Python 判断是否含有某关键词的方法有:使用 in 操作符、使用字符串方法 str.contains()、正则表达式等。其中,使用 in 操作符是最常见且最简单的方法。下面详细讲解如何使用这些方法来判断字符串中是否包含某关键词。
一、使用 in 操作符
使用 in 操作符是判断字符串中是否包含某个子字符串的最简单方法。示例代码如下:
keyword = "Python"
text = "I am learning Python programming."
if keyword in text:
print("The keyword is found in the text.")
else:
print("The keyword is not found in the text.")
通过这种方式,可以轻松地判断字符串中是否包含某个关键词。
二、使用字符串方法 str.contains()
Python 的字符串类提供了一些方法来处理字符串,其中 str.contains()
是一个常用的方法。这种方法需要借助 pandas 库来实现。示例代码如下:
import pandas as pd
keyword = "Python"
text = "I am learning Python programming."
if pd.Series([text]).str.contains(keyword).any():
print("The keyword is found in the text.")
else:
print("The keyword is not found in the text.")
这种方法的优点是可以处理更复杂的字符串匹配,例如区分大小写、使用正则表达式等。
三、使用正则表达式
正则表达式是一种强大的工具,可以用来匹配字符串中的复杂模式。Python 提供了 re 模块来处理正则表达式。示例代码如下:
import re
keyword = "Python"
text = "I am learning Python programming."
if re.search(keyword, text):
print("The keyword is found in the text.")
else:
print("The keyword is not found in the text.")
这种方法的优点是可以处理更复杂的字符串匹配,例如精确匹配、忽略大小写等。
四、忽略大小写的判断
有时候我们需要忽略大小写来判断字符串中是否包含某个关键词。可以使用字符串的 lower()
或 upper()
方法将字符串和关键词统一转换成小写或大写,然后再进行比较。示例代码如下:
keyword = "python"
text = "I am learning Python programming."
if keyword.lower() in text.lower():
print("The keyword is found in the text.")
else:
print("The keyword is not found in the text.")
这种方法的优点是简单易懂且适用范围广。
五、判断多个关键词
有时候我们需要判断字符串中是否包含多个关键词,可以使用循环或者任意一个关键词匹配成功的方式来实现。示例代码如下:
keywords = ["Python", "programming"]
text = "I am learning Python programming."
if any(keyword in text for keyword in keywords):
print("At least one keyword is found in the text.")
else:
print("No keywords are found in the text.")
这种方法可以处理多个关键词的匹配需求。
六、判断是否包含完整单词
有时候我们需要判断字符串中是否包含某个完整的单词,而不仅仅是子字符串。可以使用正则表达式来实现。示例代码如下:
import re
keyword = r'\bPython\b'
text = "I am learning Python programming."
if re.search(keyword, text):
print("The keyword is found in the text.")
else:
print("The keyword is not found in the text.")
这种方法的优点是可以精确匹配完整单词。
七、处理包含特殊字符的关键词
如果关键词中包含特殊字符,可以使用正则表达式中的转义字符来处理。示例代码如下:
import re
keyword = r'\bC\+\+\b'
text = "I am learning C++ programming."
if re.search(keyword, text):
print("The keyword is found in the text.")
else:
print("The keyword is not found in the text.")
这种方法的优点是可以处理包含特殊字符的关键词。
八、判断是否包含多个完整单词
如果需要判断字符串中是否包含多个完整单词,可以使用正则表达式中的组匹配来实现。示例代码如下:
import re
keywords = [r'\bPython\b', r'\bprogramming\b']
text = "I am learning Python programming."
if all(re.search(keyword, text) for keyword in keywords):
print("All keywords are found in the text.")
else:
print("Not all keywords are found in the text.")
这种方法的优点是可以处理多个完整单词的匹配需求。
九、提高匹配效率
在处理大量文本时,提高匹配效率是非常重要的。可以使用编译后的正则表达式来提高匹配效率。示例代码如下:
import re
keyword = re.compile(r'\bPython\b')
text = "I am learning Python programming."
if keyword.search(text):
print("The keyword is found in the text.")
else:
print("The keyword is not found in the text.")
这种方法的优点是可以提高匹配效率,特别是在处理大量文本时。
十、总结
通过以上几种方法,可以灵活地判断 Python 字符串中是否包含某个关键词。每种方法都有其优点和适用场景,可以根据实际需求选择合适的方法。无论是简单的 in 操作符,还是强大的正则表达式,都可以帮助我们快速、准确地判断字符串中是否包含某个关键词。
相关问答FAQs:
如何在Python中检查一个字符串是否包含特定关键词?
您可以使用Python的in
运算符来判断一个字符串是否包含特定的关键词。例如,您可以这样写:if '关键词' in '您的字符串'
。如果关键词存在,条件将返回True。
在Python中,是否可以忽略大小写来判断关键词?
确实可以。您可以将字符串和关键词都转换为小写或大写,再进行比较。例如,使用str.lower()
方法:if '关键词'.lower() in '您的字符串'.lower()
。这种方式确保了大小写不影响判断结果。
Python中如何处理多个关键词的判断?
如果需要检查多个关键词,可以使用循环或列表推导式。例如,您可以定义一个关键词列表,遍历该列表并检查每个关键词是否在目标字符串中。示例代码如下:
keywords = ['关键词1', '关键词2', '关键词3']
for keyword in keywords:
if keyword in target_string:
print(f"找到关键词: {keyword}")
这种方法非常灵活,适用于多种场景。
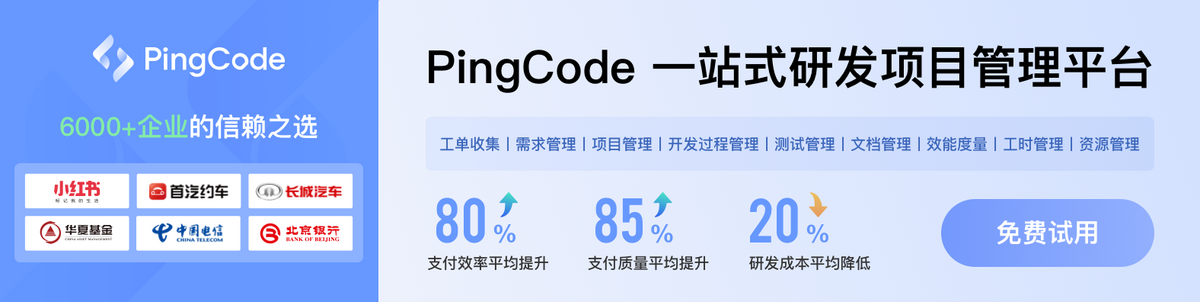