Python输出字符串并添加数据的方法有多种:使用加号连接、使用占位符、使用format()方法、使用f-string。 在这几种方法中,使用f-string 是最简洁和直观的方式。详细解释如下:
使用f-string:f-string 是 Python 3.6 引入的一种格式化字符串的方式,它在字符串前加上字母 'f',并在字符串内部使用大括号 {} 包含表达式。这种方法使代码更简洁可读。
示例代码:
name = "John"
age = 30
print(f"My name is {name} and I am {age} years old.")
在上面的代码中,{name}
和 {age}
会被变量 name
和 age
的值替换,输出结果是:My name is John and I am 30 years old.
接下来,我们将详细介绍Python输出字符串加数据的其他几种方法,包括使用加号连接、占位符和format()方法。
一、使用加号连接
加号连接是最简单的一种方式,通过使用 +
运算符将字符串和其他数据连接在一起。但需要注意的是,所有连接的部分都必须是字符串类型,如果不是,需要使用 str()
函数进行转换。
示例代码:
name = "Alice"
age = 25
print("My name is " + name + " and I am " + str(age) + " years old.")
在上面的代码中,str(age)
将 age
变量的值从整数转换为字符串,然后通过 +
运算符将它们连接在一起。输出结果是:My name is Alice and I am 25 years old.
二、使用占位符
占位符是一种通过 %
运算符将字符串和数据结合的方法。常见的占位符包括 %s
(字符串)、%d
(整数)和 %f
(浮点数)。这种方法在旧版 Python 中非常常见,但在新版 Python 中逐渐被其他方法取代。
示例代码:
name = "Bob"
age = 28
print("My name is %s and I am %d years old." % (name, age))
在上面的代码中,%s
和 %d
是占位符,% (name, age)
将 name
和 age
变量的值依次替换占位符。输出结果是:My name is Bob and I am 28 years old.
三、使用format()方法
format()
方法是一种通过调用字符串对象的 format()
方法将数据插入到字符串中的方法。它使用 {}
作为占位符,并在 format()
方法中传入数据。
示例代码:
name = "Charlie"
age = 22
print("My name is {} and I am {} years old.".format(name, age))
在上面的代码中,{}
是占位符,format(name, age)
将 name
和 age
变量的值依次替换占位符。输出结果是:My name is Charlie and I am 22 years old.
此外,format()
方法还支持通过位置参数和关键字参数进行更灵活的格式化。
示例代码:
name = "David"
age = 35
print("My name is {0} and I am {1} years old.".format(name, age))
print("My name is {name} and I am {age} years old.".format(name=name, age=age))
在上面的代码中,{0}
和 {1}
是位置参数,占位符将被 format()
方法中的第一个和第二个参数替换;{name}
和 {age}
是关键字参数,占位符将被对应的参数值替换。输出结果都是:My name is David and I am 35 years old.
四、使用f-string
f-string 是 Python 3.6 引入的一种格式化字符串的方式,它在字符串前加上字母 'f',并在字符串内部使用大括号 {} 包含表达式。这种方法使代码更简洁可读。
示例代码:
name = "Eve"
age = 27
print(f"My name is {name} and I am {age} years old.")
在上面的代码中,{name}
和 {age}
会被变量 name
和 age
的值替换,输出结果是:My name is Eve and I am 27 years old.
f-string 还支持在大括号内进行表达式计算。
示例代码:
name = "Frank"
age = 40
print(f"My name is {name} and I will be {age + 1} years old next year.")
在上面的代码中,{age + 1}
表达式会被计算结果替换,输出结果是:My name is Frank and I will be 41 years old next year.
总结
Python 提供了多种输出字符串并添加数据的方法,包括加号连接、占位符、format()方法和f-string。在这几种方法中,f-string 是最简洁和直观的方式,尤其在处理复杂表达式时非常方便。不同的方法各有优缺点,开发者可以根据具体需求选择合适的方式。
加号连接:
- 简单直观,但需要手动转换数据类型。
占位符:
- 适用于简单的字符串格式化,但不支持复杂表达式。
format()方法:
- 强大灵活,但语法较为冗长。
f-string:
- 简洁直观,支持复杂表达式,是推荐的字符串格式化方式。
在实际开发中,推荐使用 f-string 进行字符串格式化,以提高代码的可读性和可维护性。希望通过本文的介绍,读者能够更好地理解和掌握 Python 输出字符串并添加数据的方法,提高编程效率和代码质量。
相关问答FAQs:
如何在Python中将字符串与数字结合输出?
在Python中,可以使用格式化字符串来将字符串与数字结合输出。常用的方法包括使用f-string
(Python 3.6及以上版本)、format()
方法以及百分号%
格式化。例如:
name = "Alice"
age = 30
print(f"{name} is {age} years old.") # 使用f-string
print("{} is {} years old.".format(name, age)) # 使用format方法
print("%s is %d years old." % (name, age)) # 使用百分号格式化
这些方法都可以有效地将字符串和数字组合在一起。
Python中可以如何控制字符串输出的格式?
在Python中,可以通过格式化选项控制字符串的输出格式。使用format()
方法或f-string
时,可以指定宽度、对齐方式、精度等。例如:
value = 12.34567
print(f"{value:.2f}") # 输出保留两位小数
print("{:<10} is {:>5}".format("Item", 5)) # 左对齐和右对齐
这样可以确保输出符合特定的格式要求。
在Python中如何连接多个字符串和数字进行输出?
连接多个字符串和数字可以使用多种方式,最常见的是使用+
运算符或格式化方法。以下是几个示例:
part1 = "The total is "
number = 100
result = part1 + str(number) # 使用+运算符连接
print(result)
# 使用format
print("The total is {}".format(number))
# 使用f-string
print(f"The total is {number}")
无论使用哪种方法,都可以实现字符串与数字的有效连接。
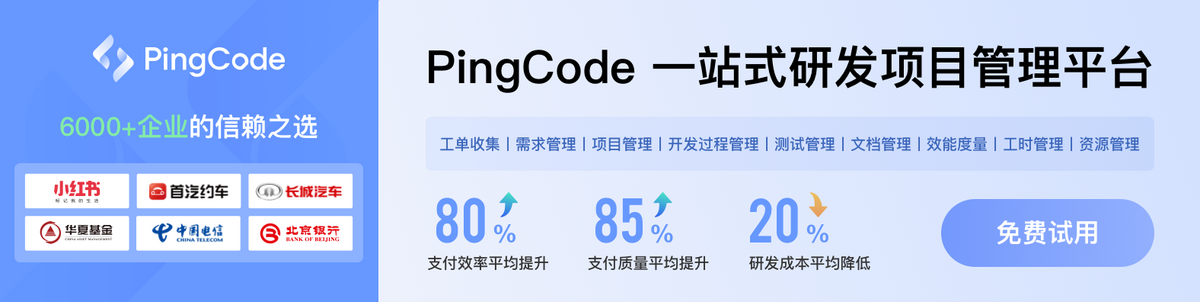