在Python中调用基类的成员方法有多种方式,主要包括使用类名直接调用、使用super()函数调用。这两种方式各有其应用场景,下面我将详细解释并演示如何使用它们。
使用类名直接调用
使用类名直接调用基类的方法是一种显式调用父类方法的方式。这种方法比较直观,适合在方法重写时依然需要调用父类的同名方法的场景。
class BaseClass:
def base_method(self):
print("Base class method")
class DerivedClass(BaseClass):
def base_method(self):
# Call the base class method
BaseClass.base_method(self)
print("Derived class method")
Create an instance of DerivedClass
derived = DerivedClass()
derived.base_method()
在这个例子中,DerivedClass
重写了 base_method
,但仍然需要调用 BaseClass
的 base_method
。使用类名直接调用时,需要显式地传递 self
。
使用super()函数调用
super()
函数是Python中调用基类方法的推荐方式。它提供了一种隐式调用基类方法的方式,能够正确处理多重继承情况。
class BaseClass:
def base_method(self):
print("Base class method")
class DerivedClass(BaseClass):
def base_method(self):
# Call the base class method
super().base_method()
print("Derived class method")
Create an instance of DerivedClass
derived = DerivedClass()
derived.base_method()
在这个例子中,使用 super()
函数调用基类的 base_method
。super()
处理了 self
的传递,代码更加简洁。
二、使用类名直接调用的详细描述
使用类名直接调用基类方法是最直接的方式之一,尤其在简单的继承结构中,这种方式显得非常直观。通过这种方式调用基类方法时,我们必须显式地传递实例 self
。这种调用方式的主要优点是清晰明确,适合于单继承或继承层次比较浅的情况。
在多继承结构中,类名直接调用的方式可能会导致代码的可读性降低,并且更容易出错,因为每个基类的方法都需要显式调用。使用类名直接调用的方法适用于以下情况:
- 简单继承结构:继承结构简单,类与类之间的关系明确。
- 显式调用:需要明确地展示调用关系,代码可读性高。
三、多重继承中的super()调用
在多重继承结构中,super()
函数显得尤为重要。它能够自动处理继承树中的方法解析顺序(MRO),避免了显式调用基类方法的复杂性。下面是一个多重继承的例子:
class BaseClass1:
def base_method(self):
print("Base class 1 method")
class BaseClass2:
def base_method(self):
print("Base class 2 method")
class DerivedClass(BaseClass1, BaseClass2):
def base_method(self):
# Call the base class method
super().base_method()
print("Derived class method")
Create an instance of DerivedClass
derived = DerivedClass()
derived.base_method()
在这个例子中,super()
函数按照MRO顺序调用 BaseClass1
的 base_method
。在多重继承的复杂结构中,super()
使代码的可维护性和可读性更高。
四、重写初始化方法并调用基类初始化
在实际开发中,经常会遇到重写类的初始化方法 __init__
的情况。在这种情况下,我们需要调用基类的 __init__
方法以确保基类的初始化代码被执行。
class BaseClass:
def __init__(self, value):
self.value = value
print(f"Base class init with value: {value}")
class DerivedClass(BaseClass):
def __init__(self, value, extra):
# Call the base class __init__
super().__init__(value)
self.extra = extra
print(f"Derived class init with extra: {extra}")
Create an instance of DerivedClass
derived = DerivedClass(10, 20)
在这个例子中,DerivedClass
重写了 __init__
方法,并在其中调用了基类的 __init__
方法,确保基类的初始化代码被正确执行。
五、调用多重继承中的特定基类方法
在多重继承中,有时需要调用特定基类的方法,而不是按照MRO顺序调用。此时,可以使用类名直接调用的方式:
class BaseClass1:
def base_method(self):
print("Base class 1 method")
class BaseClass2:
def base_method(self):
print("Base class 2 method")
class DerivedClass(BaseClass1, BaseClass2):
def base_method(self):
# Call a specific base class method
BaseClass2.base_method(self)
print("Derived class method")
Create an instance of DerivedClass
derived = DerivedClass()
derived.base_method()
在这个例子中,DerivedClass
调用了 BaseClass2
的 base_method
,而不是按照MRO顺序调用 BaseClass1
的 base_method
。这种方式适用于需要显式调用特定基类方法的情况。
六、总结
在Python中调用基类的方法主要有两种方式:使用类名直接调用和使用 super()
函数调用。类名直接调用适用于简单继承结构,代码清晰明确;super()
函数调用适用于多重继承结构,能够自动处理方法解析顺序,提高代码的可维护性。
在实际开发中,应根据具体情况选择合适的调用方式,以确保代码的可读性和维护性。在多重继承结构中,推荐使用 super()
函数调用基类方法,以避免显式调用带来的复杂性。
相关问答FAQs:
在Python中,如何使用super()来调用基类的方法?
使用super()
函数可以方便地调用基类的成员方法,而不需要直接引用基类的名字。这种方式可以确保在多重继承的情况下正确地调用父类的方法。示例代码如下:
class Base:
def greet(self):
return "Hello from Base!"
class Derived(Base):
def greet(self):
return super().greet() + " And also from Derived!"
d = Derived()
print(d.greet()) # 输出: Hello from Base! And also from Derived!
在什么情况下需要调用基类的成员方法?
调用基类的成员方法通常在子类中需要扩展或修改基类的功能时使用。例如,在子类中重写一个方法时,可以使用基类的方法来执行一些基础逻辑,然后在此基础上添加更多的功能。这样可以避免重复代码,增强代码的可维护性。
基类的方法可以被重写吗?如何进行?
基类的方法可以被子类重写,重写的方法需要与基类中的方法同名,并且参数列表保持一致。在重写时,如果需要保留基类的方法实现,可以通过super()
调用基类的方法。示例:
class Animal:
def sound(self):
return "Some sound"
class Dog(Animal):
def sound(self):
base_sound = super().sound()
return base_sound + " and Bark!"
dog = Dog()
print(dog.sound()) # 输出: Some sound and Bark!
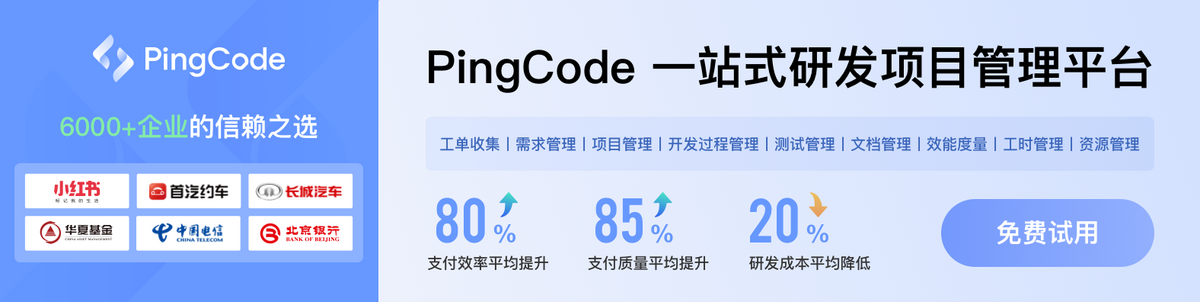