Python中可以通过多种方法将GBK编码转换为UTF-8编码,这些方法包括使用内置的编解码器、pandas库、以及通过手动读取和写入文件等。其中最常见的是使用Python内置的编解码器来完成转换。接下来将详细介绍这些方法。
一、使用Python内置编解码器
Python内置的编解码器使得从一种编码转换为另一种编码变得非常简单。可以通过打开文件并指定读取和写入的编码来完成这一操作。
# 读取GBK编码的文件并将其转换为UTF-8编码
with open('gbk_encoded_file.txt', 'r', encoding='gbk') as file:
content = file.read()
with open('utf8_encoded_file.txt', 'w', encoding='utf-8') as file:
file.write(content)
在这个例子中,首先使用open
函数以GBK编码读取文件内容,然后以UTF-8编码将内容写入新的文件。这种方法非常直观且高效,适用于大多数文件转换需求。
二、使用pandas库
如果需要处理的是包含文本数据的大型数据文件,pandas库提供了非常便捷的方法来进行编码转换。
import pandas as pd
读取GBK编码的CSV文件并将其转换为UTF-8编码
df = pd.read_csv('gbk_encoded_file.csv', encoding='gbk')
df.to_csv('utf8_encoded_file.csv', encoding='utf-8', index=False)
在这个例子中,pandas的read_csv
和to_csv
函数允许我们在读取和写入CSV文件时指定编码。这不仅简单易用,而且处理大型数据文件时也非常高效。
三、手动读取和写入文件
对于某些特殊情况,可能需要手动读取文件内容并逐行转换编码。这种方法虽然稍微繁琐,但可以提供更大的灵活性。
# 手动读取GBK编码的文件并将其转换为UTF-8编码
with open('gbk_encoded_file.txt', 'rb') as file:
content = file.read().decode('gbk')
with open('utf8_encoded_file.txt', 'wb') as file:
file.write(content.encode('utf-8'))
这里使用二进制模式打开文件,并手动进行解码和编码操作。这种方法虽然较为低级,但在处理非文本文件或需要精细控制编码转换过程时非常有用。
四、使用第三方库chardet
有时候文件的编码不明确或者可能包含混合编码,此时可以使用chardet库来自动检测编码并进行转换。
import chardet
自动检测文件编码并将其转换为UTF-8编码
with open('unknown_encoded_file.txt', 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
content = raw_data.decode(encoding)
with open('utf8_encoded_file.txt', 'wb') as file:
file.write(content.encode('utf-8'))
使用chardet库可以自动检测文件编码,确保在读取文件时使用正确的编码。这种方法特别适合处理编码不确定或多种编码混合的文件。
五、批量转换文件编码
在实际应用中,可能需要对多个文件进行编码转换。可以编写一个脚本来批量处理文件。
import os
def convert_encoding(input_dir, output_dir, from_encoding, to_encoding):
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for filename in os.listdir(input_dir):
input_file = os.path.join(input_dir, filename)
output_file = os.path.join(output_dir, filename)
with open(input_file, 'r', encoding=from_encoding) as file:
content = file.read()
with open(output_file, 'w', encoding=to_encoding) as file:
file.write(content)
批量将目录中的文件从GBK转换为UTF-8
convert_encoding('gbk_encoded_files', 'utf8_encoded_files', 'gbk', 'utf-8')
这个脚本定义了一个convert_encoding
函数,可以将指定目录中的所有文件从一种编码转换为另一种编码。通过调用此函数,可以批量处理多个文件,极大地提高了效率。
六、处理异常情况
在实际操作中,可能会遇到文件中包含非法字符或编码错误的情况。为了确保转换过程的顺利进行,可以在编码转换时处理这些异常情况。
# 处理编码转换中的异常情况
try:
with open('gbk_encoded_file.txt', 'r', encoding='gbk', errors='ignore') as file:
content = file.read()
except UnicodeDecodeError as e:
print(f"读取文件时发生错误: {e}")
try:
with open('utf8_encoded_file.txt', 'w', encoding='utf-8', errors='ignore') as file:
file.write(content)
except UnicodeEncodeError as e:
print(f"写入文件时发生错误: {e}")
在这个例子中,使用errors='ignore'
参数来忽略编码转换过程中出现的错误字符。这种方法可以确保文件的绝大部分内容能够顺利转换,但可能会丢失一些非法字符。
总结
通过上述方法,Python提供了多种途径来将GBK编码转换为UTF-8编码。无论是使用内置的编解码器、pandas库、手动读取和写入文件,还是使用chardet库检测编码,Python都能高效地完成编码转换任务。此外,还可以编写脚本批量处理文件并处理异常情况,以满足不同场景下的需求。希望本文对您理解和实现编码转换有所帮助。
相关问答FAQs:
如何在Python中读取GBK编码的文件并转换为UTF-8?
在Python中,可以使用open()
函数来读取GBK编码的文件,并通过指定编码参数将其转换为UTF-8编码。示例代码如下:
with open('file_gbk.txt', 'r', encoding='gbk') as f:
content = f.read()
with open('file_utf8.txt', 'w', encoding='utf-8') as f:
f.write(content)
这个方法可以确保文件内容正确地从GBK转换为UTF-8,并保持原始内容不变。
转换GBK字符串为UTF-8字符串的简单示例是什么?
如果你有一个GBK编码的字符串,想要将其转换为UTF-8编码,可以使用encode()
和decode()
方法。示例代码如下:
gbk_string = '你好'.encode('gbk') # GBK编码
utf8_string = gbk_string.decode('gbk').encode('utf-8') # 转换为UTF-8
print(utf8_string.decode('utf-8')) # 输出UTF-8字符串
这种方式适用于处理字符串,而不是文件。
在Python中如何处理GBK和UTF-8编码的错误?
在处理编码转换时,可能会遇到一些错误,例如字符无法解码或编码。可以通过errors
参数来处理这些错误。例如,使用errors='ignore'
可以忽略无法解码的字符,而使用errors='replace'
则会用一个替代字符来替换它们。示例代码如下:
with open('file_gbk.txt', 'r', encoding='gbk', errors='replace') as f:
content = f.read()
with open('file_utf8.txt', 'w', encoding='utf-8', errors='ignore') as f:
f.write(content)
这样可以确保程序的稳定性,避免因为编码问题导致的崩溃。
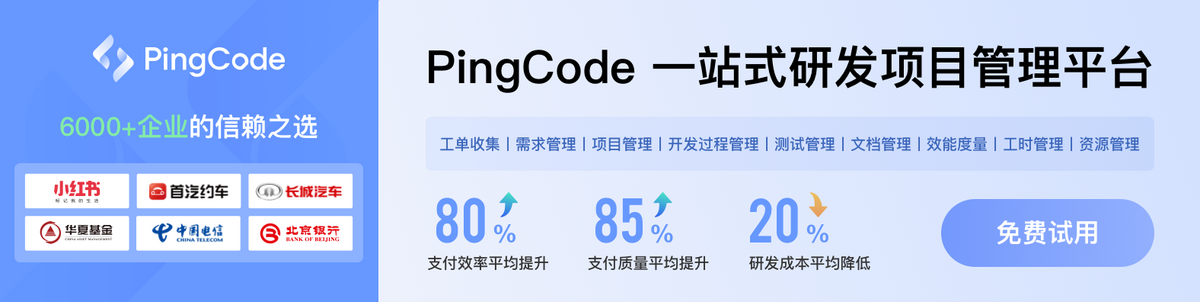