Python根据四个点切图的方法是:使用图像处理库Pillow、使用OpenCV库、使用多边形裁剪。以下将详细介绍使用Pillow库进行四个点切图的方法。
使用Pillow库
Python的Pillow库(PIL)可以方便地进行图像处理,包括裁剪、旋转、缩放等。对于四个点的裁剪,可以使用 Image.crop()
方法,并结合四个点的坐标进行处理。
from PIL import Image
def crop_image_by_points(image_path, points):
"""
根据四个点裁剪图像
:param image_path: 图像路径
:param points: 四个点坐标,格式为[(x1, y1), (x2, y2), (x3, y3), (x4, y4)]
:return: 裁剪后的图像
"""
image = Image.open(image_path)
# 确定裁剪区域的边界框
x_min = min(point[0] for point in points)
x_max = max(point[0] for point in points)
y_min = min(point[1] for point in points)
y_max = max(point[1] for point in points)
# 裁剪图像
cropped_image = image.crop((x_min, y_min, x_max, y_max))
return cropped_image
使用示例
image_path = 'example.jpg'
points = [(50, 50), (150, 50), (150, 150), (50, 150)]
cropped_image = crop_image_by_points(image_path, points)
cropped_image.show()
使用OpenCV库
OpenCV库提供了丰富的图像处理功能,可以方便地进行复杂的图像操作。以下是使用OpenCV库进行四个点裁剪的方法:
import cv2
import numpy as np
def crop_image_by_points(image_path, points):
"""
根据四个点裁剪图像
:param image_path: 图像路径
:param points: 四个点坐标,格式为[(x1, y1), (x2, y2), (x3, y3), (x4, y4)]
:return: 裁剪后的图像
"""
image = cv2.imread(image_path)
# 创建一个掩膜
mask = np.zeros(image.shape[:2], dtype=np.uint8)
points = np.array([points], dtype=np.int32)
cv2.fillPoly(mask, points, 255)
# 应用掩膜
masked_image = cv2.bitwise_and(image, image, mask=mask)
# 提取裁剪区域
x, y, w, h = cv2.boundingRect(points)
cropped_image = masked_image[y:y+h, x:x+w]
return cropped_image
使用示例
image_path = 'example.jpg'
points = [(50, 50), (150, 50), (150, 150), (50, 150)]
cropped_image = crop_image_by_points(image_path, points)
cv2.imshow('Cropped Image', cropped_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
使用多边形裁剪
对于不规则四边形的裁剪,可以使用多边形裁剪的方法。这需要使用OpenCV库的 cv2.getPerspectiveTransform
和 cv2.warpPerspective
方法。
import cv2
import numpy as np
def crop_image_by_polygon(image_path, points):
"""
根据四个点裁剪图像
:param image_path: 图像路径
:param points: 四个点坐标,格式为[(x1, y1), (x2, y2), (x3, y3), (x4, y4)]
:return: 裁剪后的图像
"""
image = cv2.imread(image_path)
height, width = image.shape[:2]
# 定义目标图像的宽度和高度
target_width = max(np.linalg.norm(np.array(points[0]) - np.array(points[1])),
np.linalg.norm(np.array(points[2]) - np.array(points[3])))
target_height = max(np.linalg.norm(np.array(points[0]) - np.array(points[3])),
np.linalg.norm(np.array(points[1]) - np.array(points[2])))
target_points = np.array([
[0, 0],
[target_width - 1, 0],
[target_width - 1, target_height - 1],
[0, target_height - 1]
], dtype="float32")
# 获取变换矩阵并进行透视变换
matrix = cv2.getPerspectiveTransform(np.array(points, dtype="float32"), target_points)
cropped_image = cv2.warpPerspective(image, matrix, (int(target_width), int(target_height)))
return cropped_image
使用示例
image_path = 'example.jpg'
points = [(50, 50), (150, 50), (150, 150), (50, 150)]
cropped_image = crop_image_by_polygon(image_path, points)
cv2.imshow('Cropped Image', cropped_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
总结
通过以上方法,我们可以在Python中使用Pillow库和OpenCV库,根据四个点坐标裁剪图像。Pillow库适用于简单的矩形裁剪,而OpenCV库则提供了更强大的功能,能够处理不规则的四边形裁剪。在实际应用中,可以根据需求选择合适的方法。
相关问答FAQs:
如何使用Python根据四个点切图?
在Python中,可以使用OpenCV和PIL等库来根据四个点进行图像切割。首先,需要确定四个点的坐标,并确保它们的顺序是正确的。接着,可以利用这些坐标创建一个多边形,并使用裁剪方法从原图中提取出这个区域。
在切图过程中,如何处理图像的坐标系?
在图像处理中,坐标系的原点通常位于左上角,X轴向右,Y轴向下。因此,在提供四个点的坐标时,需要确保它们符合这种坐标系统。这意味着左上角的点坐标应为 (x1, y1),而右下角的点坐标应为 (x2, y2)。确保坐标顺序的正确性对于准确裁剪至关重要。
切图后如何保存处理后的图像?
切图完成后,可以使用PIL库的save
方法或OpenCV的imwrite
函数来保存处理后的图像。确保指定正确的文件路径和格式,如JPEG或PNG等,以便后续使用。存储时可以考虑文件的压缩率和质量,以平衡图像质量和文件大小。
切图时如何处理异常情况?
在进行图像切割时,可能会遇到一些异常情况,例如给定的点超出图像边界或点的顺序不正确。可以通过添加条件判断来检查这些情况,并在遇到问题时返回友好的错误信息,确保程序能够稳健地运行,避免崩溃。
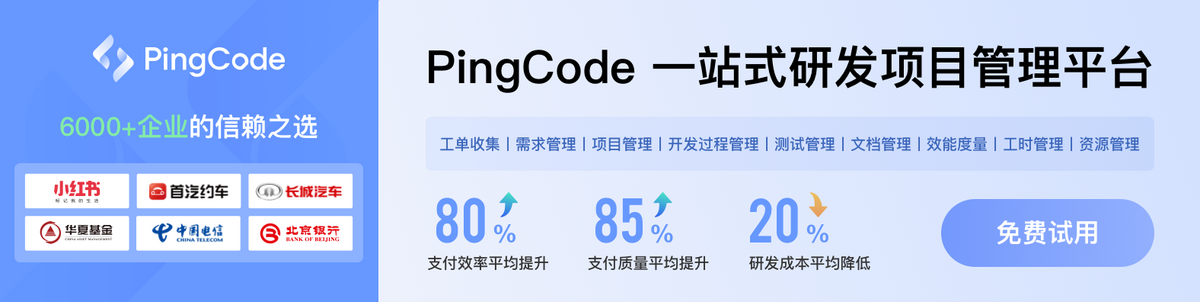