事件来获取鼠标的位置。
import cv2
def on_mouse_move(event, x, y, flags, param):
if event == cv2.EVENT_MOUSEMOVE:
print(f"Mouse position: ({x}, {y})")
cv2.namedWindow("OpenCV Mouse Position")
cv2.setMouseCallback("OpenCV Mouse Position", on_mouse_move)
while True:
img = 255 * np.ones((400, 400, 3), np.uint8)
cv2.imshow("OpenCV Mouse Position", img)
if cv2.waitKey(1) & 0xFF == 27:
break
cv2.destroyAllWindows()
在这个示例中,我们创建了一个OpenCV窗口,并绑定了一个鼠标移动事件。当鼠标在窗口中移动时,on_mouse_move
函数将被调用,并打印鼠标的当前位置。
显示十字光标
为了显示十字光标,我们可以在窗口中绘制一个十字线,并更新其位置。
import cv2
import numpy as np
def on_mouse_move(event, x, y, flags, param):
global img
if event == cv2.EVENT_MOUSEMOVE:
img = 255 * np.ones((400, 400, 3), np.uint8)
cv2.line(img, (0, y), (400, y), (0, 0, 255), 1)
cv2.line(img, (x, 0), (x, 400), (0, 0, 255), 1)
print(f"Mouse position: ({x}, {y})")
cv2.namedWindow("OpenCV Crosshair")
cv2.setMouseCallback("OpenCV Crosshair", on_mouse_move)
img = 255 * np.ones((400, 400, 3), np.uint8)
while True:
cv2.imshow("OpenCV Crosshair", img)
if cv2.waitKey(1) & 0xFF == 27:
break
cv2.destroyAllWindows()
在这个示例中,我们在窗口中绘制了两条线,分别表示十字光标的水平线和垂直线。然后在on_mouse_move
函数中更新这两条线的位置,以显示十字光标。
四、总结
在这篇文章中,我们介绍了如何使用Tkinter、pygame和OpenCV库来获取十字光标的值,并显示十字光标。每种方法都有其优缺点,具体选择哪种方法取决于您的应用场景和需求。希望本文对您有所帮助。
相关问答FAQs:
如何在Python中获取十字光标的坐标?
在Python中,若要获取十字光标的坐标,可以使用图形用户界面(GUI)库,如Tkinter或Pygame。具体步骤包括初始化窗口,设置光标样式,并通过事件监听器获取光标位置。通过绑定鼠标移动事件,可以实时捕获光标的X和Y坐标,并在界面上显示。
使用Python库时,如何自定义十字光标的外观?
在Tkinter中,可以使用Canvas组件来绘制自定义的十字光标。通过创建线条和形状,并根据鼠标位置动态更新它们的坐标,可以实现独特的光标效果。Pygame也允许通过绘制图形来创建自定义光标,利用pygame.mouse.get_pos()方法获得当前光标位置。
在数据可视化中,如何利用十字光标进行交互?
在数据可视化工具如Matplotlib中,可以通过添加十字光标功能来提升用户体验。可以使用matplotlib.widgets.Cursor模块,实时显示光标位置及其对应的数据值。这种交互方式不仅提高了图表的可读性,也使得用户能够更加便捷地分析数据。
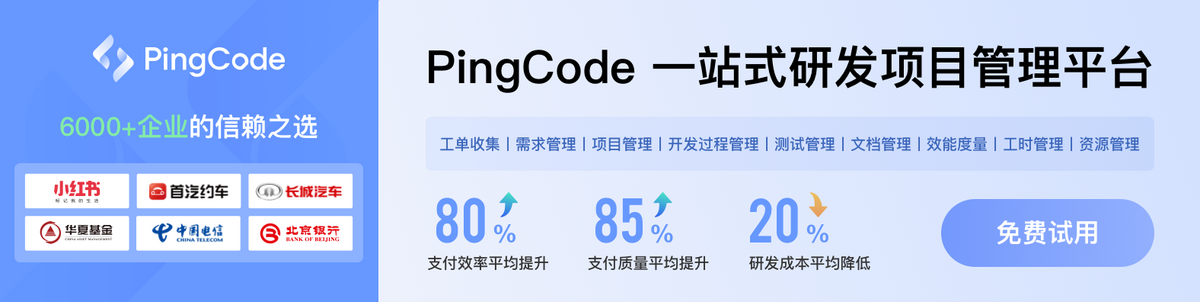