开头段落:
Python表白小程序可以通过微信公众平台、微信小程序开发平台、微信 API 接口来实现将表白信息发送到微信。通过这些平台和接口,开发者可以将 Python 代码与微信生态系统进行无缝集成,从而实现将表白信息发送到微信好友、群组或者朋友圈。接下来,我们将详细探讨这几种方法,并介绍每种方法的优缺点和具体实现步骤。
一、微信公众平台
微信公众平台是开发者可以利用的一个强大的工具,可以实现自动发送消息、推送文章等功能。通过公众号,开发者可以使用 Python 发送表白信息到关注公众号的用户。
1、创建公众号
首先,需要在微信公众平台上注册并创建一个公众号。可以选择订阅号或服务号,这取决于具体的需求和功能。
2、获取开发者权限
在公众号后台,进入“开发”模块,申请获取开发者权限。需要填写服务器配置,配置好URL和Token等信息。
3、使用微信公众平台接口
通过微信公众号的API接口,我们可以在服务器端编写Python代码,使用Flask或Django等框架搭建一个服务器,处理微信服务器发送过来的消息,并根据用户的输入发送表白信息。
from flask import Flask, request, make_response
import hashlib
app = Flask(__name__)
@app.route('/wechat', methods=['GET', 'POST'])
def wechat():
if request.method == 'GET':
# 验证消息的确来自微信服务器
token = 'your_token'
query = request.args
signature = query.get('signature', '')
timestamp = query.get('timestamp', '')
nonce = query.get('nonce', '')
echostr = query.get('echostr', '')
s = [timestamp, nonce, token]
s.sort()
s = ''.join(s)
if (hashlib.sha1(s.encode('utf-8')).hexdigest() == signature):
return make_response(echostr)
else:
# 处理微信服务器发来的消息
xml_data = request.data
# 解析xml_data,提取用户输入并发送表白信息
# ...
return make_response('')
if __name__ == '__main__':
app.run()
4、发送表白信息
在处理用户输入的部分,可以根据预定义的关键词发送表白信息。可以使用模板消息接口推送个性化的表白内容。
import requests
import json
def send_template_message(to_user, template_id, data):
access_token = 'your_access_token'
url = f'https://api.weixin.qq.com/cgi-bin/message/template/send?access_token={access_token}'
payload = {
'touser': to_user,
'template_id': template_id,
'data': data
}
headers = {'content-type': 'application/json'}
response = requests.post(url, data=json.dumps(payload), headers=headers)
return response.json()
二、微信小程序开发平台
微信小程序也是一个非常有效的工具,能够实现更多的交互功能和更好的用户体验。通过微信小程序,用户可以直接在微信中体验到表白小程序的功能,并且可以与好友分享。
1、创建小程序
首先,需要在微信小程序开发平台上注册并创建一个小程序。
2、开发小程序前端
使用微信小程序开发工具开发小程序的前端部分,可以使用WXML、WXSS和JavaScript来编写页面和逻辑。
<!-- pages/index/index.wxml -->
<view class="container">
<view class="form">
<input type="text" placeholder="请输入表白内容" bindinput="inputChange" />
<button bindtap="sendLove">发送表白</button>
</view>
</view>
// pages/index/index.js
Page({
data: {
loveMessage: ''
},
inputChange: function(e) {
this.setData({
loveMessage: e.detail.value
});
},
sendLove: function() {
// 调用云函数发送表白信息
wx.cloud.callFunction({
name: 'sendLove',
data: {
message: this.data.loveMessage
},
success: function(res) {
console.log('表白信息发送成功', res);
},
fail: function(err) {
console.error('表白信息发送失败', err);
}
});
}
});
3、开发小程序后台
使用微信云开发来构建小程序的后台逻辑,可以编写云函数来处理表白信息的发送。
// cloudfunctions/sendLove/index.js
const cloud = require('wx-server-sdk');
cloud.init();
exports.main = async (event, context) => {
const { OPENID } = cloud.getWXContext();
const { message } = event;
// 这里可以调用微信 API 接口发送表白信息
// 例如,使用订阅消息接口推送表白内容
try {
const result = await cloud.openapi.subscribeMessage.send({
touser: OPENID,
templateId: 'your_template_id',
data: {
thing1: {
value: message
},
time2: {
value: new Date().toLocaleString()
}
}
});
return result;
} catch (err) {
return err;
}
};
三、微信 API 接口
微信 API 接口提供了丰富的功能,开发者可以通过这些接口实现多种功能,包括发送表白信息。
1、获取Access Token
使用微信 API 接口,需要先获取 Access Token。可以通过公众号后台或者小程序后台获取。
import requests
def get_access_token(appid, secret):
url = f'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={appid}&secret={secret}'
response = requests.get(url)
data = response.json()
return data.get('access_token')
2、发送客服消息
通过微信 API 接口,可以使用客服消息接口发送表白信息。
def send_custom_message(access_token, to_user, message):
url = f'https://api.weixin.qq.com/cgi-bin/message/custom/send?access_token={access_token}'
payload = {
'touser': to_user,
'msgtype': 'text',
'text': {
'content': message
}
}
headers = {'content-type': 'application/json'}
response = requests.post(url, data=json.dumps(payload), headers=headers)
return response.json()
3、发送模板消息
模板消息接口也是一个非常有效的工具,可以用来发送结构化的表白信息。
def send_template_message(access_token, to_user, template_id, data):
url = f'https://api.weixin.qq.com/cgi-bin/message/template/send?access_token={access_token}'
payload = {
'touser': to_user,
'template_id': template_id,
'data': data
}
headers = {'content-type': 'application/json'}
response = requests.post(url, data=json.dumps(payload), headers=headers)
return response.json()
总结:
通过微信公众平台、微信小程序开发平台和微信 API 接口,开发者可以实现将Python表白小程序的表白信息发送到微信。每种方法都有其独特的优势和适用场景,开发者可以根据具体需求选择合适的实现方式。无论选择哪种方式,都需要遵循微信平台的开发规范和安全要求,确保用户体验和数据安全。
相关问答FAQs:
如何使用Python编写一个可以通过微信发送表白信息的小程序?
要创建一个可以通过微信发送表白信息的小程序,您需要使用Python的微信API,例如itchat
库。安装此库后,您可以通过编写代码来登录微信,并发送文本消息。确保您在程序中使用正确的好友用户名或ID,以便消息能够发送到目标联系人。
这个小程序需要哪些依赖库或工具?
制作这个小程序需要安装Python和一些相关库,如itchat
,requests
等。您可以通过命令行使用pip install itchat
进行安装。同时,确保您的Python环境已经配置好,并且可以访问网络,以便与微信服务器进行交互。
发送表白信息时,有没有什么注意事项?
在发送表白信息时,建议您提前测试程序,确保信息可以顺利发送。注意控制发送频率,以免触发微信的反垃圾机制。此外,发送的内容要真诚,避免使用过于机械的文本。同时,确保您的好友在微信上是可见的,以便信息能够成功送达。
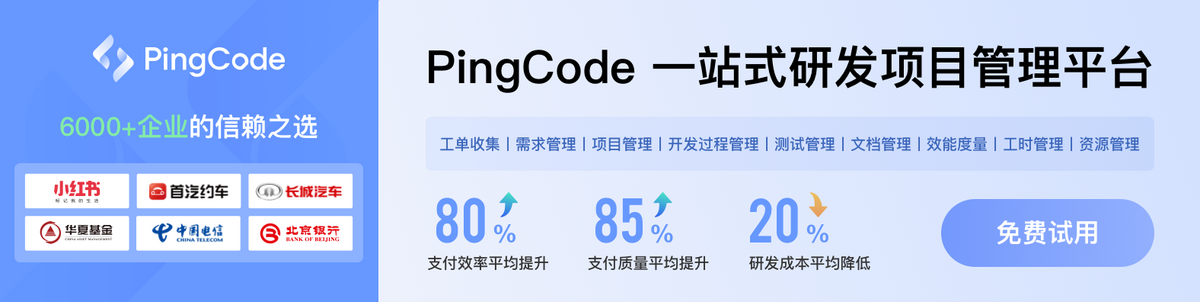