开头段落:
使用Python编程语言进行数据分析、开发Web应用程序、自动化任务、进行机器学习和人工智能研究、编写脚本和实用程序等。Python以其简单易学、功能强大和丰富的库和框架在编程界广受欢迎。在这篇文章中,我们将详细探讨如何用Python实现这些功能,并通过一些实战示例来帮助你更好地理解和应用Python编程。
一、使用Python进行数据分析
Python在数据分析领域表现出色,得益于其强大的库如Pandas、NumPy和Matplotlib等。
1. Pandas库
Pandas是Python中最为重要的数据处理库之一。它提供了高性能、易用的数据结构和数据分析工具。Pandas的核心数据结构是DataFrame,它类似于电子表格,可以有效地处理数据。
-
安装Pandas
pip install pandas
-
基本操作
import pandas as pd
创建DataFrame
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 24, 35, 32]}
df = pd.DataFrame(data)
查看数据
print(df)
数据选择
print(df['Name'])
print(df.loc[0])
数据过滤
print(df[df['Age'] > 30])
2. NumPy库
NumPy是Python中进行科学计算的基础库。它支持多维数组和矩阵运算,并且提供了大量的数学函数库。
-
安装NumPy
pip install numpy
-
基本操作
import numpy as np
创建数组
array = np.array([1, 2, 3, 4, 5])
print(array)
数组运算
print(array + 5)
print(np.mean(array))
print(np.median(array))
3. Matplotlib库
Matplotlib是Python中的绘图库,可以生成各种图表,如折线图、散点图、柱状图等。
-
安装Matplotlib
pip install matplotlib
-
基本操作
import matplotlib.pyplot as plt
创建数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制图表
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Sample Plot')
plt.show()
二、使用Python开发Web应用程序
Python在Web开发方面也有广泛的应用,特别是通过框架如Django和Flask。
1. Django框架
Django是一个高级Python Web框架,鼓励快速开发和简洁、实用的设计。
-
安装Django
pip install django
-
创建Django项目
django-admin startproject myproject
cd myproject
python manage.py runserver
-
创建应用
python manage.py startapp myapp
-
配置应用
在
settings.py
中添加应用:INSTALLED_APPS = [
...
'myapp',
]
-
定义模型
在
myapp/models.py
中定义数据模型:from django.db import models
class Person(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()
-
迁移数据库
python manage.py makemigrations
python manage.py migrate
-
创建视图和模板
在
myapp/views.py
中定义视图:from django.shortcuts import render
from .models import Person
def index(request):
persons = Person.objects.all()
return render(request, 'index.html', {'persons': persons})
在
myapp/templates/index.html
中创建模板:<!DOCTYPE html>
<html>
<head>
<title>Person List</title>
</head>
<body>
<h1>Person List</h1>
<ul>
{% for person in persons %}
<li>{{ person.name }} - {{ person.age }}</li>
{% endfor %}
</ul>
</body>
</html>
2. Flask框架
Flask是一个轻量级的Python Web框架,适合小型应用和微服务。
-
安装Flask
pip install flask
-
创建Flask应用
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
-
创建模板
在
templates/index.html
中创建模板:<!DOCTYPE html>
<html>
<head>
<title>Flask App</title>
</head>
<body>
<h1>Welcome to Flask</h1>
</body>
</html>
三、使用Python进行自动化任务
Python在自动化任务方面也非常强大,常用于Web爬虫、文件处理、系统运维等。
1. Web爬虫
使用Python进行Web爬虫通常会用到库如Requests和BeautifulSoup。
-
安装Requests和BeautifulSoup
pip install requests beautifulsoup4
-
基本操作
import requests
from bs4 import BeautifulSoup
发送请求
response = requests.get('https://example.com')
content = response.content
解析HTML
soup = BeautifulSoup(content, 'html.parser')
print(soup.title.text)
2. 文件处理
Python可以方便地处理各种文件,如文本文件、CSV文件、Excel文件等。
-
处理文本文件
# 读取文件
with open('example.txt', 'r') as file:
content = file.read()
print(content)
写入文件
with open('example.txt', 'w') as file:
file.write('Hello, world!')
-
处理CSV文件
import csv
读取CSV文件
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
写入CSV文件
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age'])
writer.writerow(['John', 28])
-
处理Excel文件
import pandas as pd
读取Excel文件
df = pd.read_excel('example.xlsx')
print(df)
写入Excel文件
df.to_excel('example.xlsx', index=False)
四、使用Python进行机器学习和人工智能研究
Python在机器学习和人工智能研究方面有广泛的应用,常用的库有Scikit-learn、TensorFlow和Keras。
1. Scikit-learn库
Scikit-learn是一个用于机器学习的Python库,提供了简单易用的工具进行数据挖掘和数据分析。
-
安装Scikit-learn
pip install scikit-learn
-
基本操作
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
加载数据集
data = load_iris()
X = data.data
y = data.target
划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
训练模型
model = RandomForestClassifier()
model.fit(X_train, y_train)
预测
y_pred = model.predict(X_test)
评估模型
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
2. TensorFlow和Keras库
TensorFlow是一个用于机器学习和深度学习的开源库,Keras是一个高级神经网络API,可以运行在TensorFlow之上。
-
安装TensorFlow和Keras
pip install tensorflow keras
-
基本操作
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
创建模型
model = Sequential([
Dense(64, activation='relu', input_shape=(4,)),
Dense(64, activation='relu'),
Dense(3, activation='softmax')
])
编译模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
训练模型
model.fit(X_train, y_train, epochs=10, batch_size=32)
评估模型
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Loss: {loss}, Accuracy: {accuracy}')
五、使用Python编写脚本和实用程序
Python非常适合编写各种脚本和实用程序,能够显著提高工作效率。
1. 系统运维脚本
Python可以用于系统运维,帮助自动化日常任务。
-
示例脚本
import os
import shutil
创建目录
os.makedirs('new_directory', exist_ok=True)
复制文件
shutil.copy('source_file.txt', 'new_directory/destination_file.txt')
删除文件
os.remove('new_directory/destination_file.txt')
删除目录
os.rmdir('new_directory')
2. 网络脚本
Python可以用于网络编程,处理网络连接、数据传输等。
-
示例脚本
import socket
创建TCP/IP套接字
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
连接服务器
server_address = ('localhost', 10000)
sock.connect(server_address)
try:
# 发送数据
message = 'This is the message. It will be repeated.'
sock.sendall(message.encode('utf-8'))
# 接收响应
data = sock.recv(1024)
print(f'Received: {data.decode("utf-8")}')
finally:
# 关闭连接
sock.close()
3. 数据处理脚本
Python可以用于处理和转换数据,适用于各种数据格式。
-
示例脚本
import json
import csv
读取JSON文件
with open('data.json', 'r') as file:
data = json.load(file)
写入CSV文件
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(data.keys())
writer.writerow(data.values())
通过以上各个方面的详细讲解和示例代码,相信你已经对如何用Python进行数据分析、开发Web应用程序、自动化任务、进行机器学习和人工智能研究、编写脚本和实用程序有了更深入的理解。Python作为一门功能强大且易于上手的编程语言,能够帮助你在各种编程任务中提高效率,解决实际问题。希望这篇文章能够成为你学习和应用Python的有力参考。
相关问答FAQs:
如何用Python进行数据分析?
Python是数据分析领域中的一种广泛使用语言,拥有丰富的库如Pandas、NumPy和Matplotlib。通过这些库,用户可以轻松地进行数据清洗、处理和可视化。首先,可以使用Pandas读取CSV或Excel文件,然后利用NumPy进行高效的数学运算,最后通过Matplotlib或Seaborn进行图表展示,帮助用户直观理解数据趋势。
Python适合初学者吗?
Python因其简洁的语法和强大的功能而被认为是初学者友好的编程语言。对于没有编程经验的用户来说,Python提供了清晰易懂的代码结构,有助于快速入门。此外,Python拥有大量的学习资源和社区支持,使得新手能够轻松找到解决方案和学习资料。
使用Python进行网页爬虫需要掌握哪些知识?
进行网页爬虫时,用户需要了解基本的HTTP请求和响应机制。此外,掌握Beautiful Soup和Requests库是非常重要的,因为它们可以帮助用户解析HTML文档并提取所需的数据。同时,了解XPath和正则表达式也有助于高效抓取和处理信息,确保数据的准确性和有效性。
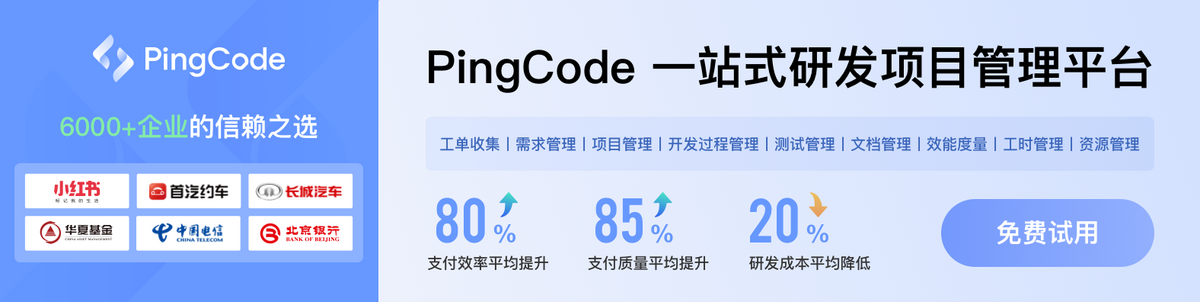