在Python中,您可以使用比较运算符(例如,>
、<
、==
、!=
、>=
、<=
)来比较两个值。通过这些运算符可以直接比较数值、字符串以及其他可比较的数据类型,并输出比较结果。 例如,可以使用if
语句结合比较运算符来输出两个数值的比较结果:使用比较运算符、结合if
语句和逻辑运算符、利用Python内置函数进行比较。接下来我们详细介绍一种方法:使用比较运算符。
一、使用比较运算符
比较运算符是Python中用于比较两个值的基础工具。以下是一些常用的比较运算符及其用法:
>
大于<
小于==
等于!=
不等于>=
大于等于<=
小于等于
示例代码
以下是一个示例代码,展示如何使用这些运算符来比较两个数值,并输出比较结果:
a = 10
b = 20
if a > b:
print(f"{a} is greater than {b}")
elif a < b:
print(f"{a} is less than {b}")
else:
print(f"{a} is equal to {b}")
这段代码将会输出 10 is less than 20
,因为变量a
的值小于变量b
的值。
二、结合if
语句和逻辑运算符
通过结合if
语句和逻辑运算符(如and
、or
、not
),可以实现更复杂的比较逻辑。
示例代码
以下是一个示例代码,展示如何结合if
语句和逻辑运算符进行复杂的比较:
x = 15
y = 20
z = 15
if x < y and x == z:
print(f"{x} is less than {y} and equal to {z}")
elif y > z or z != x:
print(f"{y} is greater than {z} or {z} is not equal to {x}")
else:
print(f"None of the conditions met")
这段代码将会输出 15 is less than 20 and equal to 15
,因为x < y
并且x == z
的条件成立。
三、利用Python内置函数进行比较
Python提供了一些内置函数,如max()
、min()
、sorted()
,可以用来进行比较和排序操作。
示例代码
以下是一个示例代码,展示如何使用这些内置函数进行比较:
numbers = [5, 10, 15, 20, 25]
max_number = max(numbers)
min_number = min(numbers)
sorted_numbers = sorted(numbers)
print(f"The maximum number is {max_number}")
print(f"The minimum number is {min_number}")
print(f"The sorted numbers are {sorted_numbers}")
这段代码将会输出:
The maximum number is 25
The minimum number is 5
The sorted numbers are [5, 10, 15, 20, 25]
四、比较字符串
Python允许比较字符串,比较的规则是按字典序(ASCII值)进行比较。
示例代码
以下是一个示例代码,展示如何比较两个字符串:
str1 = "apple"
str2 = "banana"
if str1 < str2:
print(f"{str1} comes before {str2} in alphabetical order")
elif str1 > str2:
print(f"{str1} comes after {str2} in alphabetical order")
else:
print(f"{str1} is equal to {str2}")
这段代码将会输出 apple comes before banana in alphabetical order
,因为字符串"apple"
在字典序中位于"banana"
之前。
五、比较自定义对象
在Python中,您还可以比较自定义对象。要实现这一点,需要在自定义类中实现比较运算符方法,如__lt__
(小于)、__le__
(小于等于)、__eq__
(等于)、__ne__
(不等于)、__gt__
(大于)和__ge__
(大于等于)。
示例代码
以下是一个示例代码,展示如何在自定义类中实现比较运算符:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __lt__(self, other):
return self.age < other.age
def __le__(self, other):
return self.age <= other.age
def __eq__(self, other):
return self.age == other.age
def __ne__(self, other):
return self.age != other.age
def __gt__(self, other):
return self.age > other.age
def __ge__(self, other):
return self.age >= other.age
person1 = Person("Alice", 30)
person2 = Person("Bob", 25)
if person1 > person2:
print(f"{person1.name} is older than {person2.name}")
elif person1 < person2:
print(f"{person1.name} is younger than {person2.name}")
else:
print(f"{person1.name} and {person2.name} are of the same age")
这段代码将会输出 Alice is older than Bob
,因为person1
的年龄大于person2
的年龄。
六、总结
通过上述方法,您可以在Python中实现各种类型的数据比较,并输出比较结果。无论是使用基本的比较运算符、结合if
语句和逻辑运算符、利用内置函数进行比较,还是比较字符串和自定义对象,这些方法都能够满足不同场景下的比较需求。了解并掌握这些方法,可以帮助您在编程过程中更高效地处理数据比较操作。
相关问答FAQs:
如何在Python中比较两个数字的大小并输出结果?
在Python中,可以使用简单的条件语句来比较两个数字。利用if
语句来判断哪个数字更大,或者它们是否相等。例如,可以使用如下代码:
a = 5
b = 10
if a > b:
print(f"{a} is greater than {b}")
elif a < b:
print(f"{a} is less than {b}")
else:
print(f"{a} is equal to {b}")
这种方式能够清晰地输出比较结果。
Python是否提供内置函数来比较多个数字?
是的,Python的内置函数max()
和min()
可以用于比较多个数字并返回最大的或最小的值。例如:
numbers = [3, 1, 4, 1, 5, 9]
largest = max(numbers)
smallest = min(numbers)
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
这种方法非常适合需要比较一组数字的场景。
如何在Python中比较字符串的大小?
在Python中,字符串比较是基于字典序进行的。可以直接使用>
、<
、==
等运算符来比较两个字符串。比如:
str1 = "apple"
str2 = "banana"
if str1 < str2:
print(f"{str1} comes before {str2} in alphabetical order.")
elif str1 > str2:
print(f"{str1} comes after {str2} in alphabetical order.")
else:
print(f"{str1} is the same as {str2}.")
这种方式能够直观地显示字符串之间的比较结果。
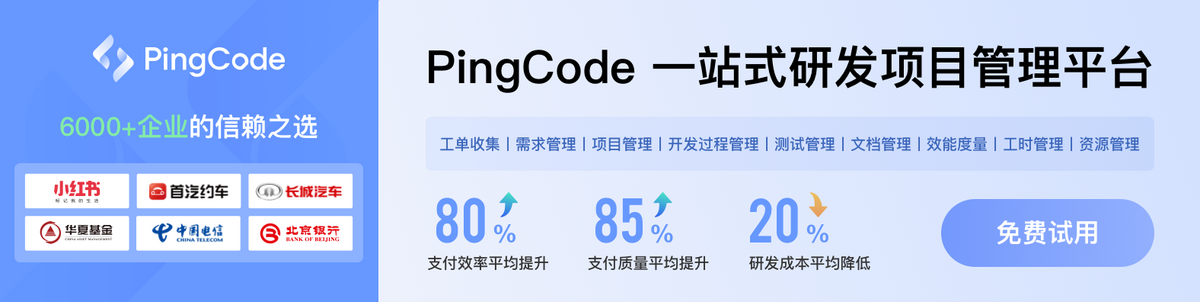