在Python中读取文件中指定内容的方法有多种,包括读取特定行、特定字符范围、特定模式匹配的内容等。 常用的方法有使用内置的open
函数和文件对象的方法来读取文件内容。接下来,我将详细介绍几种常见的方法,并提供示例代码。
一、读取特定行内容
在一些情况下,我们可能只需要读取文件中的某几行内容。可以使用循环和readlines()
方法来实现这一点。
使用readlines()
方法读取特定行
readlines()
方法将整个文件内容读取到一个列表中,每一行作为列表中的一个元素,然后通过索引可以获取特定的行。
def read_specific_lines(file_path, line_numbers):
with open(file_path, 'r') as file:
lines = file.readlines()
result = [lines[i-1] for i in line_numbers if i <= len(lines)]
return result
file_path = 'example.txt'
line_numbers = [1, 3, 5]
specific_lines = read_specific_lines(file_path, line_numbers)
for line in specific_lines:
print(line, end='')
使用循环读取特定行
通过循环和条件判断可以实现更高效的特定行读取,尤其是当文件非常大的时候。
def read_specific_lines_efficient(file_path, line_numbers):
result = []
with open(file_path, 'r') as file:
for i, line in enumerate(file, start=1):
if i in line_numbers:
result.append(line)
return result
file_path = 'example.txt'
line_numbers = [2, 4, 6]
specific_lines = read_specific_lines_efficient(file_path, line_numbers)
for line in specific_lines:
print(line, end='')
二、读取特定字符范围
有时我们可能需要从文件中读取特定字符范围的内容。这可以通过seek()
和read()
方法来实现。
def read_specific_characters(file_path, start, length):
with open(file_path, 'r') as file:
file.seek(start)
content = file.read(length)
return content
file_path = 'example.txt'
start = 10
length = 20
specific_content = read_specific_characters(file_path, start, length)
print(specific_content)
三、读取匹配特定模式的内容
在实际应用中,经常需要从文件中读取符合特定模式(如正则表达式)的内容。可以使用Python内置的re
模块来实现。
使用正则表达式读取匹配内容
import re
def read_matching_lines(file_path, pattern):
matching_lines = []
with open(file_path, 'r') as file:
for line in file:
if re.search(pattern, line):
matching_lines.append(line)
return matching_lines
file_path = 'example.txt'
pattern = r'\berror\b'
matching_lines = read_matching_lines(file_path, pattern)
for line in matching_lines:
print(line, end='')
四、逐行读取并处理文件内容
逐行读取文件内容是处理大文件的常用方法,因为它不会一次性将整个文件加载到内存中,避免了内存占用过大的问题。
使用生成器逐行读取
def read_file_line_by_line(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
file_path = 'example.txt'
for line in read_file_line_by_line(file_path):
print(line, end='')
五、读取文件中的特定列
在处理CSV文件或以特定分隔符分隔的文件时,读取特定列的数据是常见需求。可以使用csv
模块来实现。
使用csv模块读取特定列
import csv
def read_specific_columns(file_path, column_indices):
result = []
with open(file_path, 'r') as file:
reader = csv.reader(file)
for row in reader:
selected_columns = [row[i] for i in column_indices]
result.append(selected_columns)
return result
file_path = 'example.csv'
column_indices = [0, 2]
specific_columns = read_specific_columns(file_path, column_indices)
for row in specific_columns:
print(row)
六、读取文件中的特定部分内容
在一些情况下,我们可能需要读取文件中的特定部分内容,比如从某个标记开始到另一个标记结束的内容。这可以通过逐行读取和条件判断来实现。
读取标记之间的内容
def read_between_markers(file_path, start_marker, end_marker):
result = []
start_reading = False
with open(file_path, 'r') as file:
for line in file:
if start_marker in line:
start_reading = True
continue
if end_marker in line:
start_reading = False
if start_reading:
result.append(line)
return result
file_path = 'example.txt'
start_marker = 'START'
end_marker = 'END'
content_between_markers = read_between_markers(file_path, start_marker, end_marker)
for line in content_between_markers:
print(line, end='')
七、读取文件内容并处理
在实际应用中,读取文件内容后往往需要进行进一步的处理,如数据分析、统计等。下面是一个读取文件内容并统计单词出现次数的示例。
统计文件中单词出现次数
from collections import Counter
import re
def count_words_in_file(file_path):
word_count = Counter()
with open(file_path, 'r') as file:
for line in file:
words = re.findall(r'\b\w+\b', line.lower())
word_count.update(words)
return word_count
file_path = 'example.txt'
word_count = count_words_in_file(file_path)
for word, count in word_count.items():
print(f'{word}: {count}')
总结:
在Python中,读取文件中指定内容的方法多种多样,包括读取特定行、特定字符范围、特定模式匹配的内容等。可以根据具体需求选择合适的方法,并结合文件处理操作实现更复杂的功能。通过合理使用文件操作方法,可以高效地处理文件内容,从而提高开发效率和代码质量。
相关问答FAQs:
如何在Python中打开文件并读取其内容?
在Python中,可以使用内置的open()
函数打开文件,结合read()
、readline()
或readlines()
方法来读取文件内容。使用with
语句可以确保文件在读取后自动关闭,避免资源泄露。例如:
with open('文件路径', 'r') as file:
content = file.read()
print(content)
这种方法简单且安全,适合读取整个文件。
如何根据特定条件从文件中提取内容?
若想从文件中提取符合特定条件的内容,可以逐行读取文件并使用条件判断。例如,若要读取包含特定关键词的行,可以这样做:
with open('文件路径', 'r') as file:
for line in file:
if '关键词' in line:
print(line)
这种方法灵活,可以根据需要调整条件。
在Python中如何读取大型文件而不占用过多内存?
处理大型文件时,可以逐块读取文件内容,以避免占用过多内存。使用read(size)
方法可以设定每次读取的字节数,例如:
with open('文件路径', 'r') as file:
while True:
chunk = file.read(1024) # 每次读取1024字节
if not chunk:
break
print(chunk)
这种方式适合处理大文件,确保程序流畅运行。
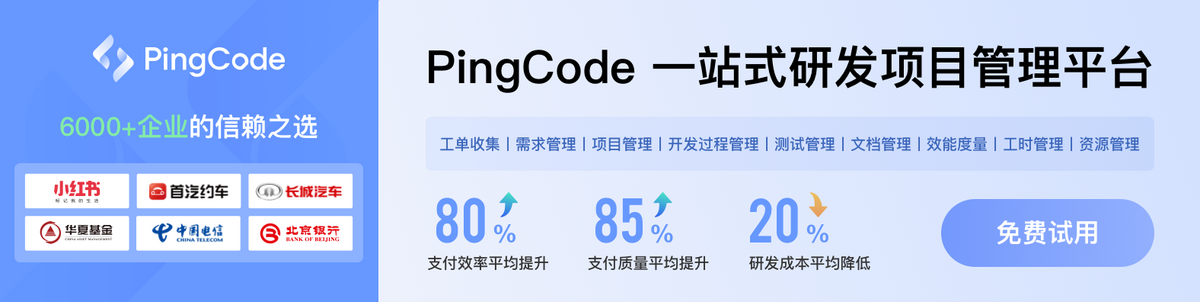