在Python中,有多种方法可以从文件的第n行开始读取。其中较为常用的方法包括使用itertools.islice
、enumerate
函数以及手动循环控制。这里,我们将详细介绍这些方法,并对其中的itertools.islice
进行详细描述。
itertools.islice
是Python标准库itertools
中的一个函数,它允许我们在处理迭代器时进行切片操作。通过itertools.islice
,我们可以指定从文件的第n行开始读取,而无需将整个文件内容加载到内存中,这在处理大文件时尤为有用。
import itertools
def read_file_from_nth_line(file_path, n):
with open(file_path, 'r') as file:
for line in itertools.islice(file, n, None):
print(line, end='')
使用示例
read_file_from_nth_line('example.txt', 5)
在上面的示例中,itertools.islice
函数接收三个参数:迭代器(这里是文件对象)、开始位置、结束位置(这里为None
表示读取到文件末尾)。这种方法有效地提高了读取效率,并节省了内存。
接下来,我们将详细介绍其他方法,并对其优缺点进行比较。
一、使用itertools.islice
正如前面提到的,itertools.islice
是一个高效的选择。它能在不将整个文件读入内存的情况下,从指定行开始读取文件。
优点:
- 高效节省内存:适合处理大文件。
- 简洁易读:代码简洁,易于理解和维护。
缺点:
- 依赖外部库:需要导入
itertools
库。
以下是使用itertools.islice
的详细示例:
import itertools
def read_file_from_nth_line(file_path, n):
with open(file_path, 'r') as file:
for line in itertools.islice(file, n, None):
print(line, end='')
使用示例
read_file_from_nth_line('example.txt', 5)
二、使用enumerate
函数
enumerate
函数可以在遍历文件时提供行号,从而实现从指定行开始读取。
优点:
- 无需额外导入库:依赖于内置函数。
- 可读性高:行号和内容一目了然。
缺点:
- 性能较低:对于大文件,效率不如
itertools.islice
。
以下是使用enumerate
的详细示例:
def read_file_from_nth_line(file_path, n):
with open(file_path, 'r') as file:
for i, line in enumerate(file):
if i >= n:
print(line, end='')
使用示例
read_file_from_nth_line('example.txt', 5)
三、手动循环控制
手动控制循环是一种最原始的方法,通过显式的循环和计数器来控制从指定行开始读取。
优点:
- 简单直接:无需导入任何库,逻辑直观。
缺点:
- 冗长繁琐:代码相对冗长,不利于维护。
- 性能较低:效率不如前两种方法。
以下是手动循环控制的详细示例:
def read_file_from_nth_line(file_path, n):
with open(file_path, 'r') as file:
current_line = 0
for line in file:
if current_line >= n:
print(line, end='')
current_line += 1
使用示例
read_file_from_nth_line('example.txt', 5)
四、总结
在实际应用中,选择哪种方法取决于具体场景和需求。对于大文件,推荐使用itertools.islice
,因为它能高效地处理文件并节省内存。对于小文件或简单任务,可以使用enumerate
或手动循环控制。这些方法各有优缺点,开发者可以根据需求选择最合适的方案。
示例总结:
-
itertools.islice
:import itertools
def read_file_from_nth_line(file_path, n):
with open(file_path, 'r') as file:
for line in itertools.islice(file, n, None):
print(line, end='')
read_file_from_nth_line('example.txt', 5)
-
enumerate
:def read_file_from_nth_line(file_path, n):
with open(file_path, 'r') as file:
for i, line in enumerate(file):
if i >= n:
print(line, end='')
read_file_from_nth_line('example.txt', 5)
-
手动循环控制:
def read_file_from_nth_line(file_path, n):
with open(file_path, 'r') as file:
current_line = 0
for line in file:
if current_line >= n:
print(line, end='')
current_line += 1
read_file_from_nth_line('example.txt', 5)
通过这些方法,开发者可以根据具体需求灵活选择,从而高效地从文件的第n行开始读取内容。
相关问答FAQs:
如何在Python中有效地读取文件的特定行数?
在Python中,可以使用内置的open()
函数结合readlines()
方法来读取文件的特定行数。通过将文件中的所有行读入列表,然后选择需要的行,可以灵活地从任何行开始读取文件。例如,如果想从第n行开始读取文件,可以这样做:
with open('yourfile.txt', 'r') as file:
lines = file.readlines()[n-1:] # 从第n行开始读取
是否可以使用其他方法从特定行开始读取文件?
除了使用readlines()
,可以使用islice
函数从itertools
模块来逐行读取文件,避免将整个文件加载到内存中。这种方法在处理大文件时尤其有效。示例如下:
from itertools import islice
with open('yourfile.txt', 'r') as file:
for line in islice(file, n-1, None): # 从第n行开始读取
print(line)
如何处理文件中不存在的行数?
在读取文件时,如果指定的行数超过文件的总行数,建议添加错误处理机制。可以通过检查文件的总行数或者使用try-except
语句来捕获异常,确保程序在处理此类情况时不会崩溃。例如:
with open('yourfile.txt', 'r') as file:
lines = file.readlines()
if n-1 < len(lines):
print(lines[n-1:])
else:
print("指定的行数超出了文件的总行数。")
如何从文件的末尾开始读取特定行?
如果希望从文件的末尾开始读取特定行,可以先读取文件的所有行,然后利用负索引访问。例如,lines[-n:]
可以返回最后n行。结合readlines()
方法使用时,可以实现:
with open('yourfile.txt', 'r') as file:
lines = file.readlines()[-n:] # 从文件末尾开始读取n行
这些方法可以帮助用户根据不同需求灵活地从文件中读取特定行数。
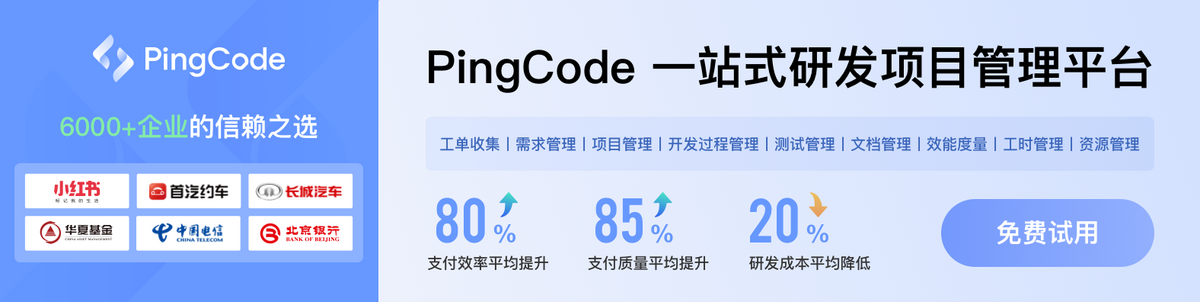