一、如何使用Python在微信上发送图片
使用Python在微信上发送图片可以通过多个方法实现:使用wxpy库、使用itchat库、使用微信企业版API等。在这篇文章中,我们将详细介绍如何使用wxpy库和itchat库来实现这一功能,并对wxpy库的具体实现进行详细描述。
- wxpy库:wxpy是一个基于itchat的微信机器人库,提供了简单易用的API,可以方便地实现微信消息的发送和接收。
- itchat库:itchat是一个开源的微信个人号接口,可以方便地实现微信消息的发送和接收。
- 微信企业版API:微信企业版API提供了丰富的接口,可以实现微信消息的发送和接收,但是需要企业认证。
接下来,我们将详细介绍wxpy库的使用方法。
二、wxpy库的使用方法
1. 安装wxpy库
首先,我们需要安装wxpy库,可以通过pip命令来安装:
pip install wxpy
2. 登录微信
使用wxpy库登录微信,代码如下:
from wxpy import Bot
初始化机器人,扫码登录
bot = Bot()
3. 发送图片
使用wxpy库发送图片,代码如下:
# 查找好友
friend = bot.friends().search('好友昵称')[0]
发送图片
friend.send_image('图片路径')
4. 完整示例
下面是一个完整的示例代码:
from wxpy import Bot
初始化机器人,扫码登录
bot = Bot()
查找好友
friend = bot.friends().search('好友昵称')[0]
发送图片
friend.send_image('图片路径')
退出登录
bot.logout()
三、itchat库的使用方法
1. 安装itchat库
首先,我们需要安装itchat库,可以通过pip命令来安装:
pip install itchat
2. 登录微信
使用itchat库登录微信,代码如下:
import itchat
登录微信
itchat.auto_login(hotReload=True)
3. 发送图片
使用itchat库发送图片,代码如下:
# 查找好友
friend = itchat.search_friends(name='好友昵称')[0]
发送图片
itchat.send_image('图片路径', toUserName=friend['UserName'])
4. 完整示例
下面是一个完整的示例代码:
import itchat
登录微信
itchat.auto_login(hotReload=True)
查找好友
friend = itchat.search_friends(name='好友昵称')[0]
发送图片
itchat.send_image('图片路径', toUserName=friend['UserName'])
退出登录
itchat.logout()
四、如何处理发送大图片的问题
在使用wxpy或itchat库发送图片时,如果图片文件较大,可能会遇到一些问题。以下是一些解决方法:
- 压缩图片:在发送图片之前,可以先对图片进行压缩,减小图片文件的大小。
- 分片发送:对于超大图片,可以将图片进行分片,然后逐片发送。
- 使用第三方存储:将大图片上传到第三方存储服务(如阿里云、腾讯云等),然后发送图片的链接。
1. 压缩图片
可以使用PIL库对图片进行压缩,代码如下:
from PIL import Image
def compress_image(input_path, output_path, quality=85):
# 打开图片
img = Image.open(input_path)
# 压缩图片
img.save(output_path, quality=quality)
压缩图片
compress_image('原图片路径', '压缩后图片路径')
2. 分片发送
可以将图片进行分片,然后逐片发送,代码如下:
import itchat
import os
def send_large_image(image_path, friend):
# 获取图片大小
image_size = os.path.getsize(image_path)
# 定义分片大小(1MB)
chunk_size = 1024 * 1024
# 计算分片数量
num_chunks = (image_size + chunk_size - 1) // chunk_size
# 逐片发送
for i in range(num_chunks):
with open(image_path, 'rb') as f:
f.seek(i * chunk_size)
chunk_data = f.read(chunk_size)
itchat.send(chunk_data, toUserName=friend['UserName'])
登录微信
itchat.auto_login(hotReload=True)
查找好友
friend = itchat.search_friends(name='好友昵称')[0]
发送大图片
send_large_image('大图片路径', friend)
退出登录
itchat.logout()
3. 使用第三方存储
可以将大图片上传到第三方存储服务,然后发送图片的链接,代码如下:
import itchat
上传图片到第三方存储服务,获取图片链接
image_url = upload_image_to_storage('大图片路径')
登录微信
itchat.auto_login(hotReload=True)
查找好友
friend = itchat.search_friends(name='好友昵称')[0]
发送图片链接
itchat.send(image_url, toUserName=friend['UserName'])
退出登录
itchat.logout()
五、总结
通过本文的介绍,我们详细了解了如何使用Python在微信上发送图片,重点介绍了wxpy库和itchat库的使用方法,并探讨了如何处理发送大图片的问题。无论是使用wxpy库、itchat库还是微信企业版API,都是实现这一功能的有效方法。希望本文的内容对您有所帮助,如果有任何疑问或建议,欢迎在下方留言交流。
相关问答FAQs:
如何使用Python在微信上发送特定大小的图片?
在使用Python发送图片之前,您可以使用Pillow库来调整图片的大小。首先,您需要安装Pillow库,可以通过命令pip install Pillow
来完成。接下来,使用以下代码调整图片大小并通过微信发送:
from PIL import Image
import itchat
# 调整图片大小
def resize_image(input_path, output_path, size):
with Image.open(input_path) as img:
img = img.resize(size)
img.save(output_path)
# 发送图片
def send_image(to_user, image_path):
itchat.auto_login()
itchat.send_image(image_path, toUser=to_user)
# 使用示例
resize_image('original_image.jpg', 'resized_image.jpg', (800, 600))
send_image('filehelper', 'resized_image.jpg')
这样,您就可以按照需要的尺寸发送图片了。
在微信上发送图片时,有没有尺寸限制?
微信对于发送图片的尺寸并没有严格的限制,但最佳尺寸通常在800×600像素左右,以确保在各种设备上显示良好。如果图片过大,可能会导致发送失败或降低图片质量。因此,建议在发送之前先调整图片大小,以适应微信的要求。
使用Python发送图片时,是否需要特别的权限或配置?
在使用Python发送图片到微信时,您需要确保已登录微信账号。itchat
库提供了自动登录的功能,但首次使用时需要扫描二维码进行验证。此外,确保您的网络连接良好,以避免发送过程中出现问题。
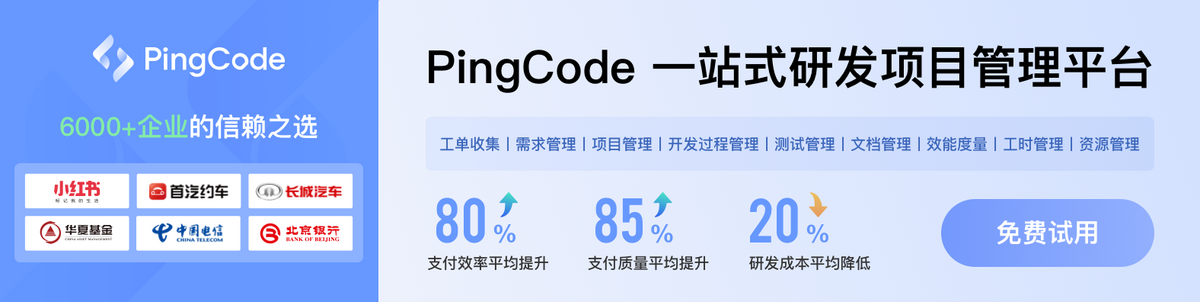